Hello guys how are you? Welcome back to my blog. Today in this blog post we are Creating a React based real estate website template using react-bootstrap.
Full Features List
- Reactjs
- Based on Bootstrap 5.x
- React-Bootstrap
- Home + 4 Pages
- 100% Responsive
- Nice and Clean Design
- Easy to customize
- Flat, modern and clean design
- react-router-dom
- react-icons/fa
For react js new comers, please check the below links:
Guys here is the complete code snippet for Creating a React based real estate website template using react-bootstrap and please do carefully to avoid the mistakes:
1. Setup your React Project
First, set up your React project using Create React App and Add important Modules:
npx create-react-app real-estate-website cd real-estate-website npm install bootstrap npm install react-router-dom npm install react-bootstrap bootstrap npm install react-icons
2. Create the Project Structure
Create a basic project structure:
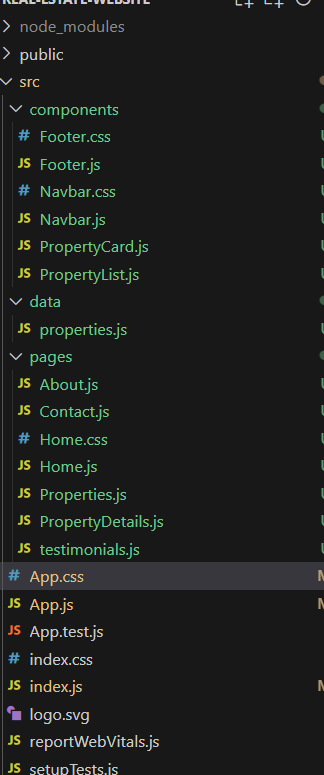
3. Create Components
Footer.css
.footer { background-color: #343a40; color: #f8f9fa; } .footer h5 { font-size: 1.25rem; margin-bottom: 1rem; } .footer p, .footer a { font-size: 1rem; color: #f8f9fa; text-decoration: none; } .footer a:hover { color: #adb5bd; } .footer .list-unstyled { padding-left: 0; } .footer .list-unstyled li { margin-bottom: 0.5rem; } .footer .d-flex a { color: #f8f9fa; transition: color 0.3s ease; } .footer .d-flex a:hover { color: #adb5bd; } .footer .text-center { margin-top: 2rem; }
Footer.js
import React from 'react'; import { Container, Row, Col, Form, Button } from 'react-bootstrap'; import { FaFacebookF, FaTwitter, FaInstagram } from 'react-icons/fa'; import './Footer.css'; // Import custom CSS const Footer = () => ( <footer className="footer bg-dark text-white mt-5 p-4"> <Container> <Row> <Col md={4} className="mb-4"> <h5>Contact Us</h5> <p>123 Real Estate St, City, Country</p> <p>Phone: +1 (234) 567-890</p> <p>Email: info@realestate.com</p> </Col> <Col md={4} className="mb-4"> <h5>Quick Links</h5> <ul className="list-unstyled"> <li><a href="#" className="text-white">Home</a></li> <li><a href="#" className="text-white">About</a></li> <li><a href="#" className="text-white">Properties</a></li> <li><a href="#" className="text-white">Contact</a></li> </ul> </Col> <Col md={4} className="mb-4"> <h5>Follow Us</h5> <div className="d-flex"> <a href="https://www.facebook.com" target="_blank" rel="noopener noreferrer" className="text-white me-3"> <FaFacebookF size={24} /> </a> <a href="https://www.twitter.com" target="_blank" rel="noopener noreferrer" className="text-white me-3"> <FaTwitter size={24} /> </a> <a href="https://www.instagram.com" target="_blank" rel="noopener noreferrer" className="text-white me-3"> <FaInstagram size={24} /> </a> </div> </Col> </Row> <Row> <Col md={12} className="text-center"> <p className="mb-0">© {new Date().getFullYear()} Real Estate. All rights reserved.</p> </Col> </Row> </Container> </footer> ); export default Footer;
Navbar.css
.custom-navbar { box-shadow: 0 4px 6px rgba(0, 0, 0, 0.1); } .custom-navbar .navbar-brand { font-size: 1.5rem; font-weight: bold; color: #f8f9fa; } .custom-navbar .nav-link { font-size: 1.1rem; margin-left: 15px; color: #f8f9fa !important; transition: color 0.3s ease; } .custom-navbar .nav-link:hover { color: #adb5bd !important; } .custom-navbar .search-form input { border-radius: 20px; } .custom-navbar .btn-outline-light { border-radius: 20px; } .custom-navbar .social-icons .nav-link { color: #f8f9fa !important; font-size: 1.2rem; margin-left: 10px; transition: color 0.3s ease; } .custom-navbar .social-icons .nav-link:hover { color: #adb5bd !important; }
Navbar.js
