Hello guys how are you? welcome back to my blog therichpost.com. Guys today in this post we are going to implement login and user sign-up functionality for a WordPress WooCommerce site in React Native, Guy we can use the WooCommerce REST API along with WordPress REST API for authentication. Here’s is the code snippet:
1. Setup WordPress REST API Authentication:
Guys WordPress provides the JWT Authentication plugin that we can use to generate tokens for login and sign-up. First, install and activate the JWT Authentication plugin in your WordPress site.
- Install the plugin: JWT Authentication for WP REST API.
- After installing the plugin, configure it by adding the following lines to your
wp-config.php
file:
define('JWT_AUTH_SECRET_KEY', 'your-top-secret-key'); define('JWT_AUTH_CORS_ENABLE', true);
2. Create User Sign-Up API:
Guys we can handle the user registration process using the WordPress API wp-json/wp/v2/users
. Use POST
requests to create new users. This API requires administrator credentials, so ensure you use proper permissions and security measures.
Example API call for user registration:
POST https://yourdomain.com/wp-json/wp/v2/users Authorization: Basic base64_encode("username:password") Body: { "username": "newuser", "email": "newuser@example.com", "password": "password123" }
3. Handle User Login:
Guys we can handle user login by sending a POST
request to the /wp-json/jwt-auth/v1/token
endpoint.
Example login API call:
POST https://yourdomain.com/wp-json/jwt-auth/v1/token Body: { "username": "yourusername", "password": "yourpassword" }
This will return a JWT token that you can use to authenticate the user for further requests.
4. React Native Implementation:
Guys now install the necessary libraries to handle API calls in React Native:
npm install axios react-native-async-storage/async-storage
Example Code for Login:
import axios from 'axios'; import AsyncStorage from '@react-native-async-storage/async-storage'; // Login function const login = async (username, password) => { try { const response = await axios.post('https://yourdomain.com/wp-json/jwt-auth/v1/token', { username: username, password: password }); const token = response.data.token; // Store the token in AsyncStorage for future use await AsyncStorage.setItem('userToken', token); console.log('Login successful:', token); } catch (error) { console.error('Login failed:', error.response.data); } };
Example Code for User Registration:
import axios from 'axios'; const registerUser = async (username, email, password) => { try { const response = await axios.post('https://yourdomain.com/wp-json/wp/v2/users', { username: username, email: email, password: password }, { headers: { Authorization: 'Basic ' + btoa('admin_username:admin_password'), // Use admin credentials here }, }); console.log('User registration successful:', response.data); } catch (error) { console.error('User registration failed:', error.response.data); } };
5. Handling Authenticated Requests:
Guys After login, we can use the stored JWT token to make authenticated requests to the WooCommerce or WordPress API by attaching it to the headers.
const getUserDetails = async () => { const token = await AsyncStorage.getItem('userToken'); const response = await axios.get('https://yourdomain.com/wp-json/wp/v2/users/me', { headers: { Authorization: `Bearer ${token}` } }); console.log('User details:', response.data); };
6. Logout:
Guy for logout, we can simply remove the token from AsyncStorage.
const logout = async () => { await AsyncStorage.removeItem('userToken'); console.log('User logged out'); };
This setup will help you implement login, sign-up, and authenticated requests in your React Native app for WooCommerce and WordPress.
Guys here is also Complete Demo for React Native Login and Signup Forms
Here’s a complete React Native demo with a login and signup form for WordPress WooCommerce.
