Exporting a table to an XLSX file in a React application can be done using third-party libraries that handle Excel file creation and management. A popular choice for this is xlsx
from SheetJS, which allows for manipulation of Excel files. Here’s a basic guide on how to implement this functionality:
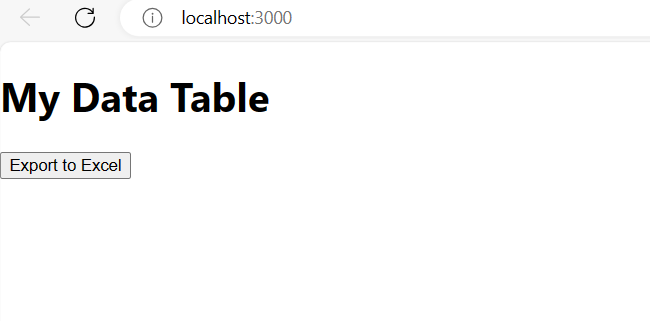
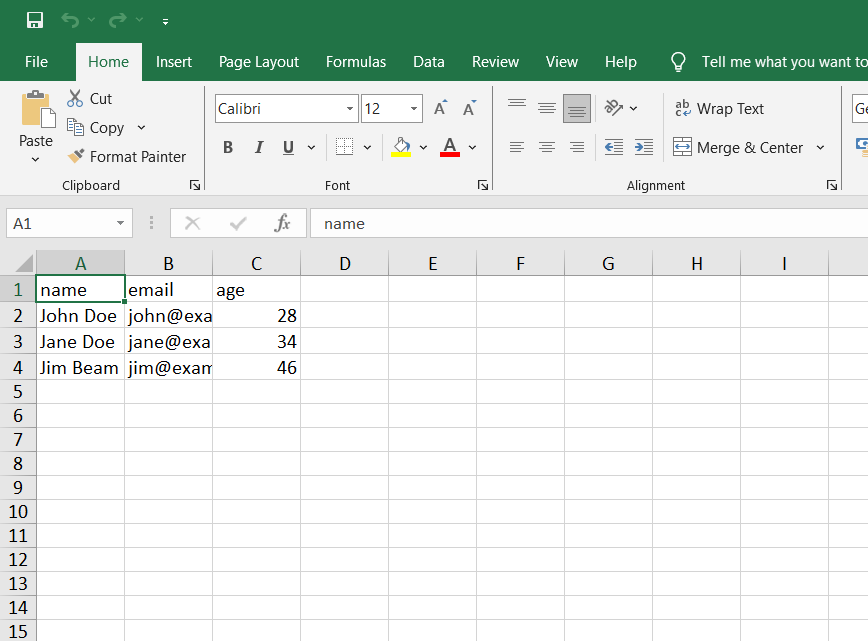
For reactjs new comers, please check the below link:
1. Install the Necessary Libraries
First, you’ll need to install xlsx
. You can add it to your React project via npm or yarn:
npm install xlsx
or
yarn add xlsx
2. Create a Component to Export the Table
Next, create a React component or function that triggers the export. Here’s a simple example where data is hardcoded, but in a real-world scenario, you would likely pass the data to the export function dynamically (e.g., via props or state).
Example Component:
import React from 'react'; import * as XLSX from 'xlsx'; function ExportToExcel() { const data = [ { name: "John Doe", email: "john@example.com", age: 28 }, { name: "Jane Doe", email: "jane@example.com", age: 34 }, { name: "Jim Beam", email: "jim@example.com", age: 46 } ]; const handleExport = () => { // Create a new workbook const wb = XLSX.utils.book_new(); // Convert data to worksheet const ws = XLSX.utils.json_to_sheet(data); // Append worksheet to workbook under the name "Sheet1" XLSX.utils.book_append_sheet(wb, ws, "Sheet1"); // Write workbook and trigger download XLSX.writeFile(wb, "Data.xlsx"); }; return ( <button onClick={handleExport}>Export to Excel</button> ); } export default ExportToExcel;
Explanation:
- Data Definition: The
data
array contains objects that represent the rows you want to export. Each object’s keys should match the desired column headers in the Excel file. - Workbook Creation: A new workbook is created using
XLSX.utils.book_new()
. - Data Conversion: The
data
array is converted into a worksheet usingXLSX.utils.json_to_sheet(data)
. - Appending Worksheet: The newly created worksheet is then appended to the workbook.
- Export:
XLSX.writeFile()
is used to generate the Excel file and prompt the user to save it locally.
3. Integrate the Export Component
Finally, use the ExportToExcel
component wherever you need the export functionality in your app:
import React from 'react'; import ExportToExcel from './ExportToExcel'; function App() { return ( <div> <h1>My Data Table</h1> <ExportToExcel /> </div> ); } export default App;
This simple example sets up the basics. Depending on your needs, you might want to extend this by dynamically generating the data from state, handling more complex data structures, or customizing the file’s formatting and styles using xlsx
options.
Jassa
Thanks
Recent Comments