Hello guys how are you? Welcome back to my blog therichpost.com. Guys today in this blog post I will let you know Protecting Routes With Auth Guard In Angular 14.
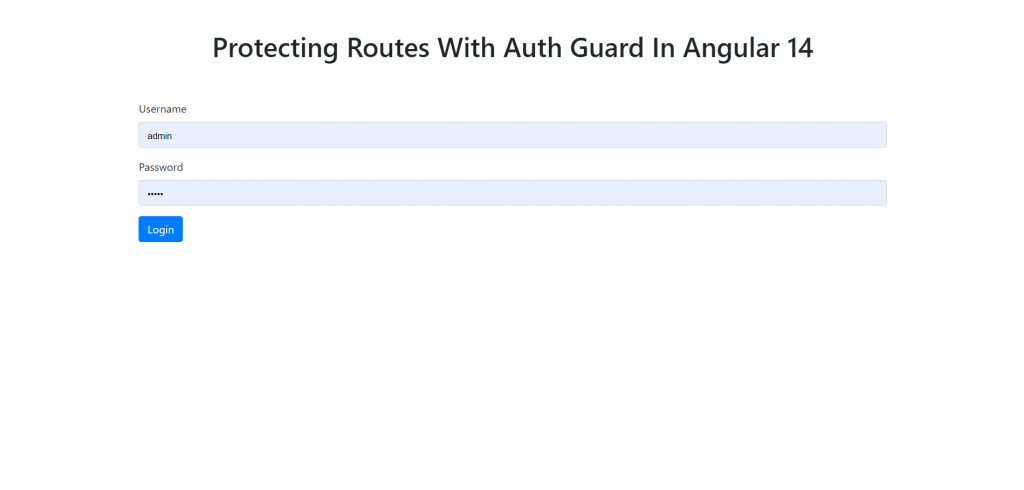
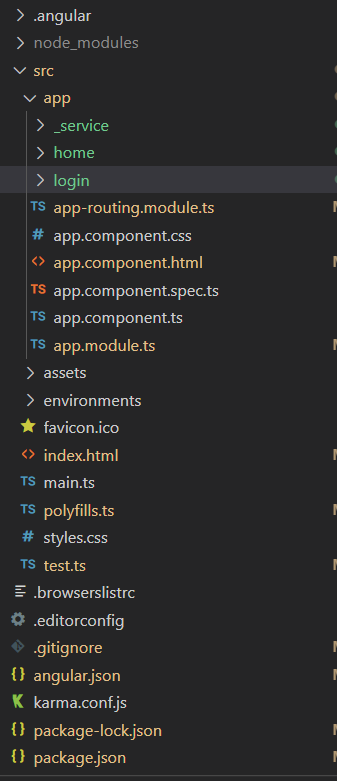
Guys Angular 14 came and if you are new in Angular then please must visit below link:
Guys here is working complete code snippet and please follow carefully:
1. Firstly friends we need fresh angular 14 setup and for this we need to run below commands but if you already have angular 14 setup then you can avoid below commands. Secondly we should also have latest node version installed on our system:
npm install -g @angular/cli ng new angulardemo //Create new Angular Project cd angulardemo// Go inside the Angular Project Folder
2. Now friends, here we need to run below commands into our project terminal to generate new components and services:
ng g service ./_service/auth-guard ng g service ./_service/authentication ng g c home ng g c login ng serve -- o
3. Now guys we need to add below code inside app/_service/auth-guard.service.ts file:
import { Injectable } from '@angular/core'; import { Router, CanActivate, ActivatedRouteSnapshot, RouterStateSnapshot } from '@angular/router'; @Injectable({ providedIn: 'root' }) export class AuthGuard implements CanActivate { constructor(private _router: Router) { } canActivate(next: ActivatedRouteSnapshot, state: RouterStateSnapshot) { if (localStorage.getItem('currentUser')) { return true; } this._router.navigate(['']); return false; } }
4. Now guys we need to add below code inside app/_service/authentication.service.ts file:
import { Injectable } from '@angular/core'; @Injectable({ providedIn: 'root' }) export class AuthenticationService { login(username: string, password: string):boolean { if (username == "admin" && password == "admin") { localStorage.setItem('currentUser', "loggedin"); return true; } return false; } logout() { localStorage.removeItem('currentUser'); } public get loggedIn(): boolean { return (localStorage.getItem('currentUser') !== null); } }
5. Now guys we need to add below code inside app/home/home.component.ts file:
import { Component, OnInit } from '@angular/core'; import { Router } from '@angular/router'; import { AuthenticationService } from '../_service/authentication.service'; @Component({ selector: 'app-home', templateUrl: './home.component.html', styleUrls: ['./home.component.css'] }) export class HomeComponent implements OnInit { constructor(private router: Router, private authenticationService: AuthenticationService) { } ngOnInit() { } logout() { this.authenticationService.logout(); this.router.navigate(['']); } }
6. Now guys we need to add below code inside app/home/home.component.html file:
<div class="container"> <h1>You are successfully logged in</h1> <button (click)="logout()" class="btn btn-danger">Logout</button> </div>
7. Now guys we need to add below code inside app/login/login.component.ts file:
import { Component, OnInit } from '@angular/core'; import { AuthenticationService } from '../_service/authentication.service'; import { Router } from '@angular/router'; @Component({ selector: 'app-login', templateUrl: './login.component.html', styleUrls: ['./login.component.css'] }) export class LoginComponent { username: string = ""; password: string = ""; title = 'auth-guard-demo'; constructor(private _auth: AuthenticationService, private _router: Router) { if (this._auth.loggedIn) { this._router.navigate(['home']); } } login(): void { if (this.username != '' && this.password != '') { if (this._auth.login(this.username, this.password)) { this._router.navigate(["home"]); } else alert("Wrong username or password"); } } }
