Hello to all, welcome to therichpost.com. In this post, I will tell you, Angular 16+ Data Sharing | State Management Between Components Using NGRX.
Angular 17 came and if you are new then please check below links:
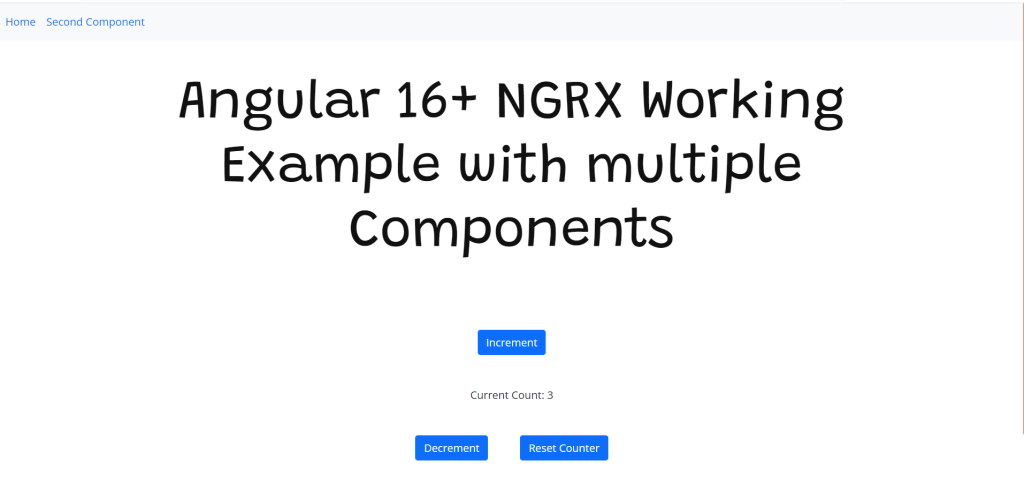
Here is the code snippet and please use it carefully:
1. Very first, you need run below commands into your terminal to get angular 17 fresh setup:
Also you have latest nodejs installed on your system
npm install -g @angular/cli //Setup Angular17 atmosphere ng new angularngrx //Install New Angular App /**You need to update your Nodejs also for this verison**/ cd angularngrx //Go inside the Angular 17 Project
2. Now run below commands into your terminal to get ngrx and bootstrap modules into your angular 17 application:
I use mostly use bootstrap to make application looks good
npm install bootstrap --save npm install @ngrx/store --save
3. Now add below code into your angular.json file:
"styles": [ ... "node_modules/bootstrap/dist/css/bootstrap.min.css", ], ...
4. Now you have to create counter.actions.ts file inside src/app folder and add below code into that file:
import { createAction } from '@ngrx/store'; export const increment = createAction('[Counter Component] Increment'); export const decrement = createAction('[Counter Component] Decrement'); export const reset = createAction('[Counter Component] Reset');
5. Now create counter.reducer.ts file inside src/app folder and add below code into that file:
import { createReducer, on } from '@ngrx/store'; import { increment, decrement, reset } from './counter.actions'; export const initialState = 0; const _counterReducer = createReducer(initialState, on(increment, state => state + 1), on(decrement, state => state - 1), on(reset, state => 0), ); export function counterReducer(state:any, action:any) { return _counterReducer(state, action); }
6. Now run below command into your terminal to create new component:
ng g c componentsecond
7. Now add below code into your src/app/app.module.ts file:
... import { StoreModule } from '@ngrx/store'; import { counterReducer } from './counter.reducer'; import { Routes, RouterModule } from '@angular/router'; const appRoutes: Routes = [ { path: 'secondcomponent', component: ComponentsecondComponent } ]; ... imports: [ ... StoreModule.forRoot({ count: counterReducer }), RouterModule.forRoot( appRoutes, { enableTracing: true } // <-- debugging purposes only ) ],
8. Now add below code into src/app/app/component.ts file:
... import { Store, select } from '@ngrx/store'; import { Observable } from 'rxjs'; import { increment, decrement, reset } from './counter.actions'; ... export class AppComponent { ... count$: Observable<number>; constructor( private store: Store<{ count: number }>){ this.count$ = store.pipe(select('count')); } increment() { this.store.dispatch(increment()); } decrement() { this.store.dispatch(decrement()); } reset() { this.store.dispatch(reset()); } }
9. Now add below into src/app/app.component.html file:
<nav class="navbar navbar-expand-sm bg-light"> <!-- Links --> <ul class="navbar-nav"> <li class="nav-item"> <a class="nav-link" [routerLink]="['/']">Home</a> </li> <li class="nav-item"> <a class="nav-link" [routerLink]="['/secondcomponent']">Second Component</a> </li> </ul> </nav> <div class="container text-center"> <h1 class="mt-5 mb-5">Angular 17 NGRX Working Example with multiple Components</h1> <button class="btn btn-primary mb-5 mt-5" id="increment" (click)="increment()">Increment</button> <div>Current Count: {{ count$ | async }}</div> <button class="btn btn-primary mb-5 mt-5 mr-5" id="decrement" (click)="decrement()">Decrement</button> <button class="btn btn-primary" id="reset" (click)="reset()">Reset Counter</button> <router-outlet></router-outlet> </div>
10. Now add below into your src/app/componentsecond/componentsecond.component.ts file:
... import { Store, select } from '@ngrx/store'; import { Observable } from 'rxjs'; export class ComponentsecondComponent implements OnInit { ... count$: Observable<number>; constructor( private store: Store<{ count: number }>) { this.count$ = store.pipe(select('count')); } }
11. Finally add below into your src/app/componentsecond/componentsecond.component.html file:
<div>Current Count: {{ count$ | async }}</div>
This is it and don’t forget to run ng serve command in the end. If you have any kind of query then please do comment below.
Note:
The main purpose of this pot is to tell you, how to easily share data between components in angular 17.
Jassa
Thanks