Hello to all, welcome to therichpost.com. In this post, I will tell you, Angular 9 Datatables with custom checkbox selection.
Angular 9 has just launched and it is in very high in demand. Angular 9 increased his performance speed. I am showing the data in Datatables with custom json data and also for giving good look to datatable, I have used bootstrap in it, here is the working picture and don’t forget to install latest node version.
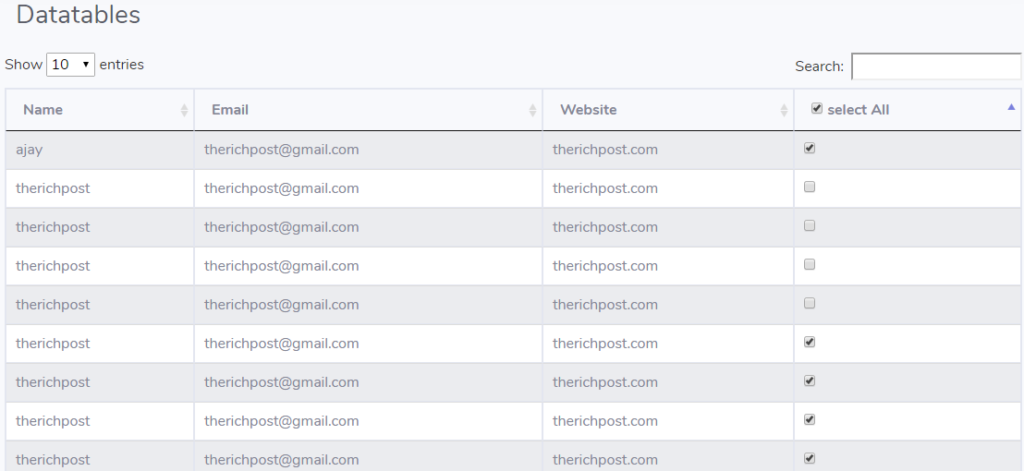
Here is the complete working code and use this carefully:
1. Here are the basics commands, you need to run for latest angular 9 setup and environment:
$ npm install -g @angular/cli $ ng new angulardatatables $ cd angulardatatables $ ng serve //Here is the url, you need to run into your browser and see working angular test project http://localhost:4200/
2. Here are the basics commands, you need to run into your terminal for datatable and its dependencies:
npm install jquery --save npm install datatables.net --save npm install datatables.net-dt --save npm install angular-datatables --save npm install @types/jquery --save-dev npm install @types/datatables.net --save-dev npm install ngx-bootstrap bootstrap --save
3. After done with commands add below code into you angular.json file:
... "styles": [ "src/styles.css", "node_modules/datatables.net-dt/css/jquery.dataTables.css", "node_modules/bootstrap/dist/css/bootstrap.min.css", ], "scripts": [ "node_modules/jquery/dist/jquery.js", "node_modules/datatables.net/js/jquery.dataTables.js", "node_modules/bootstrap/dist/js/bootstrap.js", ] ...
4. Now add below code into your app.module.ts file:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import {DataTablesModule} from 'angular-datatables'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, DataTablesModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
5. Now add below code into app.component.ts file:
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-datatables', templateUrl: './datatables.component.html', styleUrls: ['./datatables.component.css'] }) export class DatatablesComponent implements OnInit { public data = [ {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'ajay', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, {name: 'therichpost', email: 'therichpost@gmail.com', website:'therichpost.com'}, ]; dtOptions: DataTables.Settings = {}; constructor() { } isChecked = false; checkuncheckall() { if(this.isChecked == true) { this.isChecked = false; } else { this.isChecked = true; } } ngOnInit(): void { this.dtOptions = { pagingType: 'full_numbers', pageLength: 10, processing: true }; } }
6. Now add below code into app.component.html file:
<!-- Begin Page Content --> <div class="container-fluid"> <!-- Page Heading --> <div class="d-sm-flex align-items-center justify-content-between mb-4"> <h1 class="h3 mb-0 text-gray-800">Datatables</h1> </div> <!-- Content Row --> <div class="row"> <table width="100%" class="table table-striped table-bordered table-sm row-border hover" datatable [dtOptions]="dtOptions" style="width:100%"> <thead> <tr> <th>Name</th> <th>Email</th> <th>Website</th> <th><input type="checkbox" name="websitecheck" (click) = "checkuncheckall()"> select All</th> </tr> </thead> <tbody> <tr *ngFor="let group of data"> <td>{{group.name}}</td> <td>{{group.email}}</td> <td>{{group.website}}</td> <td><input type="checkbox" name="websitecheck" [checked]="isChecked" value="{{group.website}}"></td> </tr> </tbody> </table> </div> </div> <!-- /.container-fluid --> <style> .dataTables_wrapper{width:100%;} </style>
Don’t forget to run ng serve command to see final output.
Jassa Jatt
Thank you
Hi Ajay 🙂
I am doing some similar things, but additional I have to do that the selected rows could be exported to Excel.
I was trying todo that but I have some problems with thar.
Do you have an idea?
Regards.
Okay I will see, thanks.