Hello to all welcome back on my blog therichpost.com. Today in this blog post, I am going to tell you Angular 12 Bootstrap 5 Ecommerce Testing Project – Part 2.
Guys before starting this please check part 1 for basic setup like project setup, bootstrap installation, components building.
Post working information and working video:
- In this post we will get products from web api and guys you can also use this web api into your demo projects.
- We will do Angular 12 dynamic routing based upon ID for single product page.
- Angular ngFor with items slicing for realted product section.
- In next part we will do add to cart functionality.
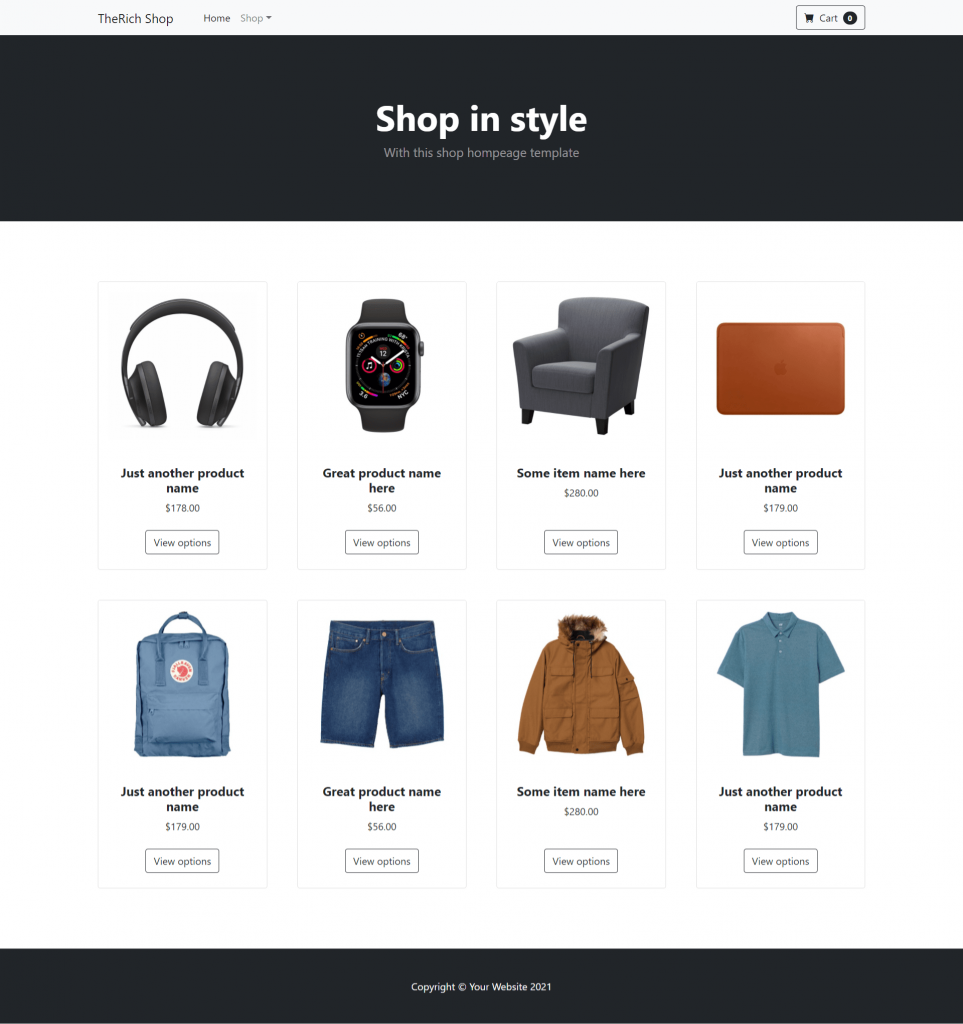
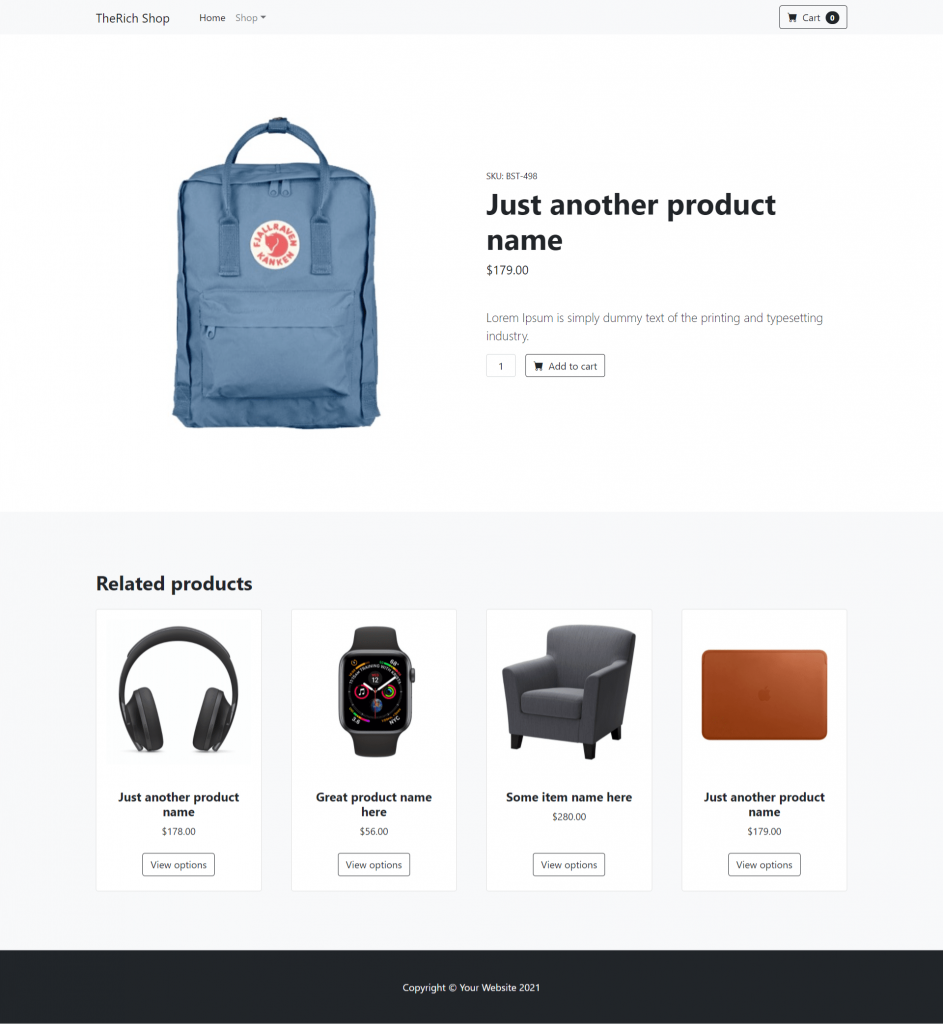
Guys Angular12 and Bootstrap5 came and if you are new in both or want to learn more about them then please check below tutorial links:
Guy’s here is working code snippet and please follow it carefully to avoid the mistakes:
1. Now guy’s, now we need to add or replace below code into our angularecom/src/app/app-routing.module.ts file to make single item route dynamic:
... const routes: Routes = [ //Dynamic item page route { path: 'item-page/:id', component: SingleitemComponent } ]; ...
2. Now guy’s, now we need to add below code into our angularecom/src/app/app-module.ts file:
... import { HttpClientModule } from '@angular/common/http'; ... @NgModule({ ... imports: [ ... HttpClientModule ],
3. Now guys, now we need to add below code into our angularecom/src/app/home/home.component.ts file to make request for getting api data:
... import { HttpClient } from '@angular/common/http'; export class HomeComponent implements OnInit { ... products : any; constructor(private http: HttpClient){ //get request this.http.get('https://www.testjsonapi.com/products/').subscribe(data => { //data storing for use in html component this.products = data; }, error => console.error(error)); } }
4. Now guys, now we need to add below code into our angularecom/src/app/home/home.component.html file:
<header class="bg-dark py-5"> <div class="container px-4 px-lg-5 my-5"> <div class="text-center text-white"> <h1 class="display-4 fw-bolder">Shop in style</h1> <p class="lead fw-normal text-white-50 mb-0">With this shop hompeage template</p> </div> </div> </header> <!--main page--> <section class="py-5"> <div class="container px-4 px-lg-5 mt-5"> <div class="row gx-4 gx-lg-5 row-cols-2 row-cols-md-3 row-cols-xl-4 justify-content-center"> <!