Hello friends, welcome back to my blog. Today in this blog post, I am going to show you, Reactjs Form Validation Working Demo.
In this we will do:
- Email Format Validation.
- Confirm Passwords Validation.
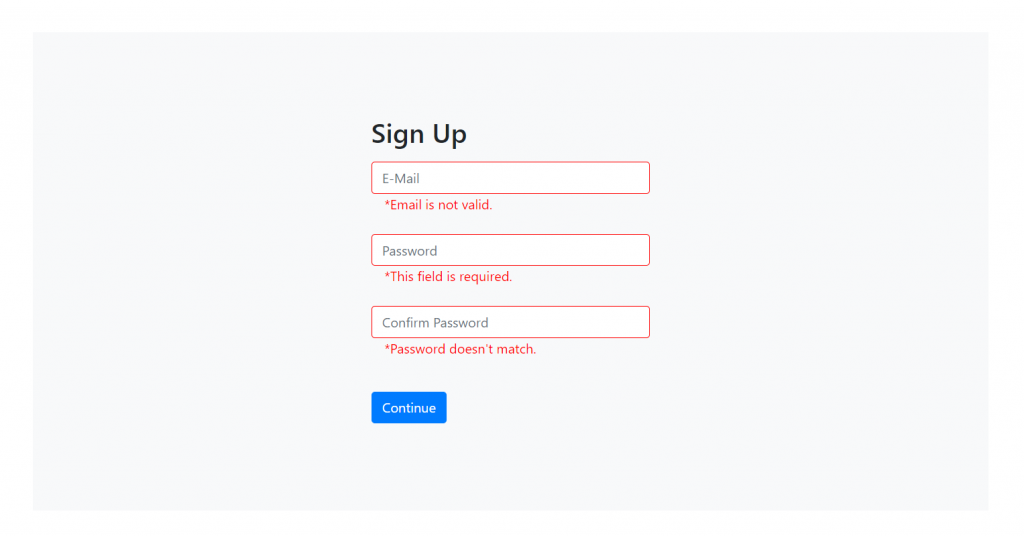
For reactjs new comers, please check the below link:
Friends now I proceed onwards and here is the working code snippet for Reactjs Form Validation Working Demo and please use this carefully to avoid the mistakes:
1. Firstly, we need fresh reactjs setup and for that, we need to run below commands into out terminal and also we should have latest node version installed on our system:
npx create-react-app reacttemplate cd reacttemplate npm start
2. Now we need to run below commands into our project terminal to get bootstrap and related modules into our reactjs application:
npm install bootstrap --save npm start //For start project again
3. Finally for the main output, we need to add below code into our reacttemplate/src/App.js file or if you have fresh setup then you can replace reacttemplate/src/App.js file code with below code:
import React from "react"; import './App.css' import 'bootstrap/dist/css/bootstrap.min.css'; class App extends React.Component { //Settings the varaibles constructor(props) { super(props); this.state = { fields: {}, errors: {} } } //Handle Validation handleValidation() { let fields = this.state.fields; let errors = {}; let formIsValid = true; //Email Validation if (!fields["email"]) { formIsValid = false; errors["email"] = "*This field is required." } function validateEmail(email) { const re = /^(([^<>()[\]\\.,;:\s@\"]+(\.[^<>()[\]\\.,;:\s@\"]+)*)|(\".+\"))@((\[[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\.[0-9]{1,3}\])|(([a-zA-Z\-0-9]+\.)+[a-zA-Z]{2,}))$/; return re.test(email); } //Email valid validation if (typeof fields["email"] != "undefined") { if (!validateEmail(fields["email"])) { formIsValid = false; errors["email"] = "*Email is not valid." } } //Pssword Validation if (!fields["password"]) { formIsValid = false; errors["password"] = "*This field is required." } //Confirm Password Validation if (!fields["cpassword"]) { formIsValid = false; errors["cpassword"] = "*This field is required." } //Confirm Password Match Validation if (fields["password"] !== fields["cpassword"]) { formIsValid = false; errors["cpassword"] = "*Password doesn't match." } //setting the errors messages this.setState({ errors: errors }); return formIsValid; } contactSubmit(e) { e.preventDefault(); if (this.handleValidation()) { alert("Success"); } else { // alert("Error"); } } //this will set and get value from inputs fields handleChange(field, e) { let fields = this.state.fields; fields[field] = e.target.value; this.setState({ fields }); } render() { return ( <div className="main_container"> <div className="container"> <div className="row h-100 justify-content-center align-items-center bg-light mt-5"> <div className="col-4 mt-5 mb-5 pt-5 pb-4 pl-4 pr-4"> <h2 className="text-left mt-1 mb-3">Sign Up</h2> <form name="contactform" onSubmit={this.contactSubmit.bind(this)}> <div className="form-group pb-2"> <input borderColor={this.state.errors["email"] ? "red" : ""} type="text" className="form-control" placeholder="E-Mail" refs="email" onChange={this.handleChange.bind(this, "email")} value={this.state.fields["email"]} /> <span showerror={this.state.errors["email"] ? "yes" : ""} className="pl-3" style={{ color: "red", display: "none" }}>{this.state.errors["email"]}</span> </div> <div className="form-group pb-2"> <input borderColor={this.state.errors["password"] ? "red" : ""} type="password" className="form-control" placeholder="Password" refs="password" onChange={this.handleChange.bind(this, "password")} value={this.state.fields["password"]} /> <span showerror={this.state.errors["password"] ? "yes" : ""} className="pl-3" style={{ color: "red", display: "none" }}>{this.state.errors["password"]}</span> </div> <div className="form-group pb-4"> <input borderColor={this.state.errors["cpassword"] ? "red" : ""} type="password" className="form-control" placeholder="Confirm Password" refs="cpassword" onChange={this.handleChange.bind(this, "cpassword")} value={this.state.fields["cpassword"]} /> <span showerror={this.state.errors["cpassword"] ? "yes" : ""} className="pl-3" style={{ color: "red", display: "none" }}>{this.state.errors["cpassword"]}</span> </div> <div className="form-group"> <button className="btn btn-primary button_contenkt mb-3">Continue</button> </div> </form> </div> </div> </div> </div> ); } } export default App;
4. Now friends, we need to below code into our reacttemplate/src/App.css file for some custom styling:
span[showerror="yes"]{ display: block!important; } input[bordercolor="red"] { border-color:red; }
Now we are done friends. If you have any kind of query or suggestion or any requirement then feel free to comment below.
Note: Friends, I just tell the basic setup and things, you can change the code according to your requirements.
I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad.
Jassa
Thanks