Dependencies:
Guys Install necessary packages before starting:
npm install axios react-native-async-storage/async-storage
Complete Demo for React Native Login and Signup Forms
import React, { useState } from 'react'; import { View, TextInput, Button, Text, Alert } from 'react-native'; import AsyncStorage from '@react-native-async-storage/async-storage'; import axios from 'axios'; // API Base URL const API_URL = 'https://yourdomain.com/wp-json'; // Authentication component const AuthForm = () => { const [username, setUsername] = useState(''); const [email, setEmail] = useState(''); const [password, setPassword] = useState(''); const [isLogin, setIsLogin] = useState(true); // Toggle between login and signup // Login function const login = async () => { try { const response = await axios.post(`${API_URL}/jwt-auth/v1/token`, { username: username, password: password, }); const token = response.data.token; // Store token in AsyncStorage await AsyncStorage.setItem('userToken', token); Alert.alert('Success', 'Login successful'); } catch (error) { console.error('Login failed:', error.response.data); Alert.alert('Error', 'Login failed. Please try again.'); } }; // Signup function const register = async () => { try { const response = await axios.post( `${API_URL}/wp/v2/users`, { username: username, email: email, password: password, }, { headers: { Authorization: 'Basic ' + btoa('admin_username:admin_password'), // Replace with admin credentials }, } ); Alert.alert('Success', 'User registration successful'); } catch (error) { console.error('Registration failed:', error.response.data); Alert.alert('Error', 'Registration failed. Please try again.'); } }; // Toggle between login and signup const toggleAuthMode = () => { setIsLogin(!isLogin); }; return ( <View style={{ padding: 20 }}> <Text style={{ fontSize: 24, textAlign: 'center', marginBottom: 20 }}> {isLogin ? 'Login' : 'Sign Up'} </Text> <TextInput placeholder="Username" value={username} onChangeText={setUsername} style={{ borderWidth: 1, padding: 10, marginBottom: 10 }} /> {!isLogin && ( <TextInput placeholder="Email" value={email} onChangeText={setEmail} style={{ borderWidth: 1, padding: 10, marginBottom: 10 }} /> )} <TextInput placeholder="Password" value={password} onChangeText={setPassword} secureTextEntry style={{ borderWidth: 1, padding: 10, marginBottom: 10 }} /> <Button title={isLogin ? 'Login' : 'Sign Up'} onPress={isLogin ? login : register} /> <Text style={{ marginTop: 20, textAlign: 'center', color: 'blue' }} onPress={toggleAuthMode} > {isLogin ? 'No account? Sign up' : 'Have an account? Login'} </Text> </View> ); }; export default AuthForm;
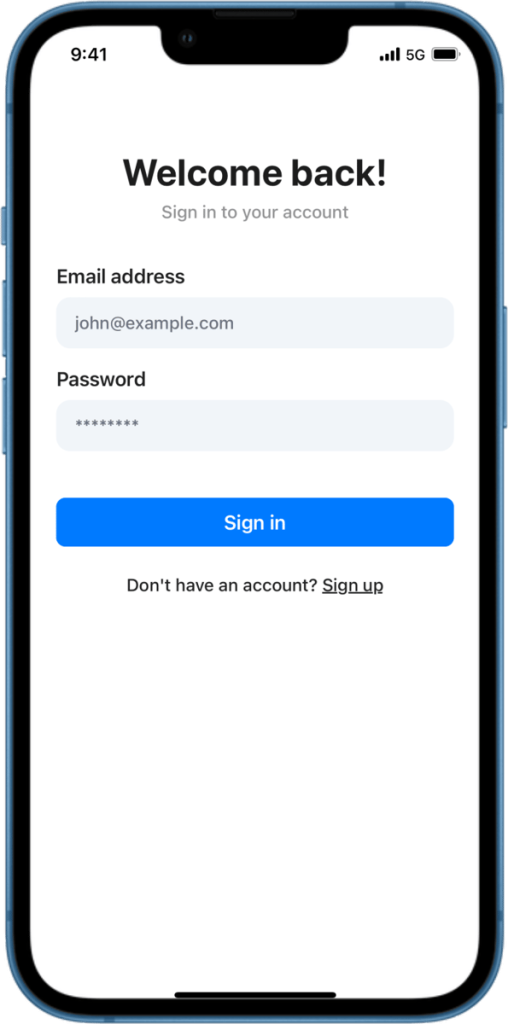
Key Features of This Demo:
- Login & Signup Forms:
- When in login mode, the email field is hidden.
- The form switches between login and signup using the
isLogin
state.
- Handling API Requests:
- Login API sends a request to
jwt-auth/v1/token
. - Signup API sends a request to
wp/v2/users
(admin credentials are required in headers).
- AsyncStorage:
- Stores the JWT token on successful login.
- UI:
- Simple UI with text inputs for username, email, and password.
- A button to submit the form for either login or signup.
- A toggle link for switching between login and signup.
Things to Remember:
- Replace
https://yourdomain.com
with your WordPress site URL. - Use appropriate admin credentials in the signup request.
- Secure the API: Ensure the API is secured to prevent misuse.
This should give you a fully functional login and signup system in your React Native app connected to a WooCommerce WordPress site!
Guys if you will have any kind of query, suggestion and requirement then feel free to comment below.
Thanks
Ajay