In Angular, you can respond to changes in data (like a signal value) using various methods, depending on how your data is structured and how you want to track its changes. Angular provides a robust framework for monitoring and reacting to data changes through mechanisms like component lifecycle hooks, template-driven events, and reactive programming with RxJS observables.
If you’re tracking a simple signal, such as a value that changes over time and you want to execute a function whenever this value changes, you can use Angular’s component lifecycle hooks or RxJS observables. Here’s how you can do it with each method:
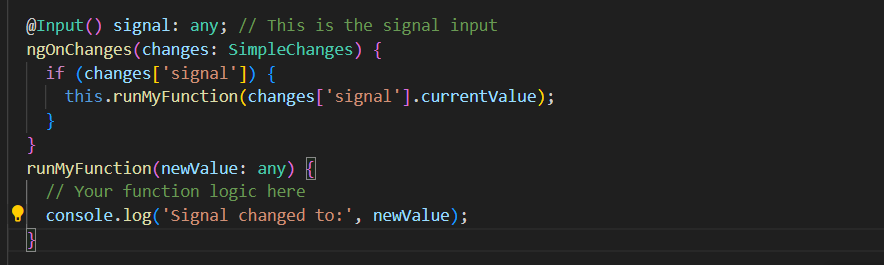
1. Using Component Lifecycle Hooks
If your signal is a component input, you can use the OnChanges
lifecycle hook to react to changes in the input properties:
import { Component, Input, OnChanges, SimpleChanges } from '@angular/core'; @Component({ selector: 'my-component', template: `<!-- component template here -->`, }) export class MyComponent implements OnChanges { @Input() signal: any; // This is the signal input ngOnChanges(changes: SimpleChanges) { if (changes['signal']) { this.runMyFunction(changes['signal'].currentValue); } } runMyFunction(newValue: any) { // Your function logic here console.log('Signal changed to:', newValue); } }
2. Using RxJS Observables
If your signal value is part of a service or it’s a stream of values, you can use RxJS Observables to subscribe to these changes:
import { Component, OnDestroy } from '@angular/core'; import { Subscription } from 'rxjs'; import { SignalService } from './signal.service'; @Component({ selector: 'my-observable-component', template: `<!-- component template here -->`, }) export class MyObservableComponent implements OnDestroy { private subscription: Subscription; constructor(private signalService: SignalService) { this.subscription = this.signalService.getSignal().subscribe({ next: (signal) => { this.runMyFunction(signal); }, error: (error) => console.error('Something went wrong: ', error), }); } runMyFunction(signal: any) { // Your function logic here console.log('Signal received:', signal); } ngOnDestroy() { // Clean up the subscription this.subscription.unsubscribe(); } }
In this example, SignalService
should be an Angular service that you create which returns an Observable from a method like getSignal()
. This Observable might be tied to a data source that emits values (like a WebSocket or event source).
Both approaches allow you to react to changes in signal values. The best method depends on your specific use case, such as whether the value changes are asynchronous, their source, and how they integrate into your application’s architecture.
jassa
Thanks
Recent Comments