Hello to all, welcome to therichpost.com. In this post, I will tell you, Bootstrap carousel slider in Angular.
I am adding Bootstrap carousel slider in Angular 8 version.
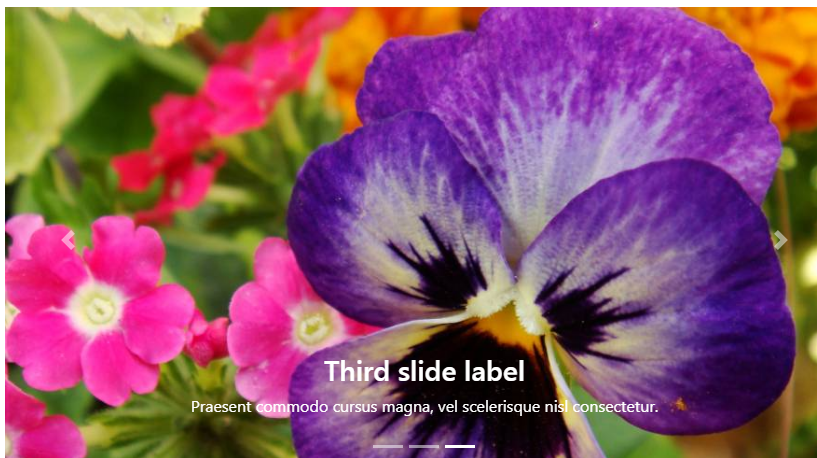
Here is the complete implementation steps and please follow them carefully:
1. Here are the basics commands to install angular 8 on your system:
npm install -g @angular/cli ng new angularslider //Create new Angular Project $ cd angularslider // Go inside the Angular Project Folder ng serve --open // Run and Open the Angular Project http://localhost:4200/ // Working Angular Project Url
2. After done with above, you need to run below commands to set bootstrap carousel environment into your angular 8 application:
ng add ngx-bootstrap --component carousel
3. Now you need to add below code into your src/app/app.module.ts file:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppComponent } from './app.component'; import { CarouselModule } from 'ngx-bootstrap/carousel'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, CarouselModule.forRoot() ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
4. Now you need to add below code into your src/app/app.component.ts file:
import { Component } from '@angular/core'; import { CarouselConfig } from 'ngx-bootstrap/carousel'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'], providers: [ { provide: CarouselConfig, useValue: { interval: 1500, noPause: true, showIndicators: true } } ] }) export class AppComponent { title = 'testfunctions'; }
5. Now you need to add below code into src/app/app.component.html file:
<carousel> <slide> <img src="image1.jpg" alt="first slide" style="display: block; width: 100%;"> <div class="carousel-caption d-none d-md-block"> <h3>First slide label</h3> <p>Nulla vitae elit libero, a pharetra augue mollis interdum.</p> </div> </slide> <slide> <img src="image2.jpg" alt="second slide" style="display: block; width: 100%;"> <div class="carousel-caption d-none d-md-block"> <h3>Second slide label</h3> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit.</p> </div> </slide> <slide> <img src="image3.jpg" alt="third slide" style="display: block; width: 100%;"> <div class="carousel-caption d-none d-md-block"> <h3>Third slide label</h3> <p>Praesent commodo cursus magna, vel scelerisque nisl consectetur.</p> </div> </slide> </carousel>
This is it and don’t forgot to run ng serve command. if you have any query then please do comment below.
Jassa
Thank you