Hello guys, how are you? Welcome back to my blog. Today in this post we will do How To integrate JWT authentication with user login functionality in a React application?
You’ll need to handle the frontend user login interface and ensure that the JWT is properly generated and validated in the backend. Below, I’ll provide a comprehensive guide on setting up both the frontend (React) and the backend, assuming a Node.js/Express backend for handling JWT.
For react js new comers, please check the below links:
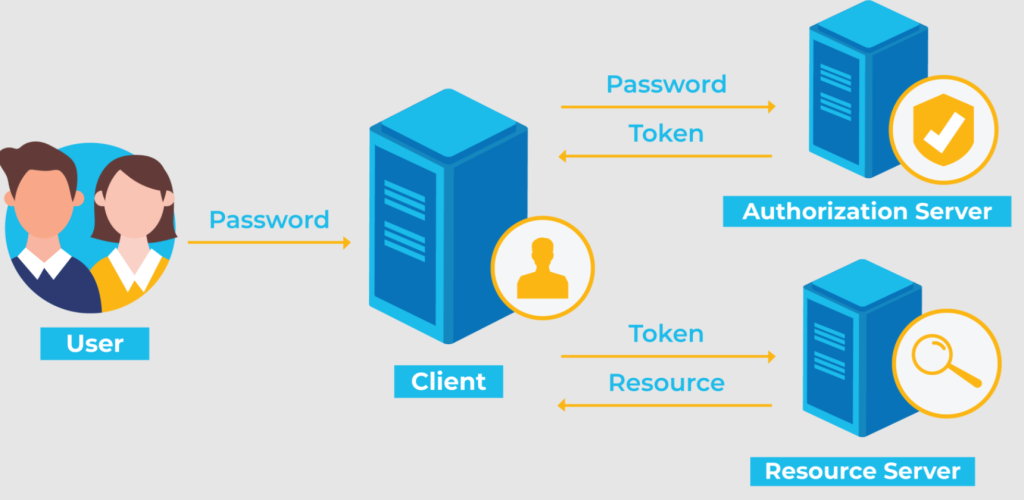
Backend Setup
You will first need to set up a backend that can generate and validate JWT tokens. Here’s an example using Node.js with Express:
- Set up the project and install dependencies:
npm init -y npm install express jsonwebtoken bcryptjs cors dotenv
- Create your server (
server.js
):
require('dotenv').config(); const express = require('express'); const jwt = require('jsonwebtoken'); const bcrypt = require('bcryptjs'); const cors = require('cors'); const app = express(); const PORT = process.env.PORT || 3000; // Middleware app.use(express.json()); app.use(cors()); // Mock user database const users = [ { id: 1, username: 'user1', password: '$2a$10$examplehash' } // Use bcrypt to hash actual passwords ]; // Login Route app.post('/login', async (req, res) => { const { username, password } = req.body; const user = users.find(u => u.username === username); if (user && await bcrypt.compare(password, user.password)) { const token = jwt.sign({ id: user.id, username: user.username }, process.env.JWT_SECRET, { expiresIn: '2h' }); res.json({ token }); } else { res.status(401).send('Username or password incorrect'); } }); app.listen(PORT, () => { console.log(`Server running on http://localhost:${PORT}`); });
In this example, replace 'your_secret_key'
with an actual secure secret key stored in an environment variable and use bcrypt
for hashing and comparing passwords.
Frontend Setup with React
Now, let’s set up the React frontend:
- Create the React app:
npx create-react-app jwt-react-auth cd jwt-react-auth npm start
- Install Axios for HTTP requests:
npm install axios
- Create the Login component (
Login.js
):
import React, { useState } from 'react'; import axios from 'axios'; const Login = ({ onLogin }) => { const [username, setUsername] = useState(''); const [password, setPassword] = useState(''); const handleLogin = async (e) => { e.preventDefault(); try { const { data } = await axios.post('http://localhost:3000/login', { username, password }); onLogin(data.token); } catch (error) { console.error('Login failed:', error.response.data); } }; return ( <form onSubmit={handleLogin}> <input type="text" value={username} onChange={e => setUsername(e.target.value)} placeholder="Username" required /> <input type="password" value={password} onChange={e => setPassword(e.target.value)} placeholder="Password" required /> <button type="submit">Login</button> </form> ); }; export default Login;
- Handle token storage and protected routes in your main App component (
App.js
):
import React, { useState } from 'react'; import Login from './Login'; import axios from 'axios'; function App() { const [token, setToken] = useState(localStorage.getItem('jwtToken')); const onLogin = (token) => { localStorage.setItem('jwtToken', token); setToken(token); axios.defaults.headers.common['Authorization'] = `Bearer ${token}`; }; return ( <div> {!token ? ( <Login onLogin={onLogin} /> ) : ( <h1>You are logged in!</h1> )} </div> ); } export default App;
This setup gives you a basic user authentication flow using JWTs in a React application. You have a login form that sends credentials to the backend, which validates them, generates a JWT, and sends it back to the frontend. The frontend then stores this token and uses it for subsequent authenticated requests. For production applications, consider more secure token storage mechanisms and additional security measures like HTTPS, token refresh strategies, and better error handling.
This is it guys and if you will have any kind of query, suggestion or requirement then feel free to comment below.
Thanks
Ajay