Hello guys how are you? Welcome back to my blog. Today in this blog post we will create FlipKart Ecommerce Website Clone in React js.
For react js new comers, please check the below links:
1. Set Up the Project
First, make sure you have Node.js and npm (or yarn) installed. Then create a new React project.
npx create-react-app flipkart-clone cd flipkart-clone
2. Set Up the Project Structure
Organize your project files. Here’s a basic structure:
src/ ├── components/ │ ├── Header.js │ ├── Footer.js │ ├── Home.js │ └── ... ├── App.js └── index.js
3. Create Basic Components
Start by creating basic components such as Header, Footer, and a Home.
Home.js
import React from 'react'; const Home = ({ products }) => ( <main> <section className="banners mt-2 mt-md-0"> <div id="bannersCarousel" className="carousel slide" data-bs-ride="carousel"> <div className="carousel-inner"> <div className="carousel-item active"> <img src="assets/images/dc989bb97070430a.jpg?q=50" className="d-block w-100" alt="..." /> </div> <div className="carousel-item"> <img src="assets/images/b212a5700f9e4f1d.jpeg?q=50" className="d-block w-100" alt="..." /> </div> <div className="carousel-item"> <img src="assets/images/a96670a0535ba0b8.jpg?q=50" className="d-block w-100" alt="..." /> </div> </div> <button className="carousel-control-prev justify-content-start" type="button" data-bs-target="#bannersCarousel" data-bs-slide="prev"> <div className="prev-icon"> <i className="fa-solid fa-chevron-left fa-xl"></i> </div> <span className="visually-hidden">Previous</span> </button> <button className="carousel-control-next justify-content-end" type="button" data-bs-target="#bannersCarousel" data-bs-slide="next"> <div className="next-icon"> <i className="fa-solid fa-chevron-right fa-xl"></i> </div> <span className="visually-hidden">Next</span> </button> </div> </section> <section className="products"> <div className="electronic-products"> <div className="products-container container-fluid p-0"> <div className="category-card"> <p className="card-title">Shop for a Cool Summer</p> <button className="btn btn-primary card-btn mt-4">View All</button> </div> <div className="swiper mySwiper"> <div className="swiper-wrapper d-flex" id="electronic-products"> </div> <div className="swiper-button-next"> <div className="next-icon"> <i className="fa-solid fa-chevron-right fa-xl"></i> </div> </div> <div className="swiper-button-prev"> <div className="prev-icon"> <i className="fa-solid fa-chevron-left fa-xl"></i> </div> </div> </div> </div> </div> <div className="sport-products mt-3"> <div className="products-container container-fluid p-0"> <div className="category-card"> <p className="card-title">Sports, Healthcare & more</p> <button className="btn btn-primary card-btn mt-4">View All</button> </div> <div className="swiper mySwiper"> <div className="swiper-wrapper d-flex" id="sport-products"> </div> <div className="swiper-button-next"> <div className="next-icon"> <i className="fa-solid fa-chevron-right fa-xl"></i> </div> </div> <div className="swiper-button-prev"> <div className="prev-icon"> <i className="fa-solid fa-chevron-left fa-xl"></i> </div> </div> </div> </div> </div> <div className="summer-products mt-3"> <div className="products-container container-fluid p-0 "> <div className="category-card"> <p className="card-title">Best Of Electronics</p> <button className="btn btn-primary card-btn mt-4">View All</button> </div> <div className="swiper mySwiper"> <div className="swiper-wrapper d-flex" id="summer-products"> </div> <div className="swiper-button-next"> <div className="next-icon"> <i className="fa-solid fa-chevron-right fa-xl"></i> </div> </div> <div className="swiper-button-prev"> <div className="prev-icon"> <i className="fa-solid fa-chevron-left fa-xl"></i> </div> </div> </div> </div> </div> </section> </main> ); export default Home;
