Hello to all, welcome to therichpost.com. In this post, I will continue with Angular 9 Laravel 7 Auth Login working tutorial Part 3.
Here you can check the first and second part of this post from where, you can get the information regarding:
1. How to install and run Angular 9: Part1
2. How to install Laravel 7 and Laravel 7 auth setup: Part2
Post Working:
In this post, I will continue with laravel and I am adding Laravel Passport module because During API connection with Laravel and Angular, we will also need security with proper authentication and for this I am using laravel Passport and it will give us access token which will help us to check login user authentication.
In this post, I will use Laravel Passport Package to create api auth and here is the full and easy process:
1. Very first, we need to install Laravel 7 Passport Package by run below command into your terminal:
composer require laravel/passport
2. After run above command, you need to add below code into your config/app.php file:
'providers' => [ .... Laravel\Passport\PassportServiceProvider::class, .... ],
3. We need to run migration command to add Passport package table in our database:
php artisan migrate
4. After successfully run above command, you can see below tables in your database:
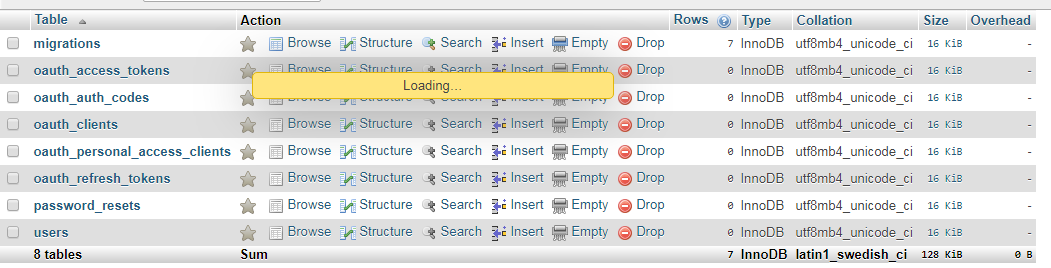
5. Next, we need to install passport with run below command into your terminal:
This will create security token keys:
php artisan passport:install
6. After run above command, we need to update app/User.php file with below code:
<?php ... use Laravel\Passport\HasApiTokens; class User extends Authenticatable { ... use HasApiTokens, Notifiable; ... }
7. After, update your app/Providers/AuthServiceProvider.php file with below code:
<?php ... use Laravel\Passport\Passport; ... class AppServiceProvider extends ServiceProvider { ... public function boot() { Schema::defaultStringLength(191); Passport::routes(); } ... }
8. After it, above your config/auth.php with below code:
return [ ..... 'guards' => [ 'web' => [ 'driver' => 'session', 'provider' => 'users', ], 'api' => [ 'driver' => 'passport', 'provider' => 'users', ], ], ..... ]
9. Finally, we need to create our API’S Routes with below code in routes/api.php:
<?php use Illuminate\Http\Request; /* |-------------------------------------------------------------------------- | API Routes |-------------------------------------------------------------------------- | | Here is where you can register API routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | is assigned the "api" middleware group. Enjoy building your API! | */ Route::post('login', 'API\AuthController@login'); Route::post('register', 'API\AuthController@register');
10. We need to create API Folder Into our app\Http\Controllers:
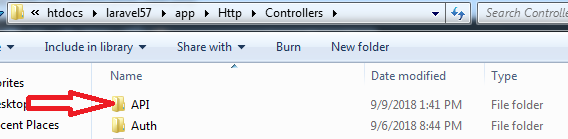
