In Laravel 11, creating a user login and signup system can be efficiently managed using Laravel Breeze, a lightweight package that provides a simple implementation of all the authentication features you need, such as login, registration, password reset, and email verification.
Here’s a step-by-step guide on setting up user login and signup in Laravel 11 using Breeze:
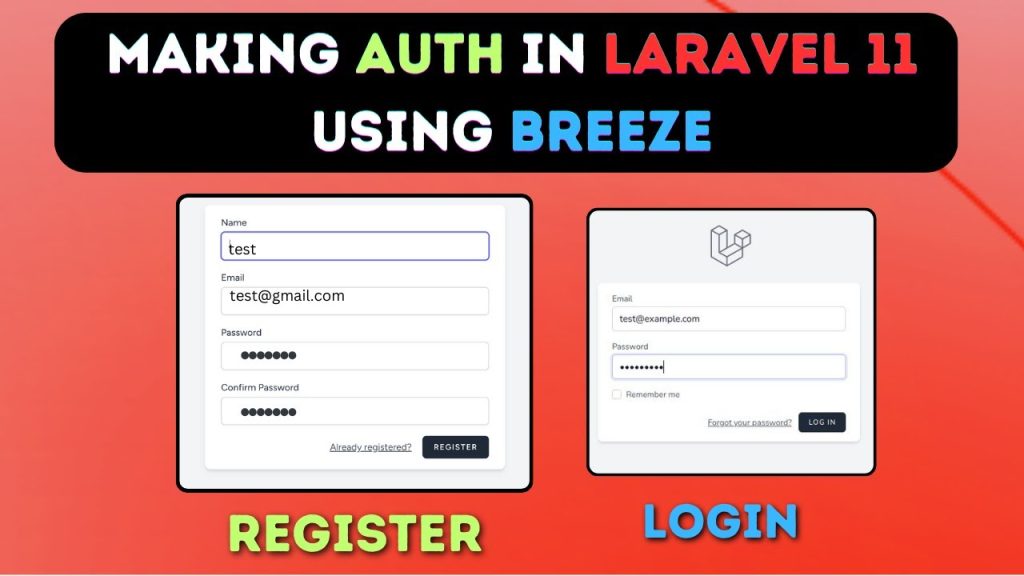
Step 1: Install Laravel
First, you need to create a new Laravel project if you haven’t already:
composer create-project --prefer-dist laravel/laravel your_project_name
Step 2: Set Up Environment
Configure your .env
file with the appropriate database settings:
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=your_database_name DB_USERNAME=your_database_username DB_PASSWORD=your_database_password
Step 3: Install Breeze
Install Laravel Breeze using Composer:
composer require laravel/breeze --dev
Once installed, run the Breeze install command:
php artisan breeze:install
This command will generate all the authentication scaffolding for your application.
Step 4: Run Migrations
Run the database migrations to create necessary tables (users, password_resets):
php artisan migrate
Step 5: Compile Assets
If you are using Breeze with Blade, you’ll need to compile your assets:
npm install npm run dev
For projects using Breeze with Vue or React, the commands are slightly different but follow a similar logic of installing dependencies and compiling assets.
Step 6: Start the Development Server
Start the Laravel development server:
php artisan serve
Step 7: Access the Authentication Pages
You can now access your application in a web browser. Breeze provides routes to all authentication views out of the box. Here are the default URLs:
- Login page:
http://localhost:8000/login
- Registration page:
http://localhost:8000/register
Both pages are fully functional, and users can register an account and log in to your application.
Optional: Email Verification
If you want to enable email verification, you need to implement the MustVerifyEmail contract in the User model:
- Open the
User
model usually located atapp/Models/User.php
. - Implement the
MustVerifyEmail
interface:
use Illuminate\Contracts\Auth\MustVerifyEmail; class User extends Authenticatable implements MustVerifyEmail { // Model content remains unchanged }
- Update the routes in
web.php
to ensure email verification is enforced:
Auth::routes(['verify' => true]);
Conclusion
Using Laravel Breeze, you have set up a basic but complete authentication system, including login, registration, and optionally, email verification. Breeze takes care of the backend logic and views, allowing you to customize them as needed for your application.