Hello guys how are you? Welcome back to my blog. Today in this blog we will going to To add DataTables in a React application with filters, you can use the react-data-table-component
library, which provides a simple way to create data tables with built-in filtering, pagination, and sorting. Below are the steps to achieve this:
For react js new comers, please check the below links:
Here’s an improved and more detailed version of the DataTableComponent
to ensure proper functionality:
DataTable Component (DataTable.js)
import React, { useState } from 'react'; import DataTable from 'react-data-table-component'; const DataTableComponent = ({ data }) => { const [filterText, setFilterText] = useState(''); const [resetPaginationToggle, setResetPaginationToggle] = useState(false); const filteredData = data.filter(item => item.name && item.name.toLowerCase().includes(filterText.toLowerCase()) ); const columns = [ { name: 'Name', selector: row => row.name, sortable: true, }, { name: 'Age', selector: row => row.age, sortable: true, }, { name: 'Country', selector: row => row.country, sortable: true, }, ]; const handleFilter = e => { setFilterText(e.target.value); setResetPaginationToggle(!resetPaginationToggle); }; return ( <div> <input type="text" placeholder="Filter by name" value={filterText} onChange={handleFilter} /> <DataTable columns={columns} data={filteredData} pagination paginationResetDefaultPage={resetPaginationToggle} /> </div> ); }; export default DataTableComponent;
Main Application Component (App.js)
import React from 'react'; import DataTableComponent from './DataTable'; const data = [ { id: 1, name: 'John Doe', age: 28, country: 'USA' }, { id: 2, name: 'Jane Smith', age: 34, country: 'UK' }, { id: 3, name: 'Sam Green', age: 23, country: 'Canada' }, // Add more data as needed ]; const App = () => { return ( <div> <h1>Data Table with Filters</h1> <DataTableComponent data={data} /> </div> ); }; export default App;
Key Changes:
- Column Selector: Updated the
selector
to use a functionrow => row.name
instead of a string. This ensures the selector works correctly with the data. - Dependencies: Ensure you have the necessary dependencies installed. Reinstall if necessary:
npm install react-data-table-component # or yarn add react-data-table-component
Running the Application:
Ensure your development server is running:
npm start # or yarn start
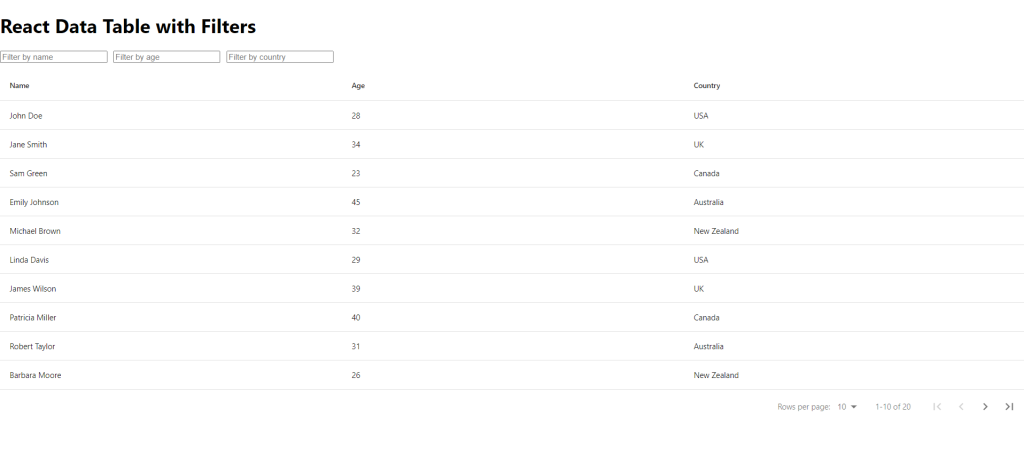
Explanation:
- Column Selector Functions: Using functions (
row => row.name
) in the column definitions ensures the correct access of properties from the data objects. - Data Filtering: The filtering logic remains the same, filtering the data based on the input text.
These changes should address the error and ensure the data table with filtering functionality works correctly in your React application.
This is it guys and if you will have any kind of query, suggestion or requirement then feel free to comment below.
Thanks
jassa