Hello to all, welcome to therichpost.com. In this post, I will continue with Angular 8 with php tutorial part 2.
Here you can check the part 1 of this post : Angular 8 with php tutorial.
In this post, I will save the angular form data into php mysql database with the help of angular HttpClient.
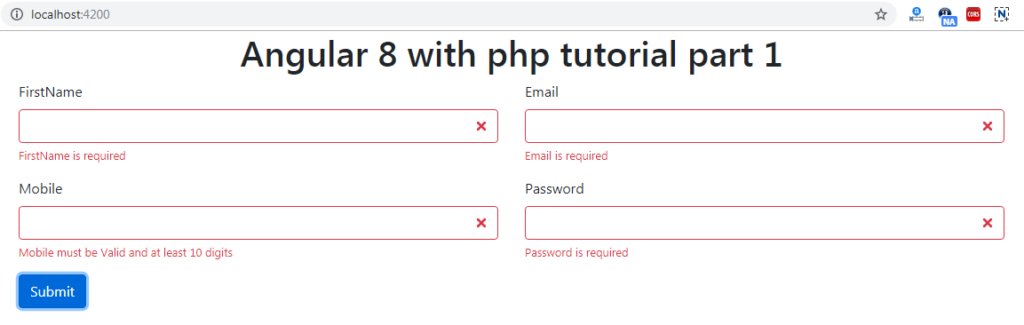
Here are the complete code snippets and please follow carefully:
1. Here are the basics commands to install angular 8 on your system:
npm install -g @angular/cli ng new angularpopup //Create new Angular Project cd angularpopup // Go inside the Angular Project Folder ng serve --open // Run and Open the Angular Project http://localhost:4200/ // Working Angular Project Url
2. After done with above, you need to run below commands to set bootstrap environment into your angular 8 application:
npm install --save bootstrap
3. Now you need to add below code into your angular.json file:
... "styles": [ "src/styles.css", "node_modules/bootstrap/dist/css/bootstrap.min.css" ], "scripts": ["node_modules/bootstrap/dist/js/bootstrap.min.js"] ...
4. Now you need to add below code into your src/app/app.module.ts file:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppComponent } from './app.component'; import { ReactiveFormsModule } from '@angular/forms'; import { HttpClientModule } from '@angular/common/http'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, ReactiveFormsModule, HttpClientModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
5. Now you need to add below code into your src/app/app.component.ts file:
import { Component } from '@angular/core'; import { FormBuilder, FormGroup, Validators } from '@angular/forms'; import { HttpClient, HttpParams } from '@angular/common/http'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { registerForm: FormGroup; submitted = false; posts: any; constructor(private formBuilder: FormBuilder, private http: HttpClient) { } ngOnInit() { this.registerForm = this.formBuilder.group({ email: ['', [Validators.required, Validators.email]], password: ['', [Validators.required, Validators.minLength(6)]], firstname: ['', [Validators.required, Validators.minLength(6)]], mobile: ['', [Validators.required, Validators.pattern(/^-?(0|[1-9]\d*)?$/), Validators.minLength(10)]] }); } // convenience getter for easy access to form fields get f() { return this.registerForm.controls; } onSubmit() { this.submitted = true; // stop here if form is invalid if (this.registerForm.invalid) { return; } if(this.submitted) { // Initialize Params Object const params = new HttpParams({ fromObject: { firstname: this.registerForm.value.firstname, password: this.registerForm.value.password, mobile: this.registerForm.value.mobile, email: this.registerForm.value.email, } }); return this.http.post('http://localhost/mypage.php', params).subscribe(data => { this.posts = data; // show data in console console.log(this.posts); }); } } }
6. Now you need to add below code into src/app/app.component.html file:
<div class="container"> <h1 class="text-center">Angular 8 with php tutorial part 2</h1> <form [formGroup]="registerForm" (ngSubmit)="onSubmit()"> <div class="row"> <div class="col-sm-6"> <div class="form-group"> <label>FirstName</label> <input type="text" formControlName="firstname" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.firstname.errors }" /> <div *ngIf="submitted && f.firstname.errors" class="invalid-feedback"> <div *ngIf="f.firstname.errors.required">FirstName is required</div> </div> </div> </div> <div class="col-sm-6"> <div class="form-group"> <label>Email</label> <input type="text" formControlName="email" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.email.errors }" /> <div *ngIf="submitted && f.email.errors" class="invalid-feedback"> <div *ngIf="f.email.errors.required">Email is required</div> <div *ngIf="f.email.errors.email">Email must be a valid email address</div> </div> </div> </div> <div class="col-sm-6"> <div class="form-group"> <label>Mobile</label> <input type="text" formControlName="mobile" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.mobile.errors }" /> <div *ngIf="submitted && f.mobile.errors" class="invalid-feedback"> <div *ngIf="f.mobile.errors">Mobile must be Valid and at least 10 digits</div> </div> </div> </div> <div class="col-sm-6"> <div class="form-group"> <label>Password</label> <input type="password" formControlName="password" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.password.errors }" /> <div *ngIf="submitted && f.password.errors" class="invalid-feedback"> <div *ngIf="f.password.errors.required">Password is required</div> <div *ngIf="f.password.errors.minlength">Password must be at least 6 characters</div> </div> </div> </div> </div> <button type="submit" class="btn btn-primary">Submit</button> </form> </div>
7. Now you need to add below code into your mypage.php file to connect php mysql and save data in database:
In phpmyadmin, I have created database user and in user database, I have created userdata table, in other words, this code will save data in phpmyadmin. For this, you need xampp software to run php and mysql.
