Hello friends, welcome back to my blog. Today in this blog post, I am going to show you, Reactjs Bootstrap 5 Ecommerce Testing Project – Part 3 Checkout Page.
Guy’s before starting this post please check part 1 and part 2 for basics setup.
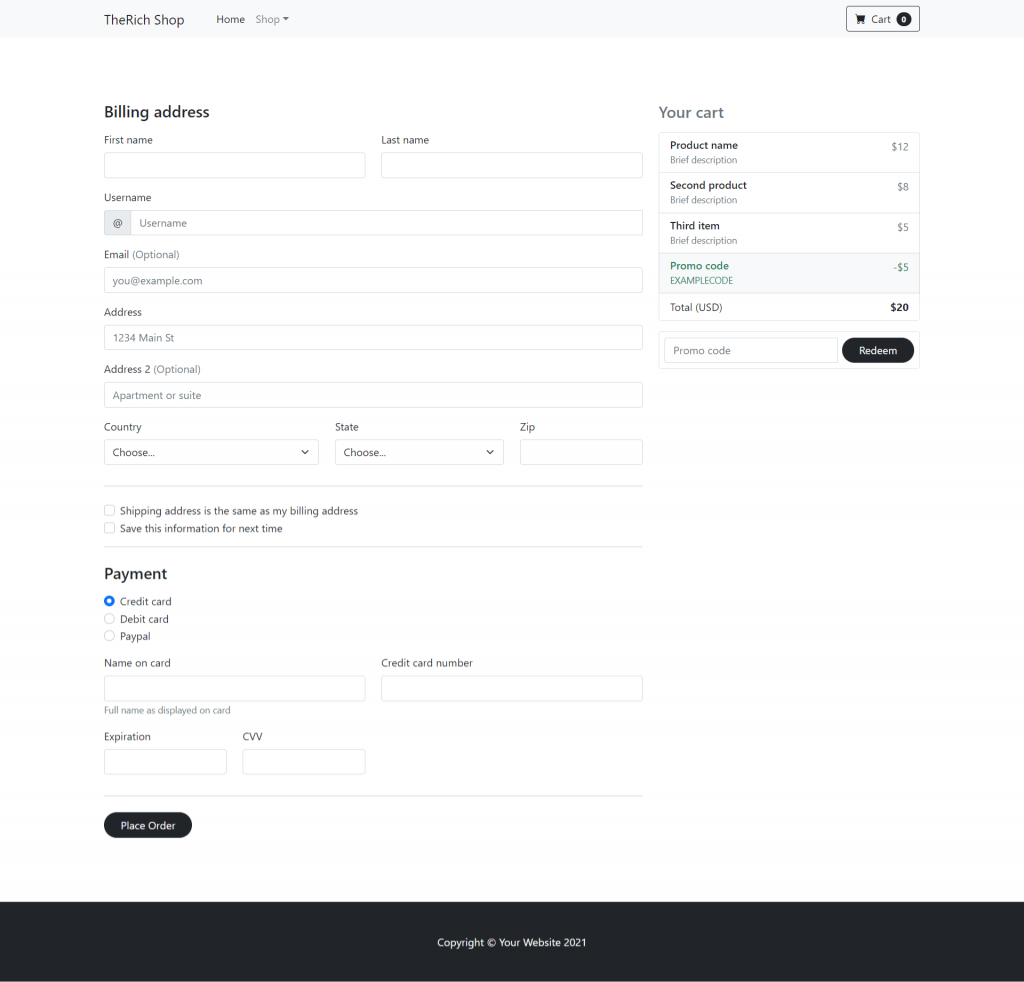
For reactjs and bootstrap5 new comers, please check the below links:
Friends now I proceed onwards and here is the working code snippet and please use this carefully to avoid the mistakes:
1. Now guy’s we will create `Checkout.js` file inside src/views folder and add below code inside it:
import React from 'react'; class checkout extends React.Component { render() { return ( <div className="checkout"> <section class="py-5"> <div class="container px-4 px-lg-5 my-5"> <div class="row"> <div class="col-md-4 order-md-2 mb-4"> <h4 class="d-flex justify-content-between align-items-center mb-3"> <span class="text-muted">Your cart</span> <span class="badge badge-secondary badge-pill">3</span> </h4> <ul class="list-group mb-3"> <li class="list-group-item d-flex justify-content-between lh-condensed"> <div> <h6 class="my-0">Product name</h6> <small class="text-muted">Brief description</small> </div> <span class="text-muted">$12</span> </li> <li class="list-group-item d-flex justify-content-between lh-condensed"> <div> <h6 class="my-0">Second product</h6> <small class="text-muted">Brief description</small> </div> <span class="text-muted">$8</span> </li> <li class="list-group-item d-flex justify-content-between lh-condensed"> <div> <h6 class="my-0">Third item</h6> <small class="text-muted">Brief description</small> </div> <span class="text-muted">$5</span> </li> <li class="list-group-item d-flex justify-content-between bg-light"> <div class="text-success"> <h6 class="my-0">Promo code</h6> <small>EXAMPLECODE</small> </div> <span class="text-success">-$5</span> </li> <li class="list-group-item d-flex justify-content-between"> <span>Total (USD)</span> <strong>$20</strong> </li> </ul> <form class="card p-2"> <div class="input-group"> <input type="text" class="form-control me-2" placeholder="Promo code"/> <div class="input-group-append"> <button type="button" class="btn btn-dark px-4 rounded-pill">Redeem</button> </div> </div> </form> </div> <div class="col-md-8 order-md-1"> <h4 class="mb-3">Billing address</h4> <form class="needs-validation" novalidate> <div class="row"> <div class="col-md-6 mb-3"> <label for="firstName" class="form-label">First name</label> <input type="text" class="form-control" id="firstName" placeholder="" value="" required /> <div class="invalid-feedback"> Valid first name is required. </div> </div> <div class="col-md-6 mb-3"> <label for="lastName" class="form-label">Last name</label> <input type="text" class="form-control" id="lastName" placeholder="" value="" required /> <div class="invalid-feedback"> Valid last name is required. </div> </div> </div> <div class="mb-3"> <label for="username" class="form-label">Username</label> <div class="input-group"> <div class="input-group-prepend"> <span class="input-group-text">@</span> </div> <input type="text" class="form-control" id="username" placeholder="Username" required /> <div class="invalid-feedback"> Your username is required. </div> </div> </div> <div class="mb-3"> <label