Hello to all, welcome to therichpost.com. In this post, I will tell you, Laravel Rest API in Angular working example.
In this post, I am using latest versions of Angular 7.2.4, Laravel 5.8.
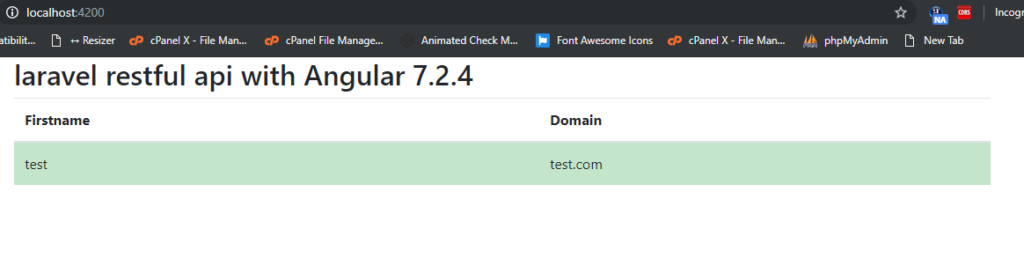
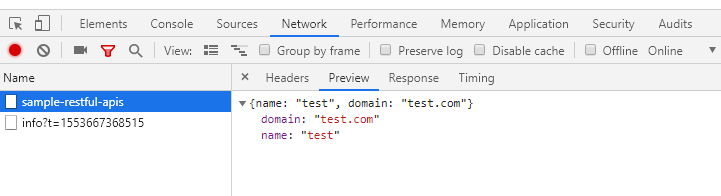
Here is the working code snippets, you need to follow carefully:
1. Here are the commands, you need to run into your terminal to install latest Angular setup:
$ ng new angularlaravelapi cd angularlaravelapi ng serve
2. Here is the code, you need to add into app.module.ts file:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppComponent } from './app.component'; import { HttpClientModule } from '@angular/common/http'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, HttpClientModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
3. Here is the code, you need to add app.component.ts file:
import { Component } from '@angular/core'; import { HttpClient } from '@angular/common/http'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'angularlaravelapi'; Data = new Array(); constructor(private http: HttpClient) { this.http.get('http://localhost/blog/public/api/sample-restful-apis').subscribe(data => { this.Data.push(data); }, error => console.error(error)); } }
4. Here is the code, you need to add app.component.html file:
<!DOCTYPE html> <html lang="en"> <head> <title>Bootstrap Example</title> <meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css"> </head> <body> <div class="container"> <h2>laravel restful api with Angular 7.2.4</h2> <table class="table"> <thead> <tr> <th>Firstname</th> <th>Domain</th> </tr> </thead> <tbody> <tr class="table-success" *ngFor="let data of Data"> <td>{{data.name}}</td> <td>{{data.domain}}</td> </tr> </tbody> </table> </div> </body> </html>
5. Here is the code, you need to add routes/api.php file into you laravel setup:
Route::get('sample-restful-apis', function() { return response()->json([ 'name' => 'test', 'domain' => 'test.com' ]); });
Now you are done, if you have any query related to this post, then please do comment below or ask question.
Jassa jatt
Thank you.