Hello to all, welcome to therichpost.com. In this post, I will tell you, How to validate angular 9 input phone number?
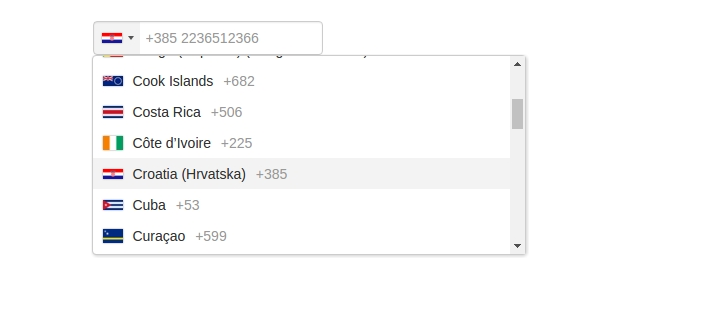
Post Working:
In this post, I am showing How to validate angular 9 input phone number? I also used Bootstrap 4 CDN for good looks of layout.
Here is the working coding steps and please follow carefully:
1. Here are the basics commands to install angular 9 on your system:
npm install -g @angular/cli ng new angularpopup //Create new Angular Project cd angularpopup // Go inside the Angular Project Folder ng serve --open // Run and Open the Angular Project http://localhost:4200/ // Working Angular Project Url
2. Here is the below command you need to run into your terminal to add intl tel input into your angular 9 application:
npm install ng2-tel-input intl-tel-input --save
3. Now you need to add below code into your angular.json file:
... "styles": ["node_modules/intl-tel-input/build/css/intlTelInput.css"], "scripts": ["node_modules/intl-tel-input/build/js/intlTelInput.min.js"] ...
4. Now you need to add below code into your src/app/app.module.ts file:
... import {Ng2TelInputModule} from 'ng2-tel-input'; import {FormsModule, ReactiveFormsModule} from '@angular/forms'; ... imports: [... Ng2TelInputModule, FormsModule, ReactiveFormsModule ...]
5. Now you need to add below code into your src/app/app.component.html file:
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.4.1/css/bootstrap.min.css"> <div class="container"> <h3 class="text-center mt-5 mb-5">Angular 9 input phone number with country code with validation working example</h3> <form [formGroup]="registerForm" (ngSubmit)="onSubmit()"> <div class="form-group"> <label for="phone">Phone Number:</label> <br> <input placeholder="1231231230" ng2TelInput (keypress)="numericOnly($event)" type="text" formControlName="phone" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.phone.errors }" /> <div *ngIf="submitted && f.phone.errors" class="invalid-feedback"> <div *ngIf="f.phone.errors.required">Phone is required</div> <div *ngIf="f.phone.errors.minlength">Phone must be at least 10 numbers.</div> </div> </div> <button type="submit" class="btn btn-primary">Submit</button> </form> </div>
6. Here is the code for app.component.css file:
.invalid-feedback{display:block;}
7. Here is the code for app.component.ts file:
import { Component, OnInit } from '@angular/core'; import { FormBuilder, FormGroup, Validators } from '@angular/forms'; @Component({ selector: 'app-inputtell', templateUrl: './inputtell.component.html', styleUrls: ['./inputtell.component.css'] }) export class AppComponent implements OnInit { registerForm: FormGroup; submitted = false; constructor(private formBuilder: FormBuilder) { } numericOnly(event) { let patt = /^([0-9])$/; let result = patt.test(event.key); return result; } ngOnInit(): void { this.registerForm = this.formBuilder.group({ phone: ['', [Validators.required, Validators.minLength(10)]] }); } get f() { return this.registerForm.controls; } onSubmit() { this.submitted = true; // stop here if form is invalid if (this.registerForm.invalid) { return; } } }
This is it and if you have any query related this post then please let me know.
Jassa
Thank you
pattern [0-9] working for India number..then how to validate other country numbers because some of the other countries have less than 10 or greater than 10 number.
Hi, can you please tell me any reference number then I will update my post according to that.
How to set values dynamically for this control
For dynamic value, you need bind the input with ng modal.
Thanks
If I’m using FormBuilder and ReactiveForms how can I bind this input with formControl?
I don’t want to use ngModel. Thanks in advance.
Yes and here is helpful link:
https://therichpost.com/angular-11-how-to-use-reactive-form-part-2-binding-data/