<?php header("Access-Control-Allow-Origin: *"); header("Access-Control-Allow-Methods: PUT, GET, POST"); header("Access-Control-Allow-Headers: Origin, X-Requested-With, Content-Type, Accept"); $conn = new mysqli('localhost','root','','user'); $sql = "INSERT INTO userdata (firstname, password, email, mobile) VALUES ('".$_POST['firstname']."', '".$_POST['password']."', '".$_POST['email']."', '".$_POST['mobile']."')"; if ($conn->query($sql) === TRUE) { echo "New record created successfully"; } else { echo "Error: " . $sql . "<br>" . $conn->error; } $conn->close();
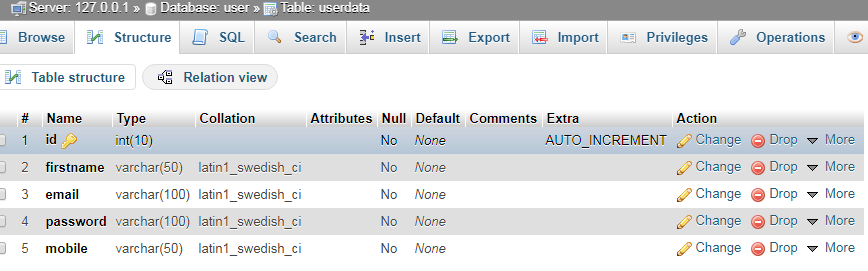
8. Fill the form to save data:
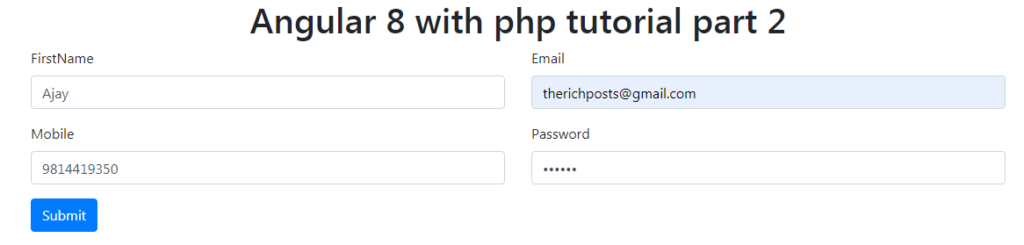
9. Data saved successfully:
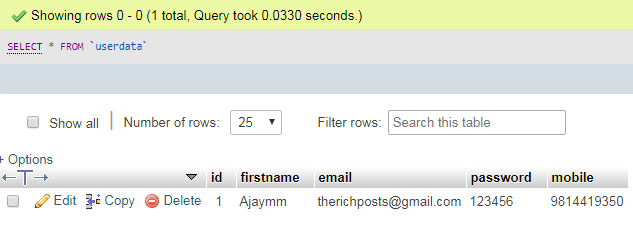
However, In this post, we have successfully added data in php mysql and if you have any kind of query then please me know. In next post, I will tell you, how to get the from php mysql in angular and update it, so please be in touch.
Jassa
Thank you