import React from 'react'; import { Navbar, Nav, Container, Form, FormControl, Button } from 'react-bootstrap'; import { Link } from 'react-router-dom'; import { FaFacebookF, FaTwitter, FaInstagram } from 'react-icons/fa'; import './Navbar.css'; // Import custom CSS const NavigationBar = () => ( <Navbar bg="dark" variant="dark" expand="lg" className="custom-navbar"> <Container> <Navbar.Brand as={Link} to="/">Real Estate</Navbar.Brand> <Navbar.Toggle aria-controls="basic-navbar-nav" /> <Navbar.Collapse id="basic-navbar-nav"> <Nav className="me-auto"> <Nav.Link as={Link} to="/">Home</Nav.Link> <Nav.Link as={Link} to="/about">About</Nav.Link> <Nav.Link as={Link} to="/properties">Properties</Nav.Link> <Nav.Link as={Link} to="/contact">Contact</Nav.Link> </Nav> <Form className="d-flex me-3 search-form"> <FormControl type="search" placeholder="Search" className="me-2" aria-label="Search" /> <Button variant="outline-light">Search</Button> </Form> <Nav className="social-icons"> <Nav.Link href="https://www.facebook.com" target="_blank"> <FaFacebookF /> </Nav.Link> <Nav.Link href="https://www.twitter.com" target="_blank"> <FaTwitter /> </Nav.Link> <Nav.Link href="https://www.instagram.com" target="_blank"> <FaInstagram /> </Nav.Link> </Nav> </Navbar.Collapse> </Container> </Navbar> ); export default NavigationBar;
PropertyCard.js
import React from 'react'; import { Card, Button } from 'react-bootstrap'; import { Link } from 'react-router-dom'; const PropertyCard = ({ property }) => ( <Card style={{ width: '18rem' }}> <Card.Img variant="top" src={property.image} /> <Card.Body> <Card.Title>{property.title}</Card.Title> <Card.Text> {property.description} </Card.Text> <Button as={Link} to={`/properties/${property.id}`} variant="primary"> More Details </Button> </Card.Body> </Card> ); export default PropertyCard;
PropertyList.js
import React from 'react'; import { Container, Row, Col } from 'react-bootstrap'; import PropertyCard from './PropertyCard'; import properties from '../data/properties'; const PropertyList = () => ( <Container> <Row> {properties.map(property => ( <Col key={property.id} sm={12} md={6} lg={4}> <PropertyCard property={property} /> </Col> ))} </Row> </Container> ); export default PropertyList;
4. Create Demo Product Data
const properties = [ { id: 1, title: 'Luxury Villa', description: 'A beautiful villa with sea view.', image: 'https://via.placeholder.com/300' }, { id: 2, title: 'Modern Apartment', description: 'A modern apartment in the city center.', image: 'https://via.placeholder.com/300' }, { id: 3, title: 'Cozy Cottage', description: 'A cozy cottage in the countryside.', image: 'https://via.placeholder.com/300' }, { id: 4, title: 'Spacious Townhouse', description: 'A spacious townhouse in a quiet neighborhood.', image: 'https://via.placeholder.com/300' }, { id: 5, title: 'Beachfront Condo', description: 'A beachfront condo with stunning views.', image: 'https://via.placeholder.com/300' } ]; export default properties;
5. Create Page Components
About.js
import React from 'react'; import { Container, Row, Col, Card, Carousel } from 'react-bootstrap'; const teamMembers = [ { name: 'John Doe', position: 'CEO', image: 'https://via.placeholder.com/150', bio: 'John has over 20 years of experience in the real estate industry and leads the company with a vision for excellence.' }, { name: 'Jane Smith', position: 'COO', image: 'https://via.placeholder.com/150', bio: 'Jane oversees all operational aspects of the business, ensuring everything runs smoothly and efficiently.' }, { name: 'Sam Wilson', position: 'CFO', image: 'https://via.placeholder.com/150', bio: 'Sam manages the financial actions of the company, focusing on profitability and growth.' } ]; const testimonials = [ { name: 'Emily Brown', feedback: 'The team at Real Estate were incredibly helpful and professional. They helped us find our dream home!', image: 