11. In app\Http\Controllers\API Folder, we need to create new file named AuthController.php file and add below code into this file:
<?php namespace App\Http\Controllers\API; use Illuminate\Http\Request; use App\Http\Controllers\Controller; use Illuminate\Support\Facades\Auth; use App\User; use Validator; class AuthController extends Controller { /** * Login API * * @return \Illuminate\Http\Response */ public function login(Request $request){ if(Auth::attempt(['email' => $request->email, 'password' => $request->password])){ $user = Auth::user(); $success['token'] = $user->createToken('LaraPassport')->accessToken; return response()->json([ 'status' => 'success', 'data' => $success ]); } else { return response()->json([ 'status' => 'error', 'data' => 'Unauthorized Access' ]); } } /** * Register API * * @return \Illuminate\Http\Response */ public function register(Request $request) { $validator = Validator::make($request->all(), [ 'name' => 'required', 'email' => 'required|email', 'password' => 'required', 'c_password' => 'required|same:password', ]); if ($validator->fails()) { return response()->json(['error'=>$validator->errors()]); } $postArray = $request->all(); $postArray['password'] = bcrypt($postArray['password']); $user = User::create($postArray); $success['token'] = $user->createToken('LaraPassport')->accessToken; $success['name'] = $user->name; return response()->json([ 'status' => 'success', 'data' => $success, ]); } }
- After all this, we need to test our’s api’s by downloading the postman software:
https://www.getpostman.com/apps
16. Here I tested Register Api by enter name, email, password, and c_password. c_password details:
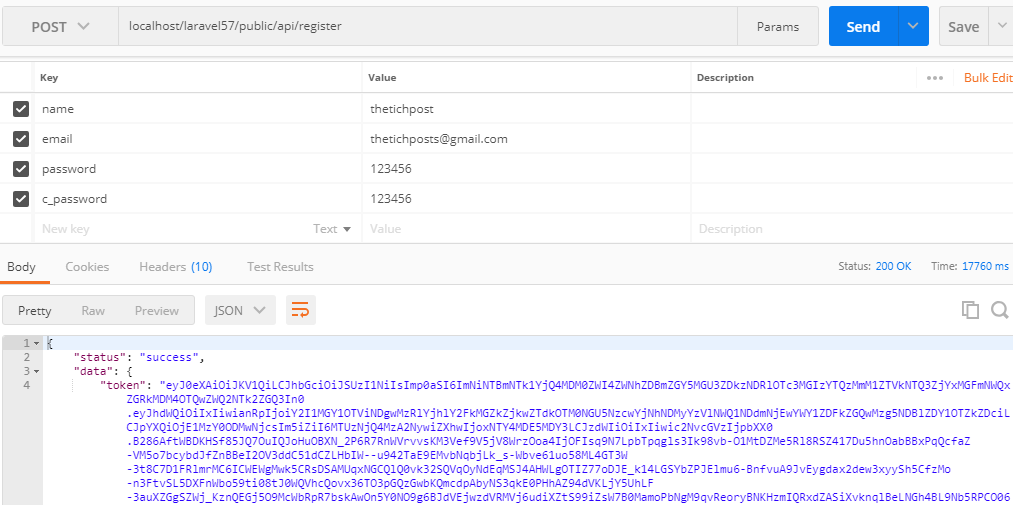
17. Here, I tested Login Api:
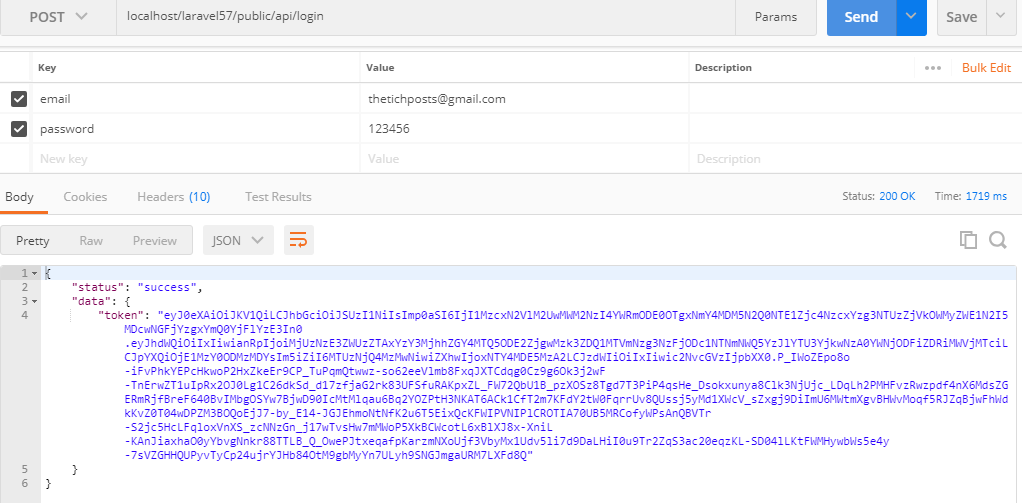
Now, we are done with Rest Api Authentication in Laravel 7 Using Passport Package . If you have any query related to this post, then do comment below or ask questions.
After this, I will also tell you, how we can create custom token without passport.
Jassa
Thank you
hello, i need help 🙂 when i tested Register in postman , i have
“error”: {
“password-C”: [
“The password- c field is required.”
]
because you are sending details with empty password.
hello , i have this error
BadMethodCallException: Method Illuminate\Auth\RequestGuard::attempt does not exist
Seems, you using some other methods?
I needed to add the following line to app/Providers/AuthServiceProvider.php
use Illuminate\Support\Facades\Schema;
Okay fine
please complete the tutorial with part 4 where you join angular login / register pages with laravel backend done in part3 ? And please also add an angular example page with limited access to logged users
Okay sure and I will come very soon. Thanks
nice, thkz for the direct to the point explanation… this saves me a lot of time…
Thank you and welcome.