Hello friends, welcome back to my blog. Today in this blog post, I am going to show you, Reactjs Bootstrap 5 User Login Registration Forms Show Hide on Button Click.
Guy’s with in this post we will do below things:
- Add Bootstrap 5 in Reactjs.
- Show Hide div on button click in reactjs.
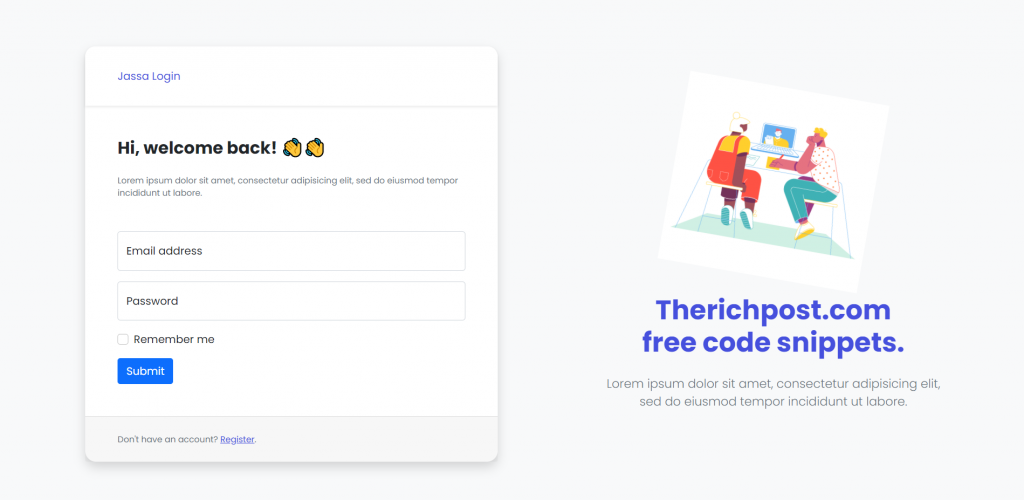
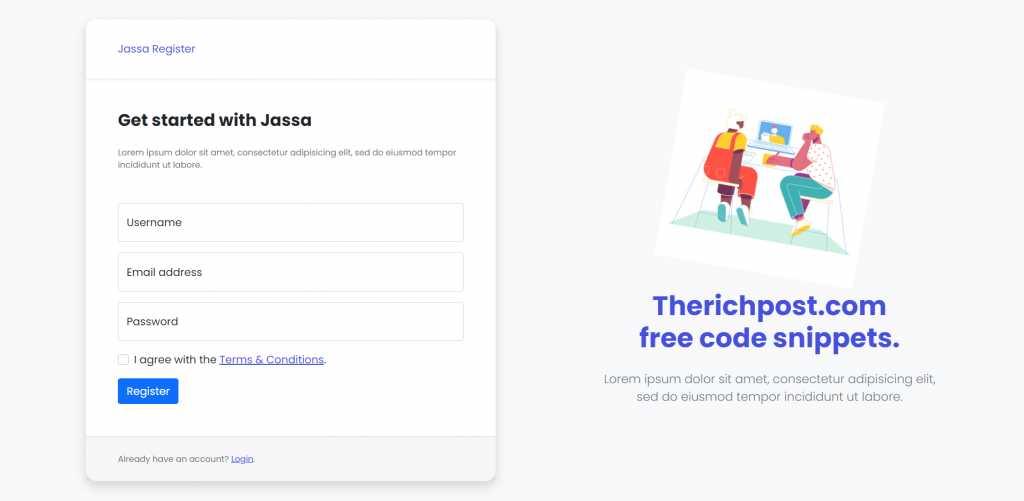
For reactjs and bootstrap 5 new comers, please check the below links:
Friends now I proceed onwards and here is the working code snippet for react ecommerce template free and please use this carefully to avoid the mistakes:
1. Firstly, we need fresh reactjs setup and for that, we need to run below commands into out terminal and also we should have latest node version installed on our system:
npx create-react-app reactboot5 cd reactboot5
2. Now we need to run below commands into our project terminal to get bootstrap and related modules into our reactjs application:
npm install bootstrap --save npm i @popperjs/core npm start //For start project again
3. Finally for the main output, we need to add below code into our reactboot5/src/App.js file or if you have fresh setup then you can replace reactboot5/src/App.js file code with below code:
import React from 'react'; import 'bootstrap/dist/css/bootstrap.min.css'; import './App.css'; class App extends React.Component { //set the state default value constructor(props) { super(props); this.state = {login: 'show col-lg-6 px-lg-4', register: 'hide'}; } showlogin= () => { //button click functionality this.setState({login: 'show col-lg-6 px-lg-4'}); this.setState({register: 'hide'}); } showregister= () => { //button click functionality this.setState({login: 'hide'}); this.setState({register: 'show col-lg-6 px-lg-4'}); } render() { return ( <div className="App"> <div className="page-holder align-items-center py-4 bg-gray-100 vh-100"> <div className="container"> <div className="row align-items-center"> <div className={this.state.login}> <div className="card"> <div className="card-header px-lg-5"> <div className="card-heading text-primary">Jassa Login</div> </div> <div className="card-body p-lg-5"> <h3 className="mb-4">Hi, welcome back! 👋👋</h3> <p className="text-muted text-sm mb-5">Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore.</p> <form id="loginForm" action="index.html"> <div className="form-floating mb-3"> <input className="form-control" id="floatingInput" type="email" placeholder="name@example.com" required /> <label for="floatingInput">Email address</label> </div> <div className="form-floating mb-3"> <input className="form-control" id="floatingPassword" type="password" placeholder="Password" required /> <label for="floatingPassword">Password</label> </div> <div className="form-check mb-3"> <input className="form-check-input" type="checkbox" name="remember" id="remember" /> <label className="form-check-label" for="remember">Remember me</label> </div> <button className="btn btn-primary" type="button">Submit</button> </form> </div> <div className="card-footer px-lg-5 py-lg-4"> <div className="text-sm text-muted">Don't have an account? <a onClick={this.showregister}>Register</a>.</div> </div> </div> </div> {/*} register {*/} <div className={this.state.register}> <div className="card"> <div className="card-header px-lg-5"> <div className="card-heading text-primary">Jassa Register</div> </div> <div className="card-body p-lg-5"> <h3 className="mb-4">Get started with Jassa</h3> <p className="text-muted text-sm mb-5">Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore.