To handle multiple instances of microfrontends in Angular 17, you can use a combination of tools and strategies to manage their integration smoothly. Microfrontends allow for a scalable architecture where different frontend teams can work independently on parts of a large application. Here’s how you can set this up in Angular:
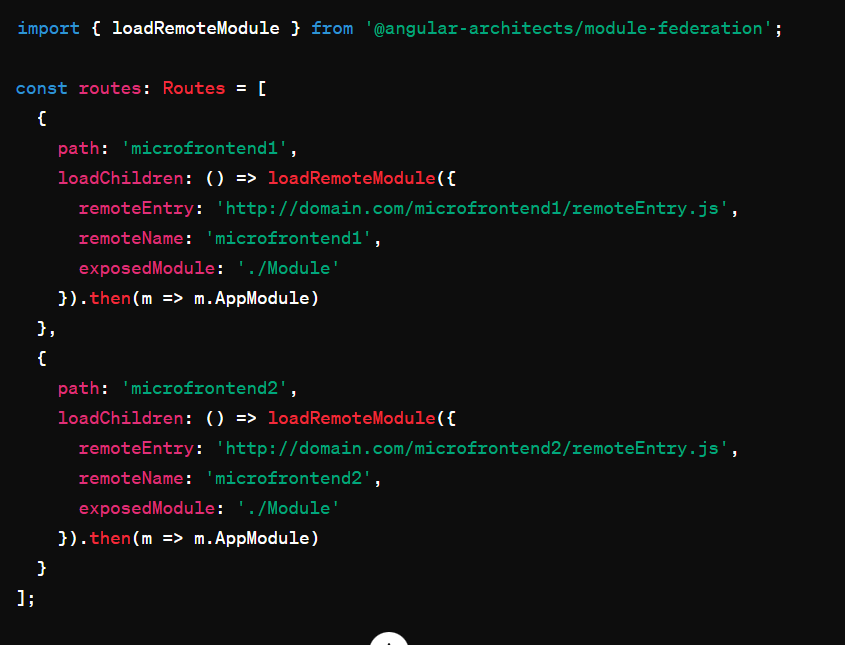
1. Module Federation
Angular 17 continues to support Module Federation, a Webpack feature that enables JavaScript applications to dynamically load code from other applications. This is particularly useful for microfrontends.
Setup:
- Host Configuration: In the Webpack configuration of the host application, you need to set up the
ModuleFederationPlugin
to define which microfrontends (remote entries) it can consume.
// webpack.config.js const ModuleFederationPlugin = require("webpack/lib/container/ModuleFederationPlugin"); module.exports = { plugins: [ new ModuleFederationPlugin({ name: 'host', remotes: { microfrontend1: 'microfrontend1@http://domain.com/microfrontend1/remoteEntry.js', microfrontend2: 'microfrontend2@http://domain.com/microfrontend2/remoteEntry.js', }, shared: { '@angular/core': { singleton: true, strictVersion: true }, '@angular/common': { singleton: true, strictVersion: true }, '@angular/router': { singleton: true, strictVersion: true }, // other shared dependencies }, }), ], };
- Microfrontend Configuration: Each microfrontend should have its own Webpack configuration using
ModuleFederationPlugin
to expose itself as a remote entry.
Usage:
- You can dynamically load a microfrontend in an Angular component using
loadRemoteModule()
from@angular-architects/module-federation
.
2. Routing Integration
Angular 17 came and Bootstrap 5 also. If you are new then you must check below two links:
To integrate multiple microfrontends seamlessly, use Angular’s Router. You can configure the router to load different microfrontends based on routes.
import { loadRemoteModule } from '@angular-architects/module-federation'; const routes: Routes = [ { path: 'microfrontend1', loadChildren: () => loadRemoteModule({ remoteEntry: 'http://domain.com/microfrontend1/remoteEntry.js', remoteName: 'microfrontend1', exposedModule: './Module' }).then(m => m.AppModule) }, { path: 'microfrontend2', loadChildren: () => loadRemoteModule({ remoteEntry: 'http://domain.com/microfrontend2/remoteEntry.js', remoteName: 'microfrontend2', exposedModule: './Module' }).then(m => m.AppModule) } ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { }
3. State Management
When dealing with multiple microfrontends, maintaining a consistent state across them can be challenging. Consider using a global state management solution like NgRx or a shared JavaScript context (like Redux) that all microfrontends can access.
4. Communication Between Microfrontends
For communication between microfrontends:
- Custom Events: Use the browser’s custom event system to emit and listen for events.
- RxJS: Share a BehaviorSubject or a Subject to emit and subscribe to data changes across different parts of the application.
Best Practices
- Isolation: Ensure each microfrontend is independent in terms of its dependencies and build process.
- Consistency: Use a design system to keep UI components consistent across different microfrontends.
- Version Control: Carefully manage versions of shared libraries to avoid conflicts.
By leveraging these strategies, you can effectively manage multiple microfrontends in Angular 17, allowing different teams to work autonomously while maintaining a cohesive application structure.
Jassa
Thanks
Hi
How can I deploy the microfrontend apps on one of the popular hosting site? eg. cloudflare, github pages, netlify aws s3 or vercel?
Hi, Here you can check:
https://therichpost.com/how-can-i-deploy-the-microfrontend-apps-on-one-of-the-popular-hosting-site/