Hello to all, welcome to therichpost.com. In this post, I will tell you, Laravel 7 Custom Access Token API Authentication.
Post Working:
In this post, I am creating custom access token during auth login and auth registration. I am doing this in Laravel 7.
Here is the working image from Passport:
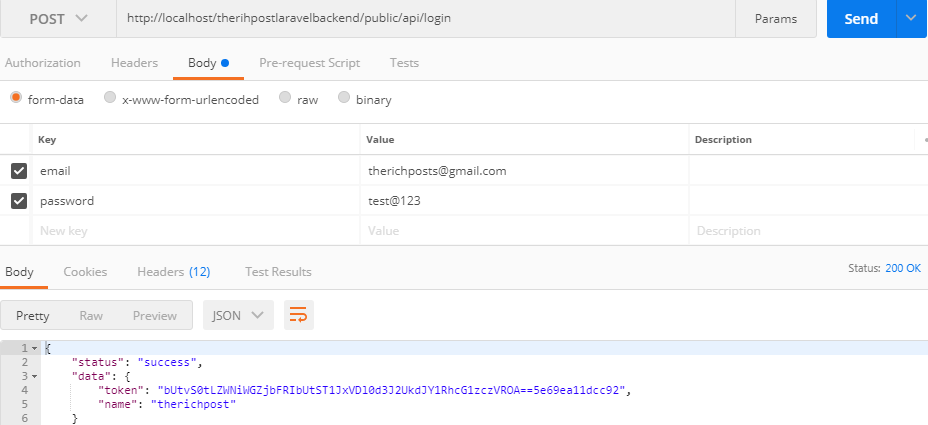
- Here is the link from where you can get the laravel 7 setup and Laravel 7 auth:
Laravel 7 setup and Laravel 7 Auth:
2. We need to create our API’S Routes with below code in routes/api.php:
Route::post('login', 'API\AuthController@login'); Route::post('register', 'API\AuthController@register');
3. We need to create API Folder Into our app\Http\Controllers:
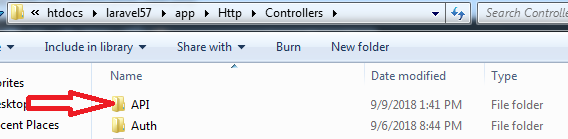
4. In app\Http\Controllers\API Folder, we need to create new file named AuthController.php file and add below code into this file:
<?php namespace App\Http\Controllers\API; use Illuminate\Http\Request; use App\Http\Controllers\Controller; use Illuminate\Support\Facades\Auth; use App\User; use Validator; use Illuminate\Support\Str; class AuthController extends Controller { /** * Login API * * @return \Illuminate\Http\Response */ private $apiToken; public function __construct() { $this->apiToken = uniqid(base64_encode(Str::random(40))); } public function login(Request $request){ if(Auth::attempt(['email' => $request->email, 'password' => $request->password])){ $user = Auth::user(); $success['token'] = $this->apiToken; $success['name'] = $user->name; return response()->json([ 'status' => 'success', 'data' => $success ]); } else { return response()->json([ 'status' => 'error', 'data' => 'Unauthorized Access' ]); } } /** * Register API * * @return \Illuminate\Http\Response */ public function register(Request $request) { $validator = Validator::make($request->all(), [ 'name' => 'required', 'email' => 'required|email', 'password' => 'required', 'c_password' => 'required|same:password', ]); if ($validator->fails()) { return response()->json(['error'=>$validator->errors()]); } $postArray = $request->all(); $postArray['password'] = bcrypt($postArray['password']); $user = User::create($postArray); $success['token'] = $this->apiToken; $success['name'] = $user->name; return response()->json([ 'status' => 'success', 'data' => $success, ]); } }
5. Here you can check the working login example in Postman:
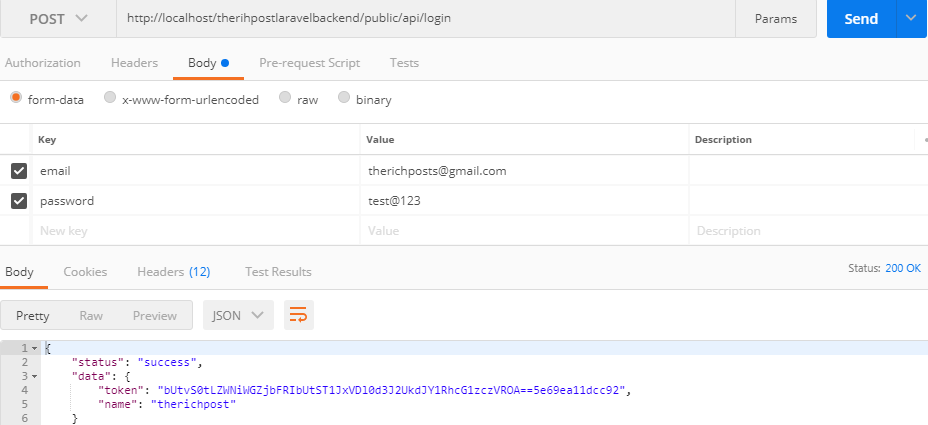
If you have any kind of query then please do comment below.
Jassa
Thank you.
hello when I test this tutorial in login it show to me
{
“status”: “error”,
“data”: “Unauthorized Access”
}
how can I fixed this problem ??
Because your login details are not correct.
no I added the same email and password in the register please help me
You need to debug your code during. Please check your login email and password again.
same problem 🙁