</p> <form action="index.html"> <div className="form-floating mb-3"> <input className="form-control" id="username" type="email" placeholder="name@example.com" required /> <label for="username">Username</label> </div> <div className="form-floating mb-3"> <input className="form-control" id="floatingInput" type="email" placeholder="name@example.com" required /> <label for="floatingInput">Email address</label> </div> <div className="form-floating mb-3"> <input className="form-control" id="floatingPassword" type="password" placeholder="Password" required /> <label for="floatingPassword">Password</label> </div> <div className="form-check mb-3"> <input className="form-check-input" type="checkbox" name="agree" id="agree" /> <label className="form-check-label" for="agree">I agree with the <a href="#">Terms & Conditions</a>.</label> </div> <div className="form-group"> <button className="btn btn-primary" id="regidter" type="button" name="registerSubmit">Register</button> </div> </form> </div> <div className="card-footer px-lg-5 py-lg-4"> <div className="text-sm text-muted">Already have an account? <a onClick={this.showlogin} >Login</a>.</div> </div> </div> </div> <div className="col-lg-6 col-xl-5 ms-xl-auto px-lg-4 text-center text-primary"><img className="img-fluid mb-4" width="300" src="https://therichpost.com/wp-content/uploads/2021/06/login_page_image.png" alt="" style={{transform: "rotate(10deg)"}} /> <h1 className="mb-4">Therichpost.com <br className="d-none d-lg-inline" />free code snippets.</h1> <p className="lead text-muted">Lorem ipsum dolor sit amet, consectetur adipisicing elit, sed do eiusmod tempor incididunt ut labore.</p> </div> </div> </div> </div> </div> ) }; } export default App;
4. Now we need to add below code into our reactboot5/src/App.css file:
@import url("https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;500;700&display=swap"); .card-header:first-child { border-radius: calc(1rem - 1px) calc(1rem - 1px) 0 0; } .card-header { position: relative; padding: 2rem 2rem; border-bottom: none; background-color: white; box-shadow: 0 0.125rem 0.25rem rgb(0 0 0 / 8%); z-index: 2; } .card { position: relative; display: flex; flex-direction: column; min-width: 0; word-wrap: break-word; background-color: #fff; background-clip: border-box; border: none; box-shadow: 0 0.5rem 1rem rgb(0 0 0 / 15%); border-radius: 1rem; } .bg-gray-100 { background-color: #f8f9fa !important; } body{ font-family: 'Poppins'!important; } .text-primary { color: #4650dd !important; } h1, .h1, h2, .h2, h3, .h3, h4, .h4, h5, .h5, h6, .h6 { font-weight: 700; line-height: 1.2; } .text-muted { color: #6c757d !important; } .lead { font-size: 1.125rem; font-weight: 300; } .text-sm { font-size: .7875rem !important; } h3, .h3 { font-size: 1.575rem; } .hide { display: none; } a { color: #4650dd!important; text-decoration: underline!important; cursor: pointer; }
Now we are done friends. If you have any kind of query or suggestion or any requirement then feel free to comment below.
Note: Friends, I just tell the basic setup and things, you can change the code according to your requirements.
I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad.
Jassa
Thanks