Hello guys, welcome back to my blog therichpost.com. Guys today in this post we will Integrating Angular 18 with SQL Server 2019 using an ASP.NET Core API as the backend.
Angular 18 came. If you are new then you must check below two links:
Now guys here is the complete code snippet and please follow carefully:
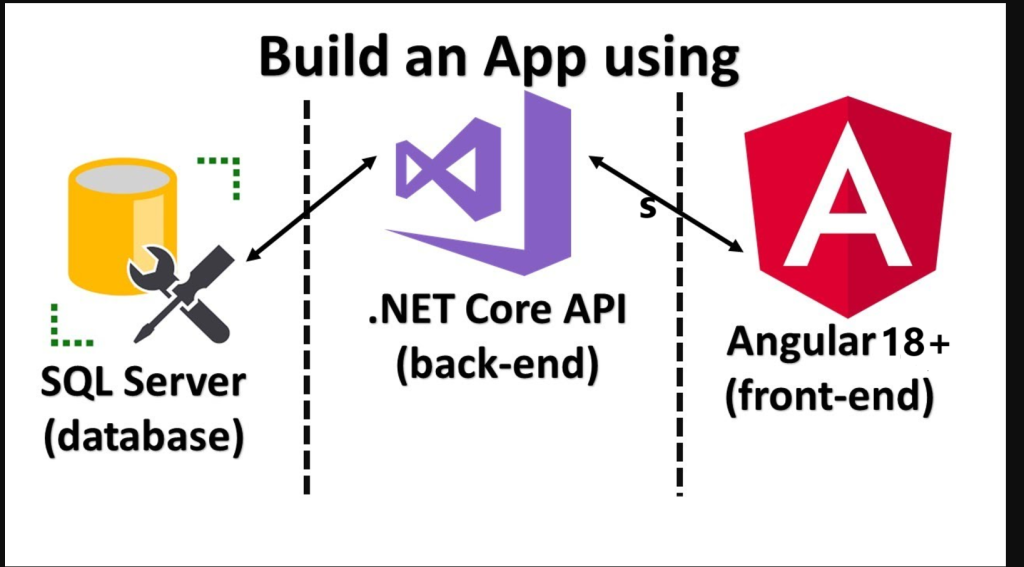
Here’s a complete example of integrating Angular 18 with SQL Server 2019 using an ASP.NET Core API as the backend.
1. Set up SQL Server 2019
Create a database and a table. For example:
CREATE DATABASE SampleDB; USE SampleDB; CREATE TABLE Employees ( Id INT PRIMARY KEY IDENTITY(1,1), Name NVARCHAR(100), Position NVARCHAR(100), Salary DECIMAL(18,2) ); INSERT INTO Employees (Name, Position, Salary) VALUES ('John Doe', 'Software Engineer', 80000);
2. Create the ASP.NET Core API
- Install .NET SDK and create an ASP.NET Core project:
dotnet new webapi -n EmployeeApi cd EmployeeApi
- Add the Entity Framework Core SQL Server package:
dotnet add package Microsoft.EntityFrameworkCore.SqlServer
- Configure the database context in
appsettings.json
:
{ "ConnectionStrings": { "DefaultConnection": "Server=localhost;Database=SampleDB;User Id=your_username;Password=your_password;" } }
- Create the
Employee
model:
public class Employee { public int Id { get; set; } public string Name { get; set; } public string Position { get; set; } public decimal Salary { get; set; } }
- Create the database context:
using Microsoft.EntityFrameworkCore; public class AppDbContext : DbContext { public AppDbContext(DbContextOptions<AppDbContext> options) : base(options) { } public DbSet<Employee> Employees { get; set; } }
- Register the context in
Program.cs
:
builder.Services.AddDbContext<AppDbContext>(options => options.UseSqlServer(builder.Configuration.GetConnectionString("DefaultConnection")));
- Create a controller for the API:
[Route("api/[controller]")] [ApiController] public class EmployeesController : ControllerBase { private readonly AppDbContext _context; public EmployeesController(AppDbContext context) { _context = context; } [HttpGet] public async Task<IActionResult> GetEmployees() { return Ok(await _context.Employees.ToListAsync()); } [HttpPost] public async Task<IActionResult> CreateEmployee([FromBody] Employee employee) { _context.Employees.Add(employee); await _context.SaveChangesAsync(); return Ok(employee); } }
- Run the API:
dotnet run
Test it at http://localhost:5000/api/employees
.
3. Create the Angular Frontend
- Create a new Angular project:
ng new angular-sql-integration cd angular-sql-integration
- Install
HttpClientModule
:
npm install
- Add
HttpClientModule
inapp.module.ts
(Not Preffer just for older servers):
import { HttpClientModule } from '@angular/common/http'; @NgModule({ declarations: [AppComponent], imports: [BrowserModule, HttpClientModule], bootstrap: [AppComponent] }) export class AppModule { }
- Create an Employee service:
ng generate service employee
In employee.service.ts
:
import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Observable } from 'rxjs'; interface Employee { id: number; name: string; position: string; salary: number; } @Injectable({ providedIn: 'root' }) export class EmployeeService { private apiUrl = 'http://localhost:5000/api/employees'; constructor(private http: HttpClient) {} getEmployees(): Observable<Employee[]> { return this.http.get<Employee[]>(this.apiUrl); } createEmployee(employee: Employee): Observable<Employee> { return this.http.post<Employee>(this.apiUrl, employee); } }
- Use the service in
app.component.ts
:
import { Component, OnInit } from '@angular/core'; import { EmployeeService } from './employee.service'; @Component({ selector: 'app-root', template: ` <h1>Employees</h1> <ul> <li *ngFor="let employee of employees"> {{ employee.name }} - {{ employee.position }} - ${{ employee.salary }} </li> </ul> `, }) export class AppComponent implements OnInit { employees: any[] = []; constructor(private employeeService: EmployeeService) {} ngOnInit(): void { this.employeeService.getEmployees().subscribe((data) => { this.employees = data; }); } }
- Run the Angular app:
ng serve
Open it in your browser at http://localhost:4200
.
4. Test the Integration
- Ensure SQL Server, the ASP.NET Core API, and the Angular app are running.
- Verify the employee data is displayed in the Angular app.
This is a complete flow for connecting Angular with SQL Server 2019 using an ASP.NET Core API!
I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad because with your views, I will make my next posts more good and helpful.
Jassa
Thanks