Hello to all, welcome to therichpost.com In this post, I will tell you, Angular 17 data table with print csv excel copy buttons.
This post next part : Angular datatables with custom button event click open bootstrap modal popup
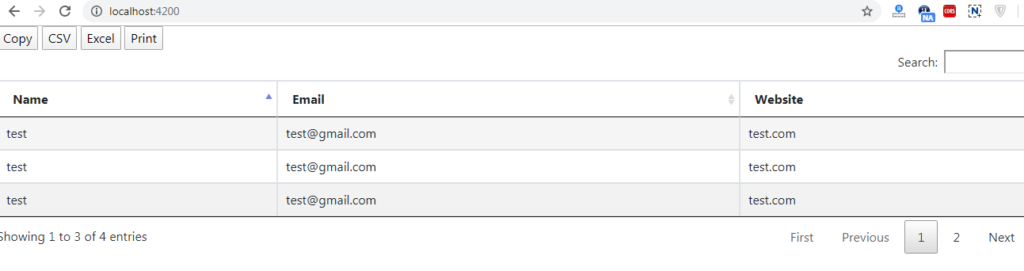
Today, you see angular 8 datatables with csv, excel, copy and print buttons. I have used bootstrap also for good looks.
Here are complete steps and please follow carefully:
1. Here are the basics commands to install angular 8 on your system:
npm install -g @angular/cli ng new angularpopup //Create new Angular Project $ cd angularpopup // Go inside the Angular Project Folder ng serve --open // Run and Open the Angular Project http://localhost:4200/ // Working Angular Project Url
2. After done with above, you need to run below commands to set bootstrap, datatable, jquery environment into your angular 8 application:
npm install jquery --save npm install datatables.net --save npm install datatables.net-dt --save npm install angular-datatables --save npm install @types/jquery --save-dev npm install @types/datatables.net --save-dev npm install ngx-bootstrap bootstrap --save npm install datatables.net-buttons --save npm install datatables.net-buttons-dt --save npm install @types/datatables.net-buttons --save-dev npm install jszip --save
3. Now you need to add below code into your angular.json file:
... "styles": [ "src/styles.css", "node_modules/datatables.net-dt/css/jquery.dataTables.css", "node_modules/bootstrap/dist/css/bootstrap.min.css", ], "scripts": [ "node_modules/jquery/dist/jquery.js", "node_modules/datatables.net/js/jquery.dataTables.js", "node_modules/bootstrap/dist/js/bootstrap.js", "node_modules/jszip/dist/jszip.js", "node_modules/datatables.net-buttons/js/dataTables.buttons.js", "node_modules/datatables.net-buttons/js/buttons.colVis.js", "node_modules/datatables.net-buttons/js/buttons.flash.js", "node_modules/datatables.net-buttons/js/buttons.html5.js", "node_modules/datatables.net-buttons/js/buttons.print.js" ] ...
4. Now you need to add below code into your src/app/app.module.ts file:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppComponent } from './app.component'; import {DataTablesModule} from 'angular-datatables'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, DataTablesModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
4. Now you need to add below code into your src/app/app.component.ts file:
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { public data = [ {name: 'test', email: 'test@gmail.com', website:'test.com'}, {name: 'test', email: 'test@gmail.com', website:'test.com'}, {name: 'test', email: 'test@gmail.com', website:'test.com'}, {name: 'test', email: 'test@gmail.com', website:'test.com'}, ]; title = 'angulardatatables'; dtOptions: any = {}; ngOnInit() { this.dtOptions = { pagingType: 'full_numbers', pageLength: 3, processing: true, dom: 'Bfrtip', buttons: [ 'copy', 'csv', 'excel', 'print' ] }; } }
5. Now you need to add below code into src/app/app.component.html file:
<table class="table table-striped table-bordered table-sm row-border hover" datatable [dtOptions]="dtOptions"> <thead> <tr> <th>Name</th> <th>Email</th> <th>Website</th> </tr> </thead> <tbody> <tr *ngFor="let group of data"> <td>{{group.name}}</td> <td>{{group.email}}</td> <td>{{group.website}}</td> </tr> </tbody> </table>
In the end, don’t forgot to run ng serve command. If you have any query then do comment below.
Jassa
Thank you.
[…] This post first part : Angular datatable with print csv excel copy buttons […]
Hi.. I have done all the steps but still,buttons are not visible for excel pdf etc…
you must missed some library. Please check this:
https://therichpost.com/how-to-implement-datatable-with-print-excel-csv-buttons-in-angular-10/
This is an awesome piece. It works like magic.
Thanks a lot.
Thank you for lovely comment.
This is not working
I have followed your steps line by line.
Hi, are you using angular 10? Here is the updated version:
https://therichpost.com/how-to-implement-datatable-with-print-excel-csv-buttons-in-angular-10/
It give error in console TypeError: $(…).DataTable is not a function
https://therichpost.com/how-to-implement-datatable-with-print-excel-csv-buttons-in-angular-10/
Hi,
for me it is working good in development environment (ng serve in VS Code) but I got errors initializing the datatable by constructor when I run ng build for distribution. Can you help please?
Hi, can you please share your constructor code?
Hi,
solved adding those imports in typescript component,
import ‘datatables.net-buttons/js/buttons.html5’;
import * as $ from ‘jquery’;
import ‘datatables.net’;
import ‘datatables.net-buttons’;
and this is the constructor:
$(‘#dcDetailTable’).DataTable({
lengthChange: false,
searching: false,
destroy: true,
info: false,
paging: false,
ordering: this.pageSearchDcSp.codiceDataCenter ? false : true,
data: this.dcDetailData,
columns: this.dcDetailColList,
dom: ‘Bfrtip’,
buttons: [
{ extend: ‘csvHtml5’, className: ‘btn btn-link px-3 py-1 pi-yellow border-button-rounded’ }
]
});
Cheers.
Hi, I have performed all the steps mentioned above but can’t see any of the buttons with the datatable. However, My code has no errors and it is running fine but these buttons are not there.
https://therichpost.com/how-to-implement-datatable-with-print-excel-csv-buttons-in-angular-10/