Hello friends, welcome back to my blog. Today this blog post will tell you, Angular 13 Bootstrap 5 Modal Popup Forms with Validation Working Example.
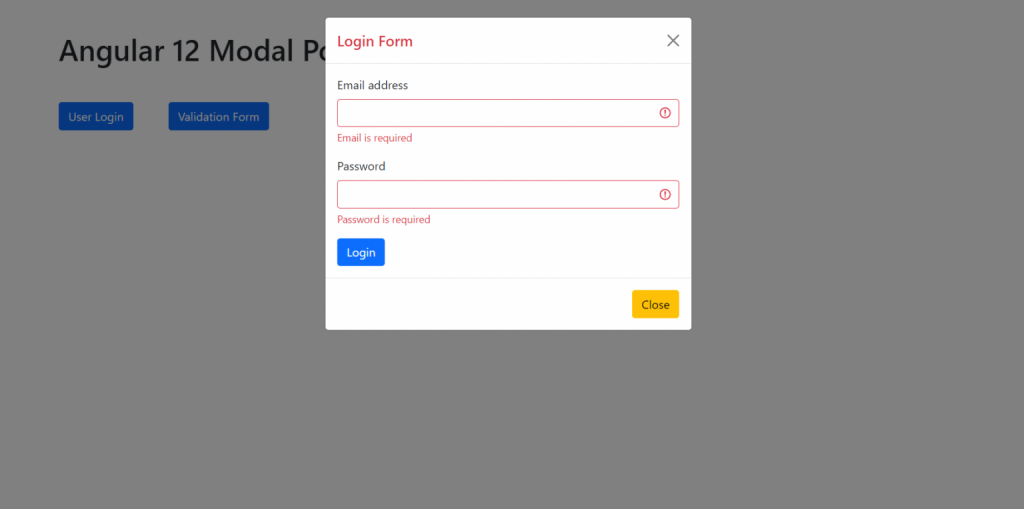
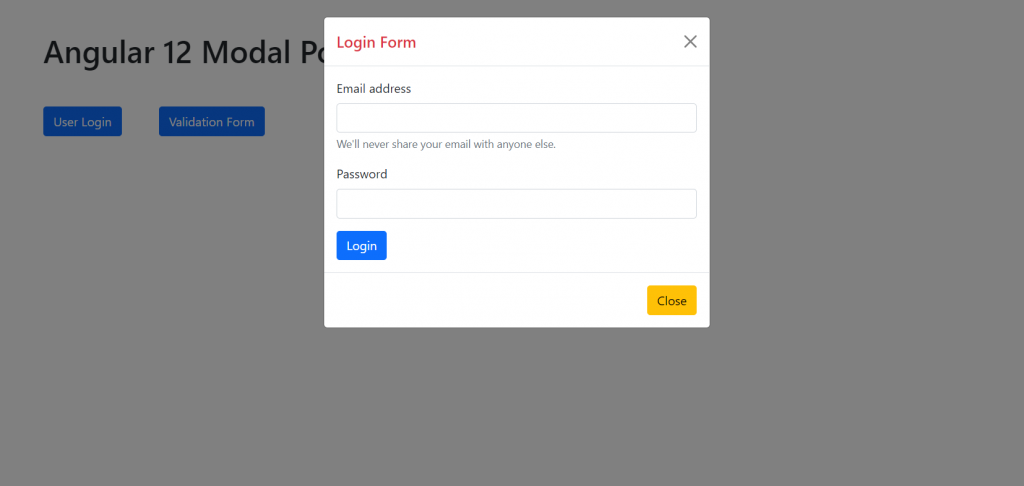
Angular13 came and Bootstrap 5 also. If you are new then you must check below two links:
Friends now I proceed onwards and here is the working code snippet and please use carefully this to avoid the mistakes:
1. Firstly friends we need fresh angular 13 setup and for this we need to run below commands but if you already have angular 13 setup then you can avoid below commands. Secondly we should also have latest node version installed on our system:
npm install -g @angular/cli ng new angularboot5 //Create new Angular Project cd angularboot5 // Go inside the Angular Project Folder
2. Now friends we need to run below commands into our project terminal to install bootstrap 5 modules into our angular application:
npm install bootstrap
3. Now friends we just need to add below code into angularboot5/src/app/app.component.html file to get final out on the web browser:
<button type="button" class="btn btn-primary me-5" data-bs-toggle="modal" data-bs-target="#exampleModal"> User Login </button> <button type="button" class="btn btn-primary" data-bs-toggle="modal" data-bs-target="#exampleModal2"> Validation Form </button> <!-- Modal --> <div class="modal fade" id="exampleModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <h5 class="modal-title text-danger" id="exampleModalLabel">Login Form</h5> <button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button> </div> <div class="modal-body"> <form> <div class="mb-3"> <label for="exampleInputEmail1" class="form-label">Email address</label> <input type="email" class="form-control" id="exampleInputEmail1" aria-describedby="emailHelp"> <div id="emailHelp" class="form-text">We'll never share your email with anyone else.</div> </div> <div class="mb-3"> <label for="exampleInputPassword1" class="form-label">Password</label> <input type="password" class="form-control" id="exampleInputPassword1"> </div> <button type="button" class="btn btn-primary">Login</button> </form> </div> <div class="modal-footer"> <button type="button" class="btn btn-warning" data-bs-dismiss="modal">Close</button> </div> </div> </div> </div> <!-- Modal 2 with validation--> <div class="modal fade" id="exampleModal2" tabindex="-1" aria-labelledby="exampleModal2Label" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <h5 class="modal-title text-danger" id="exampleModal2Label">Login Form</h5> <button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button> </div> <div class="modal-body"> <form [formGroup]="registerForm" (ngSubmit)="onSubmit()"> <div class="mb-3"> <label