Hello to all, welcome to therichpost.com. In this post, I will tell you, How to implement toastr notifications in angular 8?
I am showing toastr notifications in Angular 8 button click event. We can use this in many ways. Here is the working picture of Toastr Notifications in Angular 8 Application:
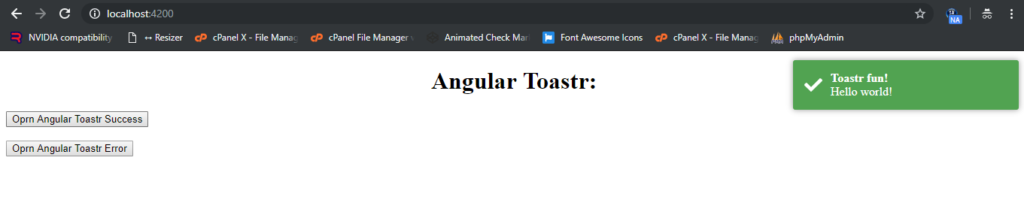
Here are the working steps and please follow carefully:
1. Here are the basics commands to install angular 8 on your system:
npm install -g @angular/cli ng new angulartoastr //Create new Angular Project $ cd angulartoastr // Go inside the Angular Project Folder ng serve --open // Run and Open the Angular Project http://localhost:4200/ // Working Angular Project Url
2. After done with above, you need to run below commands to set toastr environment into your angular 8 application:
npm install ngx-toastr --save npm install @angular/animations --save
3. Now you need to add below code into your angular.json file:
... "styles": [ ... "node_modules/ngx-toastr/toastr.css" ... ] ...
4. Now you need to add below code into your src/app/app.module.ts file:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { BrowserAnimationsModule } from '@angular/platform-browser/animations'; import { ToastrModule } from 'ngx-toastr'; import { AppComponent } from './app.component'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, BrowserAnimationsModule, // required animations module ToastrModule.forRoot() // ToastrModule added ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
5. Now you need to add below code into your src/app/app.component.ts file:
import { Component } from '@angular/core'; import { ToastrService } from 'ngx-toastr'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'angulartoastr'; constructor(private toastr: ToastrService) {} showSuccess() { this.toastr.success('Hello world!', 'Toastr fun!', {timeOut: 2000});; } showError() { this.toastr.error('everything is broken', 'Major Error', { timeOut: 3000 }); } }
5. Now you need to add below code into src/app/app.component.html file:
<div style="text-align:center"> <h1> Angular Toastr: </h1> </div> <button (click) = "showSuccess()">Oprn Angular Toastr Success</button> <br/><br/> <button (click) = "showError()">Oprn Angular Toastr Error</button>
This is it and if you have any query related to this post then please do comment below.
Jassa
Thank you
i have follow your instraction but it,s give me error in console
ngx-toastr.js:264 Uncaught TypeError: Object(…) is not a function
at ngx-toastr.js:264
at Module../node_modules/ngx-toastr/fesm5/ngx-toastr.js (ngx-toastr.js:271)
at __webpack_require__ (bootstrap:78)
at Module../src/app/pages/register/register.component.ts (main.js:2145)
at __webpack_require__ (bootstrap:78)
at Module../src/app/app-routing.module.ts (users.component.ts:8)
at __webpack_require__ (bootstrap:78)
at Module../src/app/app.module.ts (app.component.ts:8)
at __webpack_require__ (bootstrap:78)
at Module../src/main.ts (main.ts:1)
Did you add code in module file?
How to add a custom button with action in toaster ?
can you please tell me more briefly?