-- Angular ngFor loop for getting dynamic data in looping --> <div class="col mb-5" *ngFor="let data of products"> <div class="card h-100"> <!-- Product image--> <img class="card-img-top" src={{data.product_image}} alt={{data.product_title}}> <!-- Product details--> <div class="card-body p-4"> <div class="text-center"> <!-- Product name with dynamic route linking--> <a routerLink="/item-page/{{data.id}}"><h5 class="fw-bolder">{{data.product_title}}</h5></a> <!-- Product price--> {{data.product_price}} </div> </div> <!-- Product actions--> <div class="card-footer p-4 pt-0 border-top-0 bg-transparent"> <div class="text-center"><a class="btn btn-outline-dark mt-auto" href="#">View options</a></div> </div> </div> </div> </div> </div> </section>
5. Now guys, now we need to add below code into our angularecom/src/app/singleitem/singleitem.component.ts file. In this we will get dynamic ID and show single item accoding to that ID:
... import {Router, ActivatedRoute, Params} from '@angular/router'; //ActivatedRoute module to get dynamicid from route import { HttpClient } from '@angular/common/http'; export class SingleitemComponent implements OnInit { ... id:any; product:any; products:any; constructor(private activatedRoute: ActivatedRoute, private http: HttpClient) { //getting and storing dynamic ID this.id = this.activatedRoute.snapshot.paramMap.get('id'); //Single Product WEB API this.http.get('https://www.testjsonapi.com/product/'+this.id).subscribe(data => { //data storing for use in html component this.product = data; }, error => console.error(error)); } ngOnInit(): void { //get request for all the products for realted product section. this.http.get('https://www.testjsonapi.com/products/').subscribe(data => { //data storing for use in html component this.products = data; }, error => console.error(error)); } }
6. Now guys, now we need to add below code into our angularecom/src/app/singleitem/singleitem.component.html file:
<!--single page--> <section class="py-5"> <div class="container px-4 px-lg-5 my-5"> <div class="row gx-4 gx-lg-5 align-items-center"> <div class="col-md-6"><img class="card-img-top mb-5 mb-md-0" src={{product?.product_image}} alt={{product?.product_title}}></div> <div class="col-md-6"> <div class="small mb-1">SKU: BST-498</div> <h1 class="display-5 fw-bolder">{{product?.product_title}}</h1> <div class="fs-5 mb-5"> <span>{{product?.product_price}}</span> </div> <p class="lead">{{product?.product_description}}</p> <div class="d-flex"> <input class="form-control text-center me-3" id="inputQuantity" type="num" value="1" style="max-width: 3rem"> <button class="btn btn-outline-dark flex-shrink-0" type="button"> <i class="bi-cart-fill me-1"></i> Add to cart </button> </div> </div> </div> </div> </section> <!