Hello to all, welcome to therichpost.com. In this post, I will tell you, Angular Reactive Form Set Custom Error Messages Working Example.
Guys with this we will cover below things:
- Angular 14 Reactive Form Implelemtnation.
- Reactive forms validation email with valid format.
- Reactive form checkbox validation.
- Angular14 Reactive Form Responsiveness with Bootstrap 5.
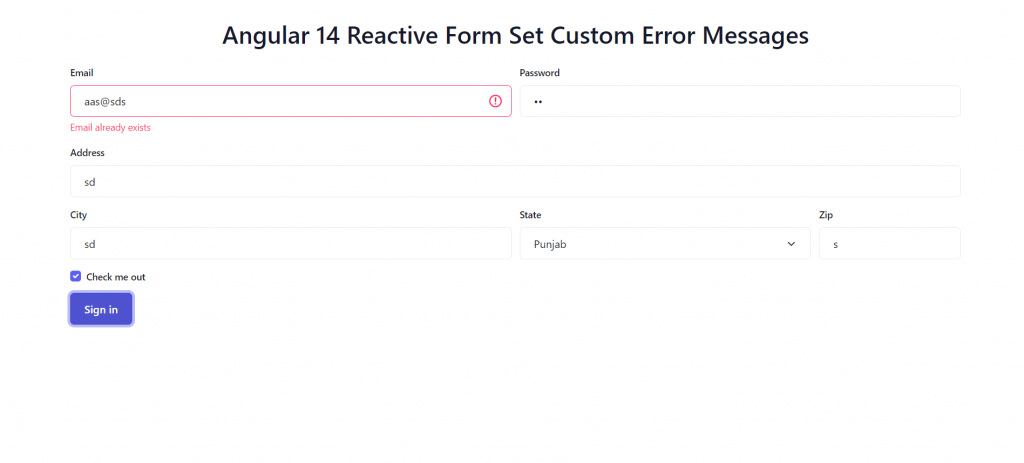
Angular14 came and if you are new then you must check below link:
Here is the code snippet and please use carefully:
1. Very first guys, here are common basics steps to add angular 14 application on your machine and also we must have latest nodejs latest version installed for angular 14:
$ npm install -g @angular/cli $ ng new angularform // Set Angular 14 Application on your pc cd angularform // Go inside project folder
2. Now run below commands to set bootstrap 5 modules into our angular 14 application for responsiveness (optional):
npm install bootstrap npm i @popperjs/core
3. Now friends we just need to add below code into angularform/angular.json file (optional):
"styles": [ ... "node_modules/bootstrap/dist/css/bootstrap.min.css" ], "scripts": [ ... "node_modules/bootstrap/dist/js/bootstrap.min.js" ]
4. Now guys we will add below code into our angularform/src/app/app.module.ts file:
... import { ReactiveFormsModule } from '@angular/forms'; @NgModule({ ... imports: [ ... ReactiveFormsModule, ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
5. Now guys we will add below code into our angularform/src/app/app.component.ts file:
import { Component } from '@angular/core'; import { FormBuilder, FormGroup, Validators } from '@angular/forms'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'angular14demos'; //Form Validables registerForm:any = FormGroup; submitted = false; //custom error message bolean variable customEmailErrorMessage = false; constructor( private formBuilder: FormBuilder){} //Add user form actions get f() { return this.registerForm.controls; } onSubmit() { this.submitted = true; // stop here if form is invalid if (this.registerForm.invalid) { return; } //True if all the fields are filled if(this.submitted) { //demo how we can set custom error messages very easily this.registerForm.controls['email'].setErrors({ incorrect: true}); this.customEmailErrorMessage = true; } } ngOnInit() { //Add User form validations this.registerForm = this.formBuilder.group({ email: ['', [Validators.required, Validators.email]], password: ['', [Validators.required]], address: ['', [Validators.required]], city: ['', [Validators.required]], state: ['', [Validators.required]], zip: ['', [Validators.required]], checkmeout: ['', [Validators.requiredTrue]] }); } }
6. Finally we will add below code into our angularform/src/app/app.component.html file:
<div class="container p-5"> <form class="row g-3" [formGroup]="registerForm" (ngSubmit)="onSubmit()"> <div class="col-md-6"> <label for="inputEmail4" class="form-label">Email</label> <input type="email" formControlName="email" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.email.errors }" id="inputEmail4"> <div *ngIf="submitted && f.email.errors" class="invalid-feedback"> <div *ngIf="f.email.errors.required">Email is required</div> <div *ngIf="f.email.errors.email">Email must be a valid email address</div> <!--custom error message--> <div *ngIf="customEmailErrorMessage">Email already exists</div> </div> </div> <div class="col-md-6"> <label for="inputPassword4" class="form-label">Password</label> <input type="password" formControlName="password" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.password.errors }" id="inputPassword4"> <div *ngIf="submitted && f.password.errors" class="invalid-feedback"> <div *ngIf="f.password.errors.required">Password is required</div> </div> </div> <div class="col-12"> <label for="inputAddress" class="form-label">Address</label> <input type="text" formControlName="address" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.address.errors }" id="inputAddress" placeholder="1234 Main St"> <div *ngIf="submitted && f.address.errors" class="invalid-feedback"> <div *ngIf="f.address.errors.required">Password is required</div> </div> </div> <div class="col-md-6"> <label for="inputCity" class="form-label">City</label> <input placeholder="Ludhiana" type="text" formControlName="city" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.city.errors }" id="inputCity"> <div *ngIf="submitted && f.city.errors" class="invalid-feedback"> <div *ngIf="f.city.errors.required">City is required</div> </div> </div> <div class="col-md-4"> <label for="inputState" class="form-label">State</label> <select id="inputState" class="form-select" formControlName="state" [ngClass]="{ 'is-invalid': submitted && f.state.errors }"> <option value="">Choose...</option> <option value="punjab">Punjab</option> </select> <div *ngIf="submitted && f.state.errors" class="invalid-feedback"> <div *ngIf="f.state.errors.required">State is required</div> </div> </div> <div class="col-md-2"> <label for="inputZip" class="form-label">Zip</label> <input type="text" formControlName="zip" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.zip.errors }" id="inputZip"> <div *ngIf="submitted && f.zip.errors" class="invalid-feedback"> <div *ngIf="f.zip.errors.required">State is required</div> </div> </div> <div class="col-12"> <div class="form-check"> <input value="" class="form-check-input" formControlName="checkmeout" [ngClass]="{ 'is-invalid': submitted && f.checkmeout.errors }" id="inputZip" type="checkbox" id="gridCheck"> <label class="form-check-label" for="gridCheck"> Check me out </label> <div *ngIf="submitted && f.checkmeout.errors" class="invalid-feedback"> <div *ngIf="f.checkmeout.errors.required">This is required</div> </div> </div> </div> <div class="col-12"> <button type="submit" class="btn btn-primary">Sign in</button> </div> </form> </div>
Now we are done friends and please run ng serve command to check the output in browser(locahost:4200) and if you have any kind of query then please do comment below.
Note: Friends, I just tell the basic setup and things, you can change the code according to your requirements. For better understanding please watch video above.
Guys I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad.
Jassa
Thanks