for="email" class="form-label">Email <span class="text-muted">(Optional)</span></label> <input type="email" class="form-control" id="email" placeholder="you@example.com" /> <div class="invalid-feedback"> Please enter a valid email address for shipping updates. </div> </div> <div class="mb-3"> <label for="address" class="form-label">Address</label> <input type="text" class="form-control" id="address" placeholder="1234 Main St" required /> <div class="invalid-feedback"> Please enter your shipping address. </div> </div> <div class="mb-3"> <label for="address2" class="form-label">Address 2 <span class="text-muted">(Optional)</span></label> <input type="text" class="form-control" id="address2" placeholder="Apartment or suite" /> </div> <div class="row"> <div class="col-md-5 mb-3"> <label for="country" class="form-label">Country</label> <select class="form-select d-block w-100" id="country" required> <option value="">Choose...</option> <option>United States</option> </select> <div class="invalid-feedback"> Please select a valid country. </div> </div> <div class="col-md-4 mb-3"> <label for="state" class="form-label">State</label> <select class="form-select d-block w-100" id="state" required> <option value="">Choose...</option> <option>California</option> </select> <div class="invalid-feedback"> Please provide a valid state. </div> </div> <div class="col-md-3 mb-3"> <label for="zip" class="form-label">Zip</label> <input type="text" class="form-control" id="zip" placeholder="" required /> <div class="invalid-feedback"> Zip code required. </div> </div> </div> <hr class="mb-4" /> <div class="form-check"> <input type="checkbox" class="form-check-input" id="same-address" /> <label class="form-check-label" for="same-address">Shipping address is the same as my billing address</label> </div> <div class="form-check"> <input type="checkbox" class="form-check-input" id="save-info" /> <label class="form-check-label" for="save-info">Save this information for next time</label> </div> <hr class="mb-4" /> <h4 class="mb-3">Payment</h4> <div class="d-block my-3"> <div class="form-check"> <input id="credit" name="paymentMethod" type="radio" class="form-check-input" checked required /> <label class="form-check-label" for="credit">Credit card</label> </div> <div class="form-check"> <input id="debit" name="paymentMethod" type="radio" class="form-check-input" required /> <label class="form-check-label" for="debit">Debit card</label> </div> <div class="form-check"> <input id="paypal" name="paymentMethod" type="radio" class="form-check-input" required /> <label class="form-check-label" for="paypal">Paypal</label> </div> </div> <div class="row"> <div class="col-md-6 mb-3"> <label for="cc-name" class="form-label">Name on card</label> <input type="text" class="form-control" id="cc-name" placeholder="" required /> <small class="text-muted">Full name as displayed on card</small> <div class="invalid-feedback"> Name on card is required </div> </div> <div class="col-md-6 mb-3"> <label for="cc-number" class="form-label">Credit card number</label> <input type="text" class="form-control" id="cc-number" placeholder="" required /> <div class="invalid-feedback"> Credit card number is required </div> </div> </div> <div class="row"> <div class="col-md-3 mb-3"> <label