8. Now guys we need to add below code inside app/login/login.component.html file:
<div class="container"> <div class="row"> <div class="form-group col-md-12"> <label>Username</label> <input type="text" [(ngModel)]="username" name="username" class="form-control" /> </div> <div class="form-group col-md-12"> <label>Password</label> <input type="password" [(ngModel)]="password" name="password" class="form-control" /> </div> <div class="form-group col-md-3"> <button (click)="login()" class="btn btn-primary">Login</button> </div> </div> </div>
9. Now guys we need to add below code inside app/app-routing.module.ts file:
import { NgModule } from '@angular/core'; import { Routes, RouterModule } from '@angular/router'; import { HomeComponent } from './home/home.component'; import { AuthGuard } from './_service/auth-guard.service'; import { LoginComponent } from './login/login.component'; const routes: Routes = [ { path: 'home', component: HomeComponent, canActivate: [AuthGuard], data: { title: 'Home' } }, { path: 'login', component: LoginComponent, data: { title: 'Login' } }, { path: '', component: LoginComponent, data: { title: 'Login' } } ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { }
10. Now guys we need to add below code inside app/app.module.ts file:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { FormsModule } from '@angular/forms'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { AuthenticationService } from './_service/authentication.service'; import { HomeComponent } from './home/home.component'; import { LoginComponent } from './login/login.component'; @NgModule({ declarations: [ AppComponent, HomeComponent, LoginComponent ], imports: [ BrowserModule, AppRoutingModule, FormsModule, ], providers: [AuthenticationService], bootstrap: [AppComponent] }) export class AppModule { }
11. Now guys we need to add below code inside app/app.component.html file:
<router-outlet></router-outlet>
12. Now guys we need to add below code inside src/index.html file:
<!doctype html> <html lang="en"> <head> <meta charset="utf-8"> <title>AuthGuardDemo</title> <base href="/"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="icon" type="image/x-icon" href="favicon.ico"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css"> <script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/popper.js/1.14.7/umd/popper.min.js"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/js/bootstrap.min.js"></script> </head> <body> <app-root></app-root> </body> </html>
Now we are done friends. If you have any kind of query, suggestion and new requirement then feel free to comment below.
Note: Friends, In this post, I just tell the basic setup and things, you can change the code according to your requirements.
I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad because with your views, I will make my next posts more good and helpful.
Jassa
Thanks
Hi,
I already have authGuard in my project (angular 13).
Some pages don’t have AuthGuard (like ErrorPage or PageNotFound).
I updated recently to Angular 14, and since, The ErrorPage or PageNotFound don’t work without AuthGuard, Each time I call an « anonymous page », it redirect to my login Page (Auth Guard is called).
Do you have the same problem ?
Tx
No it is working fine for me in Angular 14 as well.
Thanks