'https://via.placeholder.com/150' }, { name: 'Michael Johnson', feedback: 'A wonderful experience from start to finish. Highly recommend their services!', image: 'https://via.placeholder.com/150' } ]; const About = () => ( <Container className="my-5"> <Row className="mb-4"> <Col> <h1>About Us</h1> <p> Welcome to Real Estate, your trusted partner in finding the perfect home. With over two decades of experience, we have been helping people find their dream homes with ease and confidence. </p> </Col> </Row> <Row className="mb-4"> <Col> <h2>Our History</h2> <p> Established in 2000, Real Estate has grown from a small local agency to a nationally recognized company. Our commitment to excellence and customer satisfaction has been the cornerstone of our success. </p> </Col> </Row> <Row className="mb-4"> <Col> <h2>Mission and Vision</h2> <p> <strong>Mission:</strong> To provide exceptional real estate services with integrity, professionalism, and respect for our clients and the community. </p> <p> <strong>Vision:</strong> To be the leading real estate company known for transforming lives through outstanding service and a dedication to excellence. </p> </Col> </Row> <Row className="mb-4"> <Col> <h2>Meet Our Team</h2> <Row> {teamMembers.map(member => ( <Col key={member.name} sm={12} md={4} className="mb-4"> <Card> <Card.Img variant="top" src={member.image} /> <Card.Body> <Card.Title>{member.name}</Card.Title> <Card.Subtitle className="mb-2 text-muted">{member.position}</Card.Subtitle> <Card.Text>{member.bio}</Card.Text> </Card.Body> </Card> </Col> ))} </Row> </Col> </Row> <Row className="mb-4"> <Col> <h2>Testimonials</h2> <Carousel> {testimonials.map((testimonial, index) => ( <Carousel.Item key={index}> <Card className="text-center"> <Card.Body> <Card.Img variant="top" src={testimonial.image} className="rounded-circle w-25 mb-3" /> <Card.Text className="blockquote mb-0"> "{testimonial.feedback}" </Card.Text> <footer className="blockquote-footer mt-2">{testimonial.name}</footer> </Card.Body> </Card> </Carousel.Item> ))} </Carousel> </Col> </Row> </Container> ); export default About;
Contact.js
import React, { useState } from 'react'; import { Container, Row, Col, Form, Button } from 'react-bootstrap'; const Contact = () => { const [formData, setFormData] = useState({ name: '', email: '', message: '' }); const handleChange = (e) => { const { name, value } = e.target; setFormData({ ...formData, [name]: value }); }; const handleSubmit = (e) => { e.preventDefault(); // Handle form submission (e.g., send data to a server) console.log('Form data submitted:', formData); // Reset form fields setFormData({ name: '', email: '', message: '' }); }; return ( <Container className="my-5"> <Row className="mb-4 text-center"> <Col> <h1>Contact Us</h1> <p>We would love to hear from you. Please fill out the form below to get in touch with us.</p> </Col> </Row> <Row className="mb-4"> <Col md={6}> <h2>Contact Details</h2> <p><strong>Address:</strong> 123 Real Estate St, City, Country</p> <p><strong>Phone:</strong> +1 (234) 567-890</p> <p><strong>Email:</strong> info@realestate.com</p> </Col> <Col md={6}> <h2>Contact Form</h2> <Form onSubmit={handleSubmit}> <Form.Group className="mb-3" controlId="formName"> <Form.Label>Name</Form.Label> <Form.Control type="text" placeholder="Enter your name" name="name" value={formData.name} onChange={handleChange} required /> </Form.Group> <Form.Group className="mb-3" controlId="formEmail"> <Form.Label>Email address</Form.Label> <Form.Control type="email" placeholder="Enter your email" name="email" value={formData.email} onChange={handleChange} required /> </Form.Group> <Form.Group className="mb-3" controlId="formMessage"> <Form.Label>Message</Form.Label> <Form.Control as="textarea" rows={3} placeholder="Enter your message" name="message" value={formData.message} onChange={handleChange} required /> </Form.Group> <Button variant="primary" type="submit"> Submit </Button> </Form> </Col> </Row> </Container> ); }; export default Contact;