for="cc-expiration" class="form-label">Expiration</label> <input type="text" class="form-control" id="cc-expiration" placeholder="" required /> <div class="invalid-feedback"> Expiration date required </div> </div> <div class="col-md-3 mb-3"> <label for="cc-expiration" class="form-label">CVV</label> <input type="text" class="form-control" id="cc-cvv" placeholder="" required /> <div class="invalid-feedback"> Security code required </div> </div> </div> <hr class="mb-4" /> <button class="btn btn-dark px-4 rounded-pill" type="button">Place Order</button> </form> </div> </div> </div> </section> </div> ) } } export default checkout;
2. Guy’s for the main output, we need to add below code into our src/App.js file or if you have fresh setup then you can replace src/App.js file code with below code:
import 'bootstrap/dist/css/bootstrap.min.css'; import 'bootstrap/dist/js/bootstrap.min.js'; import './App.css'; import { BrowserRouter as Router, Route, Link } from 'react-router-dom' import Header from './layouts/Header'; //Include Heder import Footer from './layouts/Footer'; //Include Footer import Home from './views/Home'; //Iclude Home import Single from './views/Single'; //Include Single import Cart from './views/Cart'; //Include Cart import Checkout from './views/Checkout'; //Include Checkout function App() { return ( <div className="App"> <Router> <Header></Header> <Route exact path='/' component={Home} /> <Route exact path='/home' component={Home} /> <Route exact path='/singleitem' component={Single} /> <Route exact path='/cart' component={Cart} /> <Route exact path='/checkout' component={Checkout} /> </Router> <Footer></Footer> </div> ); } export default App;
3. Now guy’s we need to below code inside src/views/Cart.js file for checkout page linking(check highlighted line number):
import React from 'react'; import { Link } from 'react-router-dom'; class Cart extends React.Component { render() { return ( <div className="single"> <section class="py-5"> <div class="container px-4 px-lg-5 my-5"> <div class="row"> <div class="col-lg-12 p-5 bg-white rounded shadow-sm mb-5"> <div class="table-responsive"> <table class="table"> <thead> <tr> <th scope="col" class="border-0 bg-light"> <div class="p-2 px-3 text-uppercase">Product</div> </th> <th scope="col" class="border-0 bg-light"> <div class="py-2 text-uppercase">Price</div> </th> <th scope="col" class="border-0 bg-light"> <div class="py-2 text-uppercase">Quantity</div> </th> <th scope="col" class="border-0 bg-light"> <div class="py-2 text-uppercase">Remove</div> </th> </tr> </thead> <tbody> <tr> <th scope="row" class="border-0"> <div class="p-2"> <img src="https://therichpost.com/wp-content/uploads/2021/05/dummyimage400x300.jpg" alt="" width="70" class="img-fluid rounded shadow-sm" /> <div class="ms-3 d-inline-block align-middle"> <h5 class="mb-0"> <a href="#" class="text-dark d-inline-block align-middle">Product 1</a></h5> </div> </div> </th> <td class="border-0 align-middle"><strong>$79.00</strong></td> <td class="border-0 align-middle"><strong>3</strong></td> <td class="border-0 align-middle"><a href="#" class="text-dark"><i class="bi bi-trash"></i></a></td> </tr> <tr> <th scope="row"> <div class="p-2"> <img src="https://therichpost.com/wp-content/uploads/2021/05/dummyimage400x300.jpg" alt="" width="70" class="img-fluid