Hello to all, welcome to therichpost.com In this post, I will tell you, Angular datatables with custom button event click open bootstrap modal popup.
This post first part : Angular datatable with print csv excel copy buttons
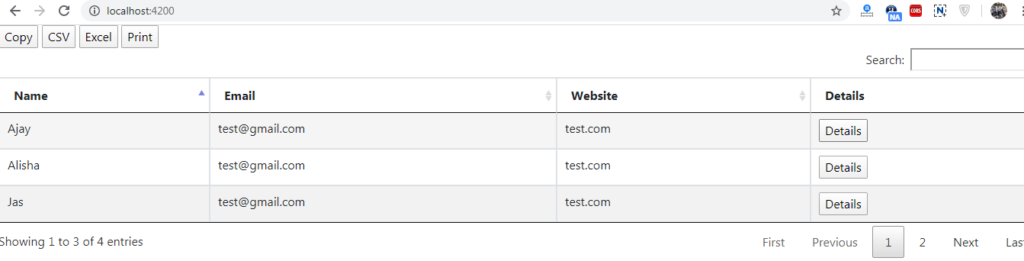
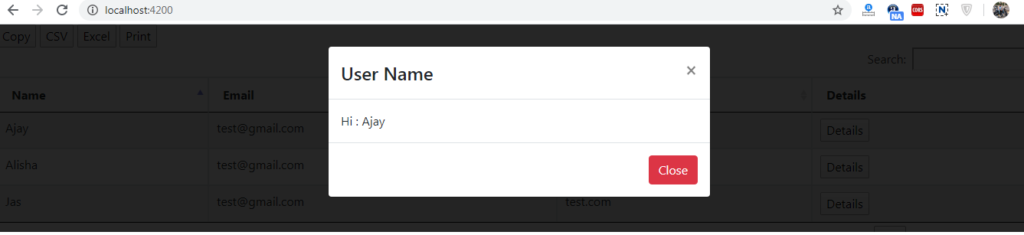
Post Working
In this post, I am adding custom button(details) in my datatable and when I will click on that button then I will get the popup with user details.
To all this action perform, I used jquery and bootstrap also.
Here are complete steps and please follow carefully:
1. Here are the basics commands to install angular 8 on your system:
npm install -g @angular/cli ng new angularpopup //Create new Angular Project $ cd angularpopup // Go inside the Angular Project Folder ng serve --open // Run and Open the Angular Project http://localhost:4200/ // Working Angular Project Url
2. After done with above, you need to run below commands to set bootstrap, datatable, jquery environment into your angular 8 application:
npm install jquery --save npm install datatables.net --save npm install datatables.net-dt --save npm install angular-datatables --save npm install @types/jquery --save-dev npm install @types/datatables.net --save-dev npm install ngx-bootstrap bootstrap --save npm install datatables.net-buttons --save npm install datatables.net-buttons-dt --save npm install @types/datatables.net-buttons --save-dev npm install jszip --save
3. Now you need to add below code into your angular.json file:
... "styles": [ "src/styles.css", "node_modules/datatables.net-dt/css/jquery.dataTables.css", "node_modules/bootstrap/dist/css/bootstrap.min.css", ], "scripts": [ "node_modules/jquery/dist/jquery.js", "node_modules/datatables.net/js/jquery.dataTables.js", "node_modules/bootstrap/dist/js/bootstrap.js", "node_modules/jszip/dist/jszip.js", "node_modules/datatables.net-buttons/js/dataTables.buttons.js", "node_modules/datatables.net-buttons/js/buttons.colVis.js", "node_modules/datatables.net-buttons/js/buttons.flash.js", "node_modules/datatables.net-buttons/js/buttons.html5.js", "node_modules/datatables.net-buttons/js/buttons.print.js" ] ...
4. Now you need to add below code into your src/app/app.module.ts file:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppComponent } from './app.component'; import {DataTablesModule} from 'angular-datatables'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, DataTablesModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
4. Now you need to add below code into your src/app/app.component.ts file:
import { Component, OnInit } from '@angular/core'; declare var $: any @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { public data = [ {name: 'Ajay', email: 'test@gmail.com', website:'test.com'}, {name: 'Alisha', email: 'test@gmail.com', website:'test.com'}, {name: 'Jassa', email: 'test@gmail.com', website:'test.com'}, {name: 'Jas', email: 'test@gmail.com', website:'test.com'}, ]; title = 'angulardatatables'; dtOptions: any = {}; ngOnInit() { this.dtOptions = { pagingType: 'full_numbers', pageLength: 3, processing: true, dom: 'Bfrtip', buttons: [ 'copy', 'csv', 'excel', 'print' ] }; $(document).on( 'click', '.getDetails', function () { $(".username").text(""); $(".username").text($(this).parents("tr").find(".fname").text()); $("#myModal").modal('show'); } ); } }
5. Now you need to add below code into src/app/app.component.html file:
<table class="table table-striped table-bordered table-sm row-border hover" datatable [dtOptions]="dtOptions"> <thead> <tr> <th>Name</th> <th>Email</th> <th>Website</th> <th>Details</th> </tr> </thead> <tbody> <tr *ngFor="let group of data"> <td class="fname">{{group.name}}</td> <td>{{group.email}}</td> <td>{{group.website}}</td> <td><button class="getDetails">Details</button></td> </tr> </tbody> </table> <!-- The Modal --> <div class="modal" id="myModal"> <div class="modal-dialog"> <div class="modal-content"> <!-- Modal Header --> <div class="modal-header"> <h4 class="modal-title">User Name</h4> <button type="button" class="close" data-dismiss="modal">×</button> </div> <!-- Modal body --> <div class="modal-body"> Hi : <span class="username"></span> </div> <!-- Modal footer --> <div class="modal-footer"> <button type="button" class="btn btn-danger" data-dismiss="modal">Close</button> </div> </div> </div> </div>
In the end, don’t forgot to run ng serve command. If you have any query then do comment below. In next part of this post, I will get the dynamic data and show in datatable with angular httpclient.
Jassa
Thank you.
1 Comment