Header.js
import React from 'react'; const Header = () => ( <header> <nav className="navbar navbar-expand-lg bg-primary p-0"> <div className="container-lg"> <button className="navbar-toggler text-white border-0 btn-outline-dark" type="button" data-bs-toggle="collapse" data-bs-target="#navbarSupportedContent" aria-controls="navbarSupportedContent" aria-expanded="false" aria-label="Toggle navigation"> <img src="data:image/svg+xml;base64,PHN2ZyBmaWxsPSIjRkZGIiBoZWlnaHQ9IjM2IiB2aWV3Qm94PSIwIDAgMjQgMjQiIHdpZHRoPSIzNiIgeG1sbnM9Imh0dHA6Ly93d3cudzMub3JnLzIwMDAvc3ZnIj48cGF0aCBkPSJNMyAxOGgxOHYtMkgzdjJ6bTAtNWgxOHYtMkgzdjJ6bTAtN3YyaDE4VjZIM3oiLz48L3N2Zz4=" alt="" /> </button> <a className="navbar-brand explore" href="#"> <img src="assets/images/flipkart.png" alt="Flipkart" width="75" /> <p> Explore <span>Plus <img src="assets/images/plus.png" width="10" alt="" srcSet="" /></span> </p> </a> <div className="collapse navbar-collapse bg-primary z-3" id="navbarSupportedContent"> <form className="d-flex w-100" role="search"> <div className="input-group"> <input className="form-control b-0 br-0" type="search" placeholder="Search for products, brands and more" aria-label="Search" id="searchInp" /> <span className="input-group-text bg-white border-0 br-0"> <button className="border-0 br-2 bg-white"> <svg width="20" height="20" viewBox="0 0 17 18" className="" xmlns="http://www.w3.org/2000/svg"> <g fill="#2874F1" fillRule="evenodd"> <path className="_34RNph" d="m11.618 9.897l4.225 4.212c.092.092.101.232.02.313l-1.465 1.46c-.081.081-.221.072-.314-.02l-4.216-4.203"> </path> <path className="_34RNph" d="m6.486 10.901c-2.42 0-4.381-1.956-4.381-4.368 0-2.413 1.961-4.369 4.381-4.369 2.42 0 4.381 1.956 4.381 4.369 0 2.413-1.961 4.368-4.381 4.368m0-10.835c-3.582 0-6.486 2.895-6.486 6.467 0 3.572 2.904 6.467 6.486 6.467 3.582 0 6.486-2.895 6.486-6.467 0-3.572-2.904-6.467-6.486-6.467"> </path> </g> </svg> </button> </span> </div> </form> <ul className="navMenu navbar-nav me-auto mb-2 mb-lg-0 d-flex align-items-center justify-content-around w-100"> <li className="nav-item"> <a className="btn btn-white nav-link active" aria-current="page" id="loginBtn" href="#">Login</a> </li> <li className="nav-item"> <a className="nav-link" href="#">Become a Seller</a> </li> <li className="nav-item dropdown"> <a className="nav-link dropdown-toggle" href="#" role="button" data-bs-toggle="dropdown" aria-expanded="false"> More </a> <ul className="dropdown-menu"> <li> <a className="dropdown-item" href="#"> <i> <svg xmlns="http://www.w3.org/2000/svg" width="16" height="14" className="" viewBox="0 0 12 14"> <g fill="none" fillRule="evenodd"> <path d="M-4-3h20v20H-4z"></path> <path fill="#2874F1" d="M6.17 13.61c-1.183 0-1.922-.723-1.922-1.88H8.09c0 1.157-.74 1.88-1.92 1.88zm4.222-5.028l1.465 1.104v1.07H0v-1.07l1.464-1.104v-2.31h.004c.035-2.54 1.33-4.248 3.447-4.652V.992C4.915.446 5.37 0 5.928 0c.558 0 1.014.446 1.014.992v.628c2.118.404 3.412 