Hello friends, how are you? Welcome back to my blog therichpost.com. Today in this blog post, I am going to tell you, How to show MongoDB Collection Data in Angular 18 Frontend?
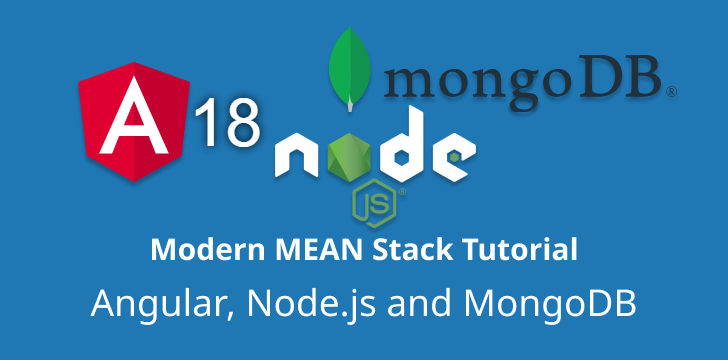
Angular 18 came . If you are new then you must check below two links:
Now guys here is the complete code snippet link and please follow carefully:
Setting up MongoDB with dummy records involves several steps. Here is a step-by-step guide to help you get started:
Step 1: Install MongoDB
- Download MongoDB:
- Visit the MongoDB download center and download the version suitable for your operating system.
- Follow the installation instructions specific to your OS.
- Install MongoDB:
- On Windows, run the installer and follow the instructions.
- On macOS, you can install MongoDB via Homebrew:
sh brew tap mongodb/brew brew install mongodb-community@<version>
- On Linux, follow the instructions on the MongoDB installation page for your distribution.
- Start MongoDB:
- On Windows, use the MongoDB Compass GUI or run the following command in your terminal:
sh mongod
- On macOS and Linux, start the MongoDB service:
sh brew services start mongodb/brew/mongodb-community
orsh sudo systemctl start mongod
Step 2: Set Up MongoDB
- Open MongoDB Shell:
- Open a terminal and run:
sh mongo
- Create a Database:
- In the MongoDB shell, create a new database:
js use mydatabase
- Create a Collection and Insert Dummy Records:
- Create a collection and insert some dummy records:
js db.mycollection.insertMany([ { name: "John Doe", age: 30, city: "New York" }, { name: "Jane Smith", age: 25, city: "Los Angeles" }, { name: "Mike Johnson", age: 35, city: "Chicago" } ])
Step 3: Verify the Records
- Query the Collection:
- Verify that the records have been inserted:
js db.mycollection.find().pretty()
You should see output similar to this:
[ { "_id": ObjectId("..."), "name": "John Doe", "age": 30, "city": "New York" }, { "_id": ObjectId("..."), "name": "Jane Smith", "age": 25, "city": "Los Angeles" }, { "_id": ObjectId("..."), "name": "Mike Johnson", "age": 35, "city": "Chicago" } ]
Optional: Use a GUI Tool
For easier management and visualization of your database, you can use a GUI tool like MongoDB Compass.
Example with Python
If you want to use Python to insert and manage dummy records, you can use the pymongo
library:
- Install
pymongo
:
pip install pymongo
- Python Script to Insert Dummy Records:
from pymongo import MongoClient client = MongoClient('localhost', 27017) db = client.mydatabase collection = db.mycollection dummy_records = [ {"name": "John Doe", "age": 30, "city": "New York"}, {"name": "Jane Smith", "age": 25, "city": "Los Angeles"}, {"name": "Mike Johnson", "age": 35, "city": "Chicago"} ] collection.insert_many(dummy_records) for record in collection.find(): print(record)
This script connects to your MongoDB instance, creates a database and collection, inserts dummy records, and prints them to the console.
By following these steps, you’ll have MongoDB set up with dummy records for testing and development purposes.
To display MongoDB data in an Angular 18 standalone components frontend, you need to follow these steps:
- Set up a backend server to fetch data from MongoDB and expose it via a REST API.
- Set up the Angular frontend to consume the REST API and display the data.
Step 1: Set Up Backend Server
We’ll use Node.js with Express and Mongoose for the backend.
- Create a new Node.js project:
mkdir myapp-backend cd myapp-backend npm init -y
- Install dependencies:
npm install express mongoose cors
- Create the backend server (
server.js
):
const express = require('express'); const mongoose = require('mongoose'); const cors = require('cors'); const app = express(); const port = 3000; // Middleware app.use(cors()); app.use(express.json()); // Connect to MongoDB mongoose.connect('mongodb://localhost:27017/mydatabase', { useNewUrlParser: true, useUnifiedTopology: true }); // Define a schema and model const userSchema = new mongoose.Schema({ name: String, age: Number, city: String }); const User = mongoose.model('User', userSchema); // Define a route to fetch data app.get('/users', async (req, res) => { try { const users = await User.find(); res.json(users); } catch (error) { res.status(500).send(error); } }); // Start the server app.listen(port, () => { console.log(`Server is running on http://localhost:${port}`); });
- Run the backend server:
node server.js
Step 2: Set Up Angular Frontend
- Create a new Angular project:
ng new myapp-frontend cd myapp-frontend
- Install Angular HTTP client:
ng add @angular/common@latest
- Create a standalone component:
ng generate component UserList --standalone
- Create a service to fetch data (
src/app/user.service.ts
):
import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Observable } from 'rxjs'; export interface User { name: string; age: number; city: string; } @Injectable({ providedIn: 'root', }) export class UserService { private apiUrl = 'http://localhost:3000/users'; constructor(private http: HttpClient) {} getUsers(): Observable<User[]> { return this.http.get<User[]>(this.apiUrl); } }
- Update the standalone component to display data (
src/app/user-list/user-list.component.ts
):
import { Component, OnInit } from '@angular/core'; import { CommonModule } from '@angular/common'; import { HttpClientModule } from '@angular/common/http'; import { UserService, User } from '../user.service'; @Component({ selector: 'app-user-list', standalone: true, imports: [CommonModule, HttpClientModule], template: ` <h1>User List</h1> <ul> <li *ngFor="let user of users"> {{ user.name }} ({{ user.age }}), {{ user.city }} </li> </ul> `, styles: [] }) export class UserListComponent implements OnInit { users: User[] = []; constructor(private userService: UserService) {} ngOnInit(): void { this.userService.getUsers().subscribe((data) => { this.users = data; }); } }
- Update
src/main.ts
to bootstrap the standalone component:
import { bootstrapApplication } from '@angular/platform-browser'; import { UserListComponent } from './app/user-list/user-list.component'; bootstrapApplication(UserListComponent) .catch(err => console.error(err));
Running the Application
- Start the Angular frontend:
ng serve
- Visit
http://localhost:4200
in your browser to see the user list displayed.
By following these steps, you will have set up a full-stack application with MongoDB as the database, an Express backend serving data via a REST API, and an Angular 18 frontend displaying the data using standalone components.
Note: Friends, In this post, I just tell the basic setup and things, you can change the code according to your requirements.
I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad because with your views, I will make my next posts more good and helpful.
Jassa
Thanks