for="exampleInputEmail1" class="form-label">Email address</label> <input type="email" formControlName="email" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.email.errors }" id="inputEmail4"> <div *ngIf="submitted && f.email.errors" class="invalid-feedback"> <div *ngIf="f.email.errors.required">Email is required</div> <div *ngIf="f.email.errors.email">Email must be a valid email address</div> </div> </div> <div class="mb-3"> <label for="exampleInputPassword1" class="form-label">Password</label> <input type="password" formControlName="password" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.password.errors }" id="inputPassword4"> <div *ngIf="submitted && f.password.errors" class="invalid-feedback"> <div *ngIf="f.password.errors.required">Password is required</div> </div> </div> <button type="submit" class="btn btn-primary">Login</button> </form> </div> <div class="modal-footer"> <button type="button" class="btn btn-warning" data-bs-dismiss="modal">Close</button> </div> </div> </div> </div>
4. Now friends we just need to add below code into angularboot5/angular.json file:
"styles": [ ... "node_modules/bootstrap/dist/css/bootstrap.min.css" ], "scripts": [ ... "node_modules/bootstrap/dist/js/bootstrap.min.js" ]
5. Now friends we just need to add below code into angularboot5/src/app/app.module.ts file for reactive forms:
... import { ReactiveFormsModule } from '@angular/forms'; @NgModule({ ... imports: [ ... ReactiveFormsModule, ],
6. Now friends we just need to add below code into angularboot5/src/app/app.component.ts file to validation and other reactive form functions:
... import { FormBuilder, FormGroup, Validators } from '@angular/forms'; export class AppComponent { //Form Validables registerForm: FormGroup; submitted = false; constructor( private formBuilder: FormBuilder){} //Add user form actions get f() { return this.registerForm.controls; } onSubmit() { this.submitted = true; // stop here if form is invalid if (this.registerForm.invalid) { return; } //True if all the fields are filled if(this.submitted) { alert("Great!!"); } } ngOnInit() { //Add User form validations this.registerForm = this.formBuilder.group({ email: ['', [Validators.required, Validators.email]], password: ['', [Validators.required]], }); } }
Friends in the end must run ng serve command into your terminal to run the angular 13 project(localhost:4200).
Now we are done friends. If you have any kind of query, suggestion and new requirement then feel free to comment below.
Note: Friends, In this post, I just tell the basic setup and things, you can change the code according to your requirements.
I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad because with your views, I will make my next posts more good and helpful.
Jassa
Thanks