Home.css
.hero-section { background: url('https://via.placeholder.com/1500x500') no-repeat center center; background-size: cover; color: white; padding: 100px 0; } .hero-section h1 { font-size: 3rem; font-weight: bold; } .hero-section p { font-size: 1.5rem; margin-bottom: 30px; } .property-card img { height: 200px; object-fit: cover; } .property-card { transition: transform 0.2s; } .property-card:hover { transform: scale(1.05); } .carousel-item img { max-height: 500px; object-fit: cover; } .blockquote { border-left: 5px solid #ddd; padding-left: 15px; margin-left: 0; margin-right: 0; font-size: 1.2em; } .blockquote-footer { font-size: 0.875em; color: #6c757d; }
Home.js
import React from 'react'; import { Container, Row, Col, Card, Button, Carousel } from 'react-bootstrap'; import { Link } from 'react-router-dom'; import properties from '../data/properties'; import testimonials from './testimonials'; import './Home.css'; // Import custom CSS const Home = () => { const featuredProperties = properties.slice(0, 3); // Select first 3 properties as featured return ( <div> <div className="hero-section"> <Container className="text-center text-white"> <h1>Welcome to Real Estate</h1> <p>Your dream home awaits. Discover a place you'll love to live.</p> <Button as={Link} to="/properties" variant="primary" size="lg">View All Properties</Button> </Container> </div> <Container className="my-5"> <Row className="text-center mb-4"> <Col> <h2>Find Your Perfect Home</h2> <p>We offer a wide range of properties to suit your needs and budget. Whether you are looking for a luxurious villa, a modern apartment, or a cozy cottage, we have something for everyone.</p> </Col> </Row> <Row className="mb-4"> <Col> <h3>Featured Properties</h3> <Row> {featuredProperties.map(property => ( <Col key={property.id} sm={12} md={6} lg={4} className="mb-4"> <Card className="property-card"> <Card.Img variant="top" src={property.image} /> <Card.Body> <Card.Title>{property.title}</Card.Title> <Card.Text>{property.description}</Card.Text> <Button as={Link} to={`/properties/${property.id}`} variant="primary">More Details</Button> </Card.Body> </Card> </Col> ))} </Row> </Col> </Row> <Row className="mb-4"> <Col> <h3>What Our Clients Say</h3> <Carousel> {testimonials.map((testimonial, index) => ( <Carousel.Item key={index}> <Card className="text-center"> <Card.Body> <Card.Img variant="top" src={testimonial.image} className="rounded-circle w-25 mb-3" /> <Card.Text className="blockquote mb-0"> "{testimonial.feedback}" </Card.Text> <footer className="blockquote-footer mt-2">{testimonial.name}</footer> </Card.Body> </Card> </Carousel.Item> ))} </Carousel> </Col> </Row> <Row className="mb-4"> <Col> <h3>Why Choose Us</h3> <Row> <Col md={4} className="mb-4"> <Card className="text-center"> <Card.Body> <Card.Title>Experienced Agents</Card.Title> <Card.Text>Our agents have years of experience in the real estate market.</Card.Text> </Card.Body> </Card> </Col> <Col md={4} className="mb-4"> <Card className="text-center"> <Card.Body> <Card.Title>Customer Focused</Card.Title> <Card.Text>We prioritize our clients' needs and provide personalized service.</Card.Text> </Card.Body> </Card> </Col> <Col md={4} className="mb-4"> <Card className="text-center"> <Card.Body> <Card.Title>Wide Range of Properties</Card.Title> <Card.Text>We offer a diverse range of properties to suit all preferences and budgets.</Card.Text> </Card.Body> </Card> </Col> </Row> </Col> </Row> </Container> </div> ); }; export default Home;
Properties.js
