Hello to all, welcome to therichpost.com. Today, I am going to tell you, How to share data between two Components in Angular 9?
If you are new in Angular 9, then you can check my old post related to Angular 9.
I am trying this first time and this is very interesting sharing data between Angular 9 components.
I have mainly used two things in this:
- Services:
- I have added custom json data in it and call this Service to Angular Components.
- LocalStorage:
- This is my trick, I have saved component data in it and share one component data into other component with help of localstorage.
Here I am sharing the code and you need to add this into your Angular 9 App:
1. Very first, you need to run common below commands to add Angular 9 project on your machine:
$ npm install -g @angular/cli $ ng new angularsharedata //Install Angular Project $ cd angularsharedata // Go to project folder $ ng generate component component-second //create new component $ ng serve //Run Project http://localhost:4200/ //Run On local server
2. After playing with above commands, you can see folder structure into your src/app folder like below image:
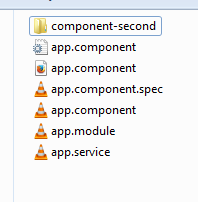
3. Now, you need to create app.service.ts file into src/app folder and below code into it:
import { Injectable } from '@angular/core'; @Injectable() export class appService { constructor() { } public posts = [ {id: 1, firstname: 'Mary', lastname: 'Taylor', age: 24}, {id: 2, firstname: 'Peter', lastname: 'Smith', age: 18}, {id: 3, firstname: 'Lauren', lastname: 'Taylor', age: 31} ]; }
4. Now add below code into your app.module.ts file:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { Routes, RouterModule } from '@angular/router'; import { AppComponent } from './app.component'; import { ComponentSecondComponent } from './component-second/component-second.component'; const appRoutes: Routes = [ { path: 'componentsecondcomponent', component: ComponentSecondComponent } ]; @NgModule({ declarations: [ AppComponent, ComponentSecondComponent ], imports: [ BrowserModule, RouterModule.forRoot(appRoutes), ], providers: [], bootstrap: [AppComponent] }) export class AppModule {}
5. Now add below code into app.component.ts file:
import { Component,OnInit } from '@angular/core'; import { appService } from './app.service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'], providers: [appService] }) export class AppComponent { title = 'angulardata'; public data:any; constructor(private appservice: appService) {} ngOnInit() { this.appservice.posts.push({id: 4, firstname: 'Linda', lastname: 'Maria', age: 30}); this.data = this.appservice.posts; localStorage.setItem("GlobalData",JSON.stringify(this.data)); console.log(this.appservice.posts); } }
6. Now add below code into app.component.html file:
<h1> Welcome to {{ title }}! </h1> <router-outlet></router-outlet>
7. Now add below code into src\app\component-second\component-second.component.ts file:
import { Component, OnInit } from '@angular/core'; @Component({ selector: 'app-component-second', templateUrl: './component-second.component.html', styleUrls: ['./component-second.component.css'] }) export class ComponentSecondComponent implements OnInit { data:any; constructor() {} ngOnInit() { this.data = JSON.parse(localStorage.getItem('GlobalData')); console.log(this.data); } }
8. Now add below code into src\app\component-second\component-second.component.html file:
<h1>Customer List</h1> <ul> <li *ngFor="let customer of data"> {{ customer.firstname}} </li> </ul>
Now run ng serve command and run http://localhost:8000/componentsecondcomponent into your browser and you will see the output like below image:
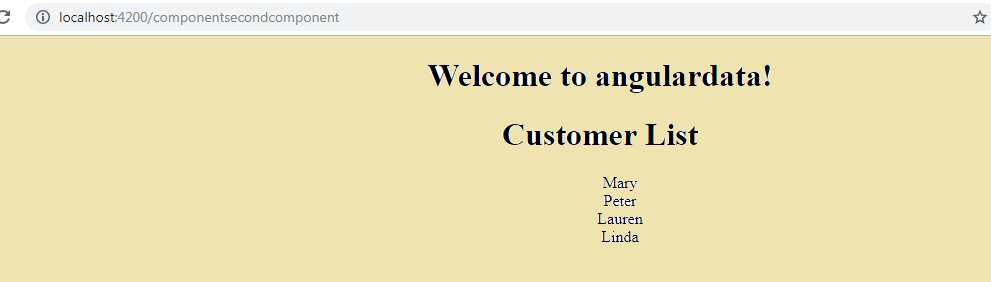
This is done, if you have any query or any have any question then you can comment below.
Harjas
Thank you
Notes: I learnt from this post is that, we can share or we can move all data between the components with the help of LocalStorage.
Recent Comments