rounded shadow-sm" /> <div class="ms-3 d-inline-block align-middle"> <h5 class="mb-0"><a href="#" class="text-dark d-inline-block">Product 2</a></h5> </div> </div> </th> <td class="align-middle"><strong>$79.00</strong></td> <td class="align-middle"><strong>3</strong></td> <td class="align-middle"><a href="#" class="text-dark"><i class="bi bi-trash"></i></a> </td> </tr> <tr> <th scope="row"> <div class="p-2"> <img src="https://therichpost.com/wp-content/uploads/2021/05/dummyimage400x300.jpg" alt="" width="70" class="img-fluid rounded shadow-sm" /> <div class="ms-3 d-inline-block align-middle"> <h5 class="mb-0"> <a href="#" class="text-dark d-inline-block">Product 3</a></h5> </div> </div> </th> <td class="align-middle"><strong>$79.00</strong></td> <td class="align-middle"><strong>3</strong></td> <td class="align-middle"><a href="#" class="text-dark"><i class="bi bi-trash"></i></a> </td> </tr> </tbody> </table> </div> </div> </div> <div class="row py-5 p-4 bg-white rounded shadow-sm"> <div class="col-lg-6"> <div class="bg-light rounded-pill px-4 py-3 text-uppercase fw-bold">Coupon code</div> <div class="p-4"> <p class="mb-4"><em>If you have a coupon code, please enter it in the box below</em></p> <div class="input-group mb-4 border rounded-pill p-2"> <input type="text" placeholder="Apply coupon" aria-describedby="button-addon3" class="form-control border-0" /> <div class="input-group-append border-0"> <button id="button-addon3" type="button" class="btn btn-dark px-4 rounded-pill"><i class="fa fa-gift mr-2"></i>Apply coupon</button> </div> </div> </div> <div class="bg-light rounded-pill px-4 py-3 text-uppercase fw-bold">Instructions for seller</div> <div class="p-4"> <p class="mb-4"><em>If you have some information for the seller you can leave them in the box below</em></p> <textarea name="" cols="30" rows="2" class="form-control"></textarea> </div> </div> <div class="col-lg-6"> <div class="bg-light rounded-pill px-4 py-3 text-uppercase fw-bold">Order summary </div> <div class="p-4"> <p class="mb-4"><em>Shipping and additional costs are calculated based on values you have entered.</em></p> <ul class="list-unstyled mb-4"> <li class="d-flex justify-content-between py-3 border-bottom"><strong class="text-muted">Order Subtotal </strong><strong>$390.00</strong></li> <li class="d-flex justify-content-between py-3 border-bottom"><strong class="text-muted">Shipping and handling</strong><strong>$10.00</strong></li> <li class="d-flex justify-content-between py-3 border-bottom"><strong class="text-muted">Tax</strong><strong>$0.00</strong></li> <li class="d-flex justify-content-between py-3 border-bottom"><strong class="text-muted">Total</strong> <h5 class="fw-bold">$400.00</h5> </li> </ul><Link class="btn btn-dark rounded-pill py-2 d-md-block" to={'/checkout'}>Procceed to checkout</Link> </div> </div> </div> </div> </section> </div> ) } } export default Cart;
Now we are done friends and in next part we will do thank you page. If you have any kind of query or suggestion or any requirement then feel free to comment below.
Note: Friends, I just tell the basic setup and things, you can change the code according to your requirements.
I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad.
Jassa
Thanks
Recent Comments