import React from 'react'; import { Container, Row, Col, Card, Button } from 'react-bootstrap'; import { Link } from 'react-router-dom'; import properties from '../data/properties'; const Properties = () => ( <Container className="my-5"> <Row className="mb-4 text-center"> <Col> <h1>Properties</h1> <p>Discover our wide range of properties to find the perfect home for you.</p> </Col> </Row> <Row> {properties.map(property => ( <Col key={property.id} sm={12} md={6} lg={4} className="mb-4"> <Card className="property-card"> <Card.Img variant="top" src={property.image} /> <Card.Body> <Card.Title>{property.title}</Card.Title> <Card.Text>{property.description}</Card.Text> <Button as={Link} to={`/properties/${property.id}`} variant="primary">More Details</Button> </Card.Body> </Card> </Col> ))} </Row> </Container> ); export default Properties;
PropertyDetails.js
import React from 'react'; import { useParams } from 'react-router-dom'; import properties from '../data/properties'; const PropertyDetails = () => { const { id } = useParams(); const property = properties.find(p => p.id === parseInt(id)); if (!property) { return <h1>Property not found</h1>; } return ( <div> <h1>{property.title}</h1> <img src={property.image} alt={property.title} /> <p>{property.description}</p> </div> ); }; export default PropertyDetails;
testimonials.js
const testimonials = [ { name: 'Emily Brown', feedback: 'The team at Real Estate were incredibly helpful and professional. They helped us find our dream home!', image: 'https://via.placeholder.com/150' }, { name: 'Michael Johnson', feedback: 'A wonderful experience from start to finish. Highly recommend their services!', image: 'https://via.placeholder.com/150' } ]; export default testimonials;
6. Style your Website
App.css
body { font-family: Arial, sans-serif; } main { padding: 20px; } .hero { background: url('https://via.placeholder.com/1500x500') no-repeat center center; background-size: cover; color: white; padding: 100px 0; } .carousel-item img { max-height: 500px; object-fit: cover; } blockquote { border-left: 5px solid #ddd; padding-left: 15px; margin-left: 0; margin-right: 0; font-size: 1.2em; } blockquote-footer { font-size: 0.875em; color: #6c757d; } .card-body { display: flex; flex-direction: column; align-items: center; text-align: center; } .card-img-top { width: 100px; height: 100px; object-fit: cover; margin-bottom: 20px; } .property-card img { height: 200px; object-fit: cover; } .property-card { transition: transform 0.2s; } .property-card:hover { transform: scale(1.05); }
7. Integrate Components in App.js
App.js
import React from 'react'; import { BrowserRouter as Router, Route, Routes } from 'react-router-dom'; import NavigationBar from './components/Navbar'; import Footer from './components/Footer'; import Home from './pages/Home'; import About from './pages/About'; import Properties from './pages/Properties'; import Contact from './pages/Contact'; import PropertyDetails from './pages/PropertyDetails'; import './App.css'; import 'bootstrap/dist/css/bootstrap.min.css'; function App() { return ( <Router> <div className="App"> <NavigationBar /> <main className="my-4"> <Routes> <Route path="/" element={<Home />} /> <Route path="/about" element={<About />} /> <Route path="/properties" element={<Properties />} /> <Route path="/contact" element={<Contact />} /> <Route path="/properties/:id" element={<PropertyDetails />} /> </Routes> </main> <Footer /> </div> </Router> ); } export default App;
8. Run Your Application
Run your application using:
npm start
This is a basic template to get you started. You can expand upon this by adding more features such as detailed property pages, contact forms, filtering options, and more.
This is it guys and if you will have any kind of query, suggestion or requirement then feel free to comment below.
Thanks
Ajay