Hello guys how are you? Welcome back on my blog. Today we will learn In React, sharing data between server-side components generally refers to managing and passing data within a server-rendered React application, such as those using Next.js or other similar frameworks. Here’s a step-by-step guide on how you can efficiently share data between server-side components in a React application:
- For react js and next js new comers, please check the below link:
- React js Basic Tutorials
- Next js Basic Tutorials
- Free React Admin Templates
- Free React Ecommerce Templates
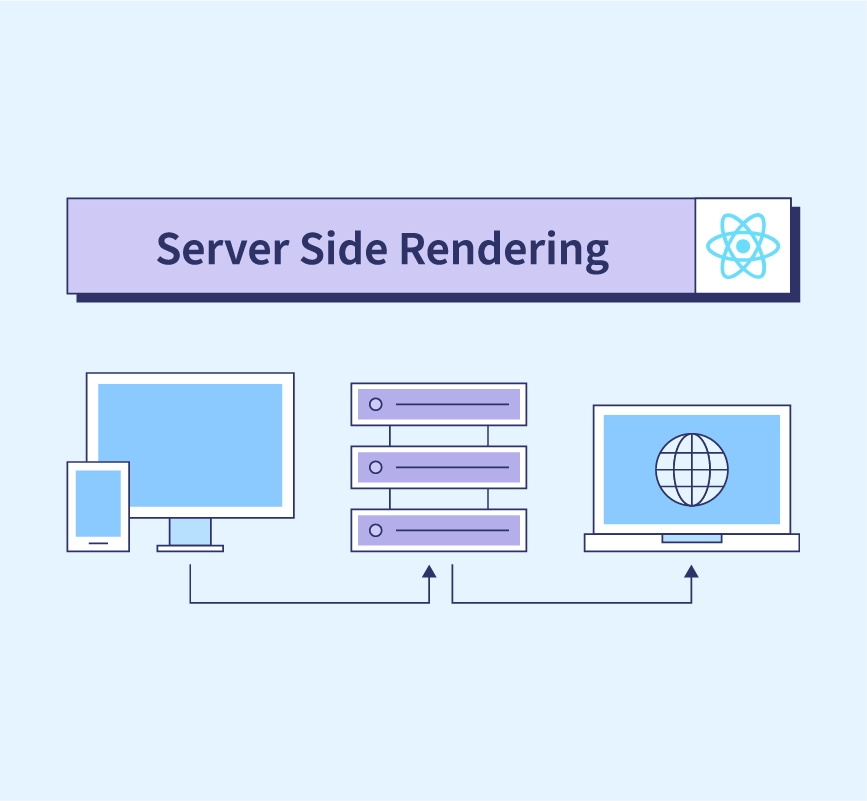
1. Using React Context for Server-Side Rendering (SSR)
Guys React Context is a great way to pass data through the component tree without having to pass props down manually at every level. In a server-rendered app, you can use React Context to share data that is fetched server-side.
Example using Next.js:
// Create a context const DataContext = React.createContext(null); // Server-side data fetching and passing it into the context function MyApp({ Component, pageProps, serverData }) { return ( <DataContext.Provider value={serverData}> <Component {...pageProps} /> </DataContext.Provider> ); } // Export getServerSideProps to fetch data on server export async function getServerSideProps(context) { const serverData = await fetchData(); return { props: { serverData } }; } function fetchData() { // Fetch your data here return { key: 'value' }; }
2. Prop Drilling
Guys Although not ideal for deeply nested components, prop drilling is a straightforward method to share data by passing props from a parent component down to its descendants.
function ParentComponent(props) { const data = useServerData(); // Custom hook to fetch server-side data return <ChildComponent data={data} />; } function ChildComponent({ data }) { return <div>{data.key}</div>; }
3. Higher Order Components (HOC)
Guys Higher Order Components can be used to wrap components and provide them with additional data props. This is useful for enhancing components with data fetched on the server.
function withServerData(Component) { return function WrappedComponent(props) { const serverData = useServerData(); // Fetch data on server return <Component {...props} serverData={serverData} />; }; } // Usage const EnhancedComponent = withServerData(ChildComponent);
4. Custom Hooks
Guys Creating a custom hook to fetch and provide data can make your components cleaner and promote code reuse.
function useServerData() { const [data, setData] = React.useState(null); React.useEffect(() => { async function fetchData() { const response = await fetch('/api/data'); const json = await response.json(); setData(json); } fetchData(); }, []); return data; }
5. State Management Libraries
Guys For complex applications, consider using state management libraries like Redux or MobX. These libraries can manage state outside the component tree and can be integrated with server-side rendering.
// Redux example with Next.js import { Provider } as 'react-redux'; import store from './store'; function MyApp({ Component, pageProps }) { return ( <Provider store={store}> <Component {...pageProps} /> </Provider> ); }
6. Next.js (when using React for SSR)
Guys If you are using Next.js, which is a popular framework for React applications that support SSR, you can use its built-in capabilities for fetching data and passing it through components.
Example using getServerSideProps
in Next.js:
// In a Next.js page component export async function getServerSideProps(context) { const data = await fetchData(); // Fetch your data here return { props: { data } }; // Pass data to the component as props } function Page({ data }) { // Use data as props in your component return <div>{data}</div>; } export default Page;
When implementing any of these strategies in server-rendered applications, it’s important to ensure that any global state or context provided at the top level is correctly serialized and rehydrated on the client-side. This ensures a smooth transition from server-rendered content to client-side interactivity.
Guys if you will have any kind of query then feel free to comment below.
Thanks
Jas
Recent Comments