Implementing JSON Web Tokens (JWT) in an Angular standalone application involves several steps. Angular 17, as a part of the evolving framework, supports standalone components, routes, and modules, simplifying application structures. Here’s how you can implement JWT for handling authentication in an Angular 17 standalone app.
Angular 17 came and Bootstrap 5 also. If you are new then you must check below two links:
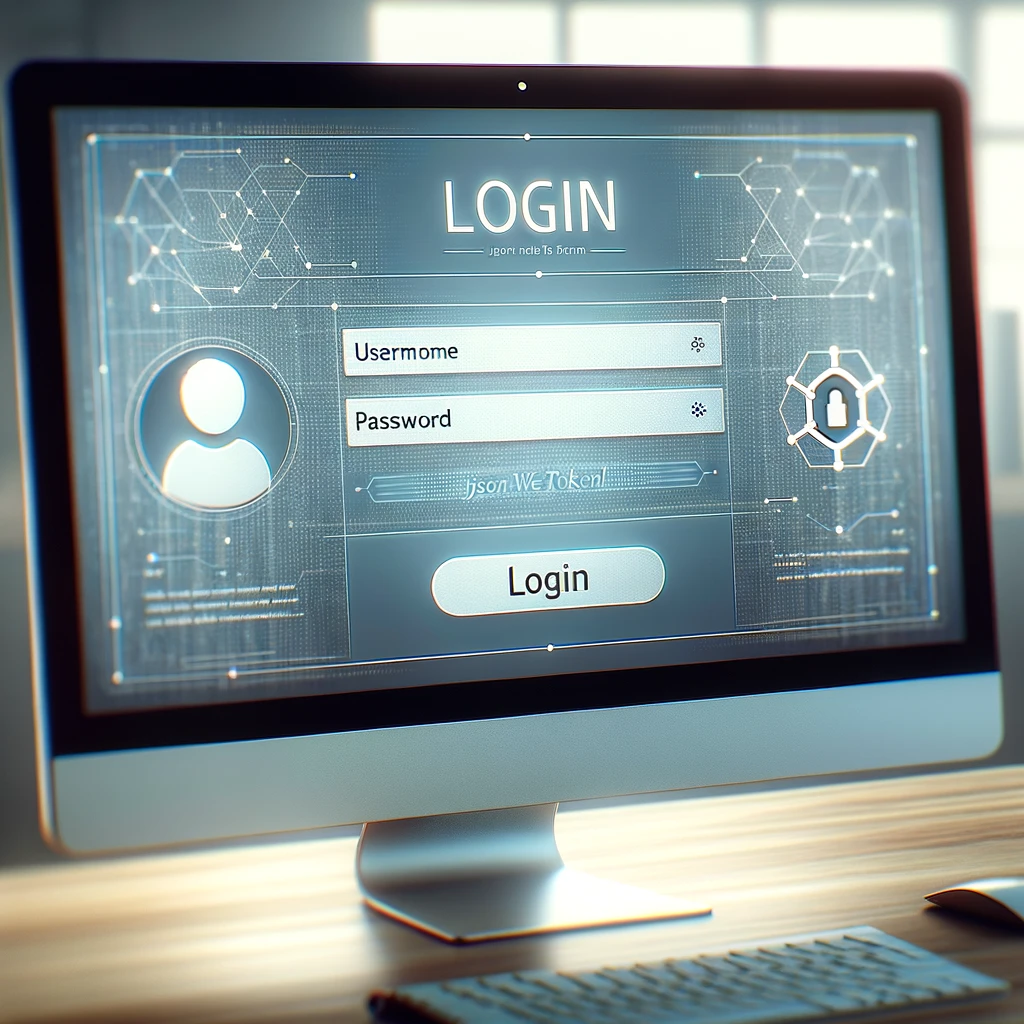
Step 1: Set Up Your Angular Project
First, ensure you have the latest Angular CLI installed. You can install or update it using the following command:
npm install -g @angular/cli
Create a new Angular project:
ng new jwt-angular-app --standalone cd jwt-angular-app
Step 2: Install Necessary Packages
You will need a few packages, such as @auth0/angular-jwt
for handling JWTs easily:
npm install @auth0/angular-jwt
Step 3: Create Authentication Service
Create an authentication service to handle JWT storage, retrieval, and the authentication state.