-- Realted Product Section --> <section class="py-5 bg-light"> <div class="container px-4 px-lg-5 mt-5"> <h2 class="fw-bolder mb-4">Related products</h2> <div class="row gx-4 gx-lg-5 row-cols-2 row-cols-md-3 row-cols-xl-4 justify-content-center"> <!-- Products looping and Slice code show only 4 products --> <div class="col mb-5" *ngFor="let data of products | slice:0:4 "> <div class="card h-100"> <!-- Product image--> <img class="card-img-top" src={{data.product_image}} alt={{data.product_title}}> <!-- Product details--> <div class="card-body p-4"> <div class="text-center"> <!-- Product name--> <h5 class="fw-bolder">{{data.product_title}}</h5> <!-- Product price--> {{data.product_price}} </div> </div> <!-- Product actions--> <div class="card-footer p-4 pt-0 border-top-0 bg-transparent"> <div class="text-center"><a class="btn btn-outline-dark mt-auto" href="#">View options</a></div> </div> </div> </div> </div> </div> </section>
Guy’s in the end please run ng serve command to check the out on browser(localhost:4200) and if you will have any query then feel free to comment below.
Guy’s I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad because with your views, I will make my next posts more good and helpful.
Jassa
Thanks
i am getting an error on singleitem.component.html
Error :Object is of type ‘unknown’.ngtsc(2571)
For those variable in the HTML file data.product_image/data.product_title
Did you follow the Part 1 properly?
Great, very helpful for my college project and waiting for part 3.
Thanks.
Thanks and third part will come soon.
API request is working fine except in singleitem.comp.html .Interpolation is showing unknown error
I updated that file now and please use and check then.
Thanks.
Awesome and thanks.
You are welcome.
Hi, The First Part is Awesome, but the Second, i have the problem where is showing Object Unknow in the single product, but i see is not having receive data from api. In the last reply you say this problem is resolve but i cant know where are this change. I waiting for you answer, thanks.
Are you getting data from API?
Same I get it too