2.112 3.446 4.65h.004v2.312z"> </path> </g> </svg> </i> Notification Preferences </a> </li> <li> <hr className="dropdown-divider" /> </li> <li> <a className="dropdown-item" href="#"> <i> <svg xmlns="http://www.w3.org/2000/svg" width="16" height="16" className="" viewBox="0 0 14 17"> <g fill="none" fillRule="evenodd"> <path fill="#2874F0" fillRule="nonzero" d="M12.25.542H1.75c-.833 0-1.5.675-1.5 1.5v10.5c0 .825.667 1.5 1.5 1.5h3L7 16.292l2.25-2.25h3c.825 0 1.5-.675 1.5-1.5v-10.5c0-.825-.675-1.5-1.5-1.5zm-4.5 12h-1.5v-1.5h1.5v1.5zM9.303 6.73l-.676.69c-.54.547-.877.997-.877 2.122h-1.5v-.375c0-.825.338-1.575.877-2.123l.93-.945c.278-.27.443-.646.443-1.058 0-.825-.675-1.5-1.5-1.5s-1.5.675-1.5 1.5H4c0-1.658 1.342-3 3-3s3 1.342 3 3c0 .66-.27 1.26-.697 1.687z"> </path> </g> </svg> </i> 24x7 Customer Care </a> </li> <li> <hr className="dropdown-divider" /> </li> <li> <a className="dropdown-item" href="#"> <i> <svg xmlns="http://www.w3.org/2000/svg" width="16" height="14" className="" viewBox="0 0 18 10"> <g fill="none" fillRule="evenodd"> <path fill="#2874F0" fillRule="nonzero" d="M12.333 0l1.91 1.908-4.068 4.067-3.333-3.333L.667 8.825 1.842 10l5-5 3.333 3.333 5.25-5.24L17.333 5V0"> </path> </g> </svg> </i> Advertise </a> </li> <li> <hr className="dropdown-divider" /> </li> <li> <a className="dropdown-item" href="#"> <i> <svg xmlns="http://www.w3.org/2000/svg" width="16" height="14" className="" viewBox="0 0 12 14"> <g fill="none" fillRule="evenodd"> <path d="M-4-3h20v20H-4z"></path> <path fill="#2874F0" fillRule="nonzero" d="M12 4.94H8.57V0H3.43v4.94H0l6 5.766 6-5.765zM0 12.354V14h12v-1.647H0z"> </path> </g> </svg> </i> Download </a> </li> </ul> </li> <li className="nav-item"> <a className="nav-link" href="#"> <i> <svg className="V3C5bO" width="14" height="14" viewBox="0 0 16 16" xmlns="http://www.w3.org/2000/svg"> <path className="_1bS9ic" d="M15.32 2.405H4.887C3 2.405 2.46.805 2.46.805L2.257.21C2.208.085 2.083 0 1.946 0H.336C.1 0-.064.24.024.46l.644 1.945L3.11 9.767c.047.137.175.23.32.23h8.418l-.493 1.958H3.768l.002.003c-.017 0-.033-.003-.05-.003-1.06 0-1.92.86-1.92 1.92s.86 1.92 1.92 1.92c.99 0 1.805-.75 1.91-1.712l5.55.076c.12.922.91 1.636 1.867 1.636 1.04 0 1.885-.844 1.885-1.885 0-.866-.584-1.593-1.38-1.814l2.423-8.832c.12-.433-.206-.86-.655-.86" fill="#fff"></path> </svg> </i> Cart </a> </li> </ul> </div> </div> </nav> </header> ); export default Header;
Footer.js
import React from 'react'; const Footer = () => ( <footer> <p>© 2024 My E-commerce Website</p> </footer> ); export default Footer;
4. Guys here is git repo link from where you will get images, css and js folders and placed inside public/assets folder:
5. Guys here code for App.js file:
import React, { useState, useEffect } from 'react'; import Header from './components/Header'; import Footer from './components/Footer'; import Home from './components/Home'; const App = () => { return ( <div className="App"> <Header /> <Home /> <Footer /> </div> ); }; export default App;
6. Start the Development Server
Start the React development server:
npm start
This will launch your application in development mode. By default, it should open a new tab in your browser at http://localhost:3000
, displaying your Flipkart clone.
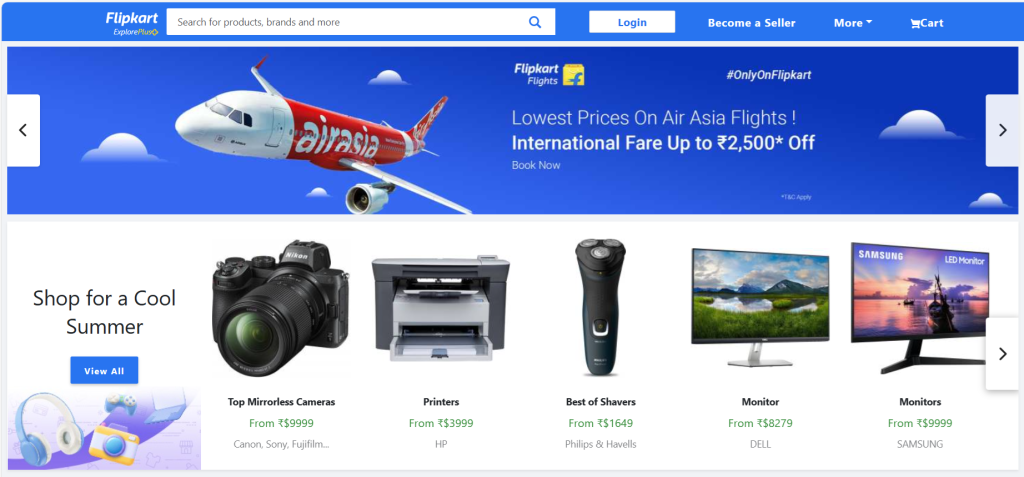
This is it guys and if you will have any kind of query, suggestions or requirements then feel free to comment below.
Thanks