// src/app/auth.service.ts import { Injectable } from '@angular/core'; import { JwtHelperService } from '@auth0/angular-jwt'; @Injectable({ providedIn: 'root' }) export class AuthService { private jwtHelper = new JwtHelperService(); constructor() {} public setToken(token: string): void { localStorage.setItem('access_token', token); } public getToken(): string | null { return localStorage.getItem('access_token'); } public isAuthenticated(): boolean { const token = this.getToken(); return token ? !this.jwtHelper.isTokenExpired(token) : false; } public logout(): void { localStorage.removeItem('access_token'); } }
Step 4: Protect Routes with Auth Guard
Create a guard to protect routes that require authentication.
// src/app/auth.guard.ts import { Injectable } from '@angular/core'; import { CanActivate, Router } from '@angular/router'; import { AuthService } from './auth.service'; @Injectable({ providedIn: 'root' }) export class AuthGuard implements CanActivate { constructor(private authService: AuthService, private router: Router) {} canActivate(): boolean { if (!this.authService.isAuthenticated()) { this.router.navigate(['/login']); return false; } return true; } }
Step 5: Configure Routing
Set up routing to use the Auth Guard.
// src/app/app.routes.ts import { RouterModule } from '@angular/router'; import { HomeComponent } from './home.component'; import { LoginComponent } from './login.component'; import { AuthGuard } from './auth.guard'; export const AppRoutes = RouterModule.forRoot([ { path: '', component: HomeComponent, canActivate: [AuthGuard] }, { path: 'login', component: LoginComponent } ], { standalone: true });
Step 6: Implement Login and Home Components
Create components for login
and home, handling the interaction with the AuthService for authentication.
Create Login Component
// src/app/login.component.ts import { Component } from '@angular/core'; import { AuthService } from './auth.service'; import { Router } from '@angular/router'; @Component({ selector: 'app-login', template: ` <div> <h2>Login</h2> <input type="text" [(ngModel)]="username" placeholder="Username"> <input type="password" [(ngModel)]="password" placeholder="Password"> <button (click)="login()">Login</button> </div> `, standalone: true, imports: [] }) export class LoginComponent { username: string = ''; password: string = ''; constructor(private authService: AuthService, private router: Router) {} login(): void { // Simulate login and token retrieval const fakeToken = 'eyJ...'; // Normally you'd get this from a backend after verifying credentials this.authService.setToken(fakeToken); this.router.navigate(['/']); } }
Create Home Component
// src/app/home.component.ts import { Component } from '@angular/core'; import { AuthService } from './auth.service'; @Component({ selector: 'app-home', template: ` <div> <h1>Home</h1> <p>Welcome to the protected area!</p> <button (click)="logout()">Logout</button> </div> `, standalone: true, imports: [] }) export class HomeComponent { constructor(private authService: AuthService) {} logout(): void { this.authService.logout(); window.location.reload(); } }
Step 7: Add Components to AppModule and Update main.ts
Since Angular 17 supports standalone components, routes, and services, you might need to adjust how you bootstrap these in your main.ts
.
// src/main.ts import { enableProdMode } from '@angular/core'; import { bootstrapApplication } from '@angular/platform-browser'; import { AppComponent } from './app/app.component'; import { environment } from './environments/environment'; import { AppRoutes } from './app/app.routes'; if (environment.production) { enableProdMode(); } bootstrapApplication(AppComponent, { providers: [AppRoutes] }).catch(err => console.error(err));
Step 8: Run Your Angular Application
Now, run your Angular application to see everything in action:
ng serve
Navigate to http://localhost:4200
to test the authentication flow. The example above uses hard-coded token values and simplistic navigation logic, which you should replace with actual authentication logic tied to your backend system. This could involve sending the username and password to a backend server, receiving a JWT upon successful authentication, and storing it for subsequent requests to protected resources.
Jassa
Thanks