Hello guys, how are you? Welcome back to my blog therichpost.com. Guys today in this blog post we will Get WooCommerce products via rest api in React Native application.
To retrieve WooCommerce products via the REST API in a React Native application, follow these steps:
1. Install Dependencies
Guys now You’ll need to install axios
or any other HTTP client library, and react-native-dotenv
if you’re using environment variables for your WooCommerce API credentials.
npm install axios npm install react-native-dotenv
2. WooCommerce REST API Credentials
Guys First, ensure you have your WooCommerce REST API credentials, including the consumer key and consumer secret. You can generate these by going to your WordPress dashboard:
- Navigate to WooCommerce > Settings > Advanced > REST API.
- Click “Add Key”, give it a description, and select the user, then generate the key.
3. Create a .env
file
Store your WooCommerce API credentials securely.
WOOCOMMERCE_API_URL=https://yourwebsite.com/wp-json/wc/v3 WOOCOMMERCE_CONSUMER_KEY=your_consumer_key WOOCOMMERCE_CONSUMER_SECRET=your_consumer_secret
4. Set Up Axios for API Requests
Guys In your React Native app, create an API service file (WooCommerceApi.js
) to handle the requests.
WooCommerceApi.js
import axios from 'axios'; import { WOOCOMMERCE_API_URL, WOOCOMMERCE_CONSUMER_KEY, WOOCOMMERCE_CONSUMER_SECRET } from '@env'; const api = axios.create({ baseURL: WOOCOMMERCE_API_URL, auth: { username: WOOCOMMERCE_CONSUMER_KEY, password: WOOCOMMERCE_CONSUMER_SECRET, }, }); export const getProducts = async () => { try { const response = await api.get('/products'); return response.data; } catch (error) { console.error('Error fetching products:', error); throw error; } };
5. Calling the API in React Native Component
Guys Now, in your React Native component, you can call the getProducts
function and handle the data accordingly.
Example Component
import React, { useState, useEffect } from 'react'; import { View, Text, FlatList, ActivityIndicator } from 'react-native'; import { getProducts } from './WooCommerceApi'; const ProductsScreen = () => { const [products, setProducts] = useState([]); const [loading, setLoading] = useState(true); useEffect(() => { const fetchProducts = async () => { try { const data = await getProducts(); setProducts(data); } catch (error) { console.error('Failed to load products', error); } finally { setLoading(false); } }; fetchProducts(); }, []); if (loading) { return ( <View> <ActivityIndicator size="large" color="#0000ff" /> </View> ); } return ( <View> <FlatList data={products} keyExtractor={(item) => item.id.toString()} renderItem={({ item }) => ( <View> <Text>{item.name}</Text> <Text>{item.price}</Text> </View> )} /> </View> ); }; export default ProductsScreen;
6. Running the App
Guys Now you can run your React Native application, and it will fetch products from your WooCommerce store via the REST API and display them in a list.
npx react-native run-android # For Android npx react-native run-ios # For iOS
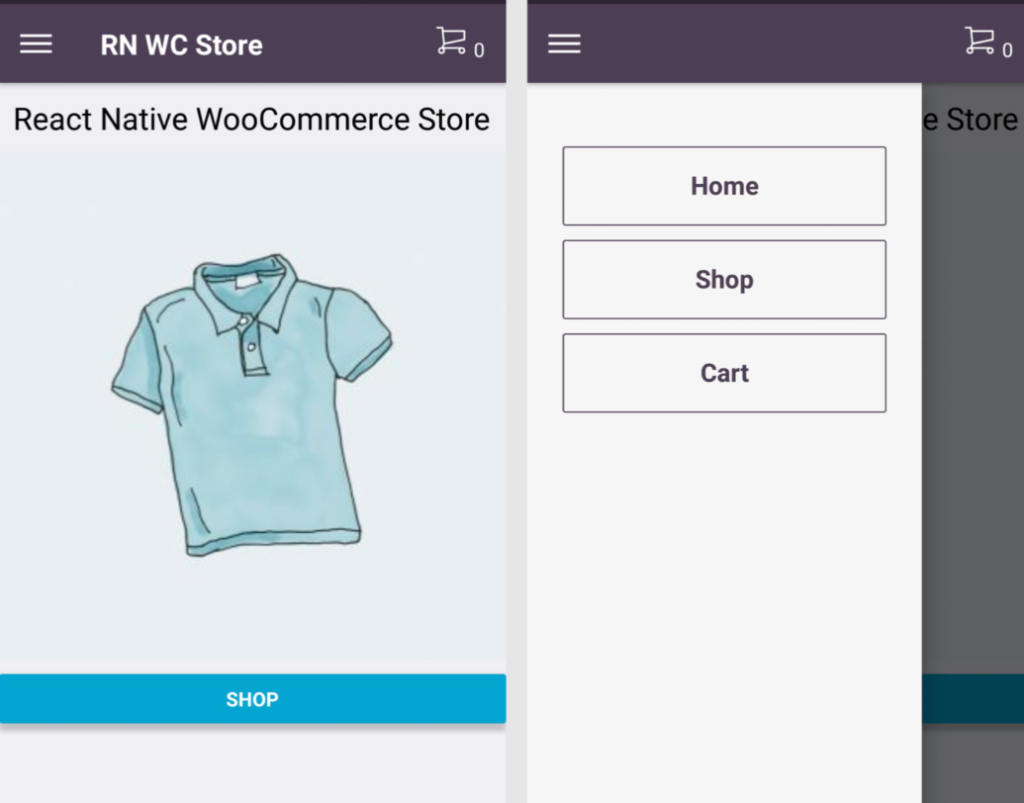
Notes:
- Ensure the API keys have sufficient permissions (read-only or read/write depending on your use case).
- If you’re using React Native CLI, remember to configure
.env
handling withreact-native-dotenv
properly.
This setup will help you successfully retrieve and display WooCommerce products in a React Native application. Guys if you will have any kind of query, suggestion and requirement then feel free to comment below.
Thanks
Ajay