Hello guys how are you? Welcome back on my blog Therichpost. Today in this post I am going to share Creating a login form in Angular and connecting it with a backend in Spring Boot.
Angular 19 came. If you are new then you must check below two links:
Now guys here is the complete code snippet and please follow carefully:
Creating a login form in Angular and connecting it with a backend in Spring Boot involves several steps. Below is a guide to implement it:
1. Backend: Spring Boot Setup
Dependencies:
- Spring Web
- Spring Boot Security
- Spring Data JPA (for database integration)
- H2/Any SQL database
- Spring Boot DevTools (optional)
Steps:
- Create a Spring Boot Application:
Generate a new Spring Boot application using Spring Initializr. - Configure Spring Security:
Use basic authentication or JWT for securing endpoints. - Create User Entity:
@Entity public class User { @Id @GeneratedValue(strategy = GenerationType.IDENTITY) private Long id; private String username; private String password; // Getters and Setters }
- User Repository:
public interface UserRepository extends JpaRepository<User, Long> { Optional<User> findByUsername(String username); }
- Service for Authentication:
@Service public class UserService implements UserDetailsService { @Autowired private UserRepository userRepository; @Override public UserDetails loadUserByUsername(String username) throws UsernameNotFoundException { User user = userRepository.findByUsername(username) .orElseThrow(() -> new UsernameNotFoundException("User not found")); return new org.springframework.security.core.userdetails.User(user.getUsername(), user.getPassword(), new ArrayList<>()); } }
- JWT Implementation (Optional):
Implement JWT for stateless authentication by creating token utilities. - REST Controller for Login:
@RestController @RequestMapping("/api/auth") public class AuthController { @PostMapping("/login") public ResponseEntity<?> login(@RequestBody LoginRequest loginRequest) { // Authenticate user and return token or status return ResponseEntity.ok("Login successful"); } }
- Application Properties:
spring.datasource.url=jdbc:h2:mem:testdb spring.datasource.driverClassName=org.h2.Driver spring.datasource.username=sa spring.datasource.password=password spring.jpa.database-platform=org.hibernate.dialect.H2Dialect
2. Frontend: Angular Setup
Steps:
- Install Angular CLI and Create a New App:
npm install -g @angular/cli ng new angular-login cd angular-login
- Install Required Libraries:
npm install @angular/forms @angular/router
- Create Login Component:
ng generate component login
- Login Component Template (
login.component.html
):
<form (ngSubmit)="onSubmit()"> <div> <label for="username">Username:</label> <input type="text" id="username" [(ngModel)]="loginData.username" name="username" required> </div> <div> <label for="password">Password:</label> <input type="password" id="password" [(ngModel)]="loginData.password" name="password" required> </div> <button type="submit">Login</button> </form>
- Login Component Logic (
login.component.ts
):
import { Component } from '@angular/core'; import { HttpClient } from '@angular/common/http'; import { Router } from '@angular/router'; @Component({ selector: 'app-login', templateUrl: './login.component.html', styleUrls: ['./login.component.css'] }) export class LoginComponent { loginData = { username: '', password: '' }; constructor(private http: HttpClient, private router: Router) {} onSubmit() { this.http.post('http://localhost:8080/api/auth/login', this.loginData).subscribe({ next: (response) => { alert('Login successful'); this.router.navigate(['/dashboard']); }, error: (err) => alert('Login failed'), }); } }
- Add Routing (
app-routing.module.ts
):
import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { LoginComponent } from './login/login.component'; const routes: Routes = [ { path: 'login', component: LoginComponent }, { path: '', redirectTo: '/login', pathMatch: 'full' } ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule {}
- App Module Configuration:
ImportFormsModule
andHttpClientModule
.
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { FormsModule } from '@angular/forms'; import { HttpClientModule } from '@angular/common/http'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { LoginComponent } from './login/login.component'; @NgModule({ declarations: [ AppComponent, LoginComponent ], imports: [ BrowserModule, AppRoutingModule, FormsModule, HttpClientModule ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
3. Run and Test
- Start the Spring Boot Backend:
Run the application and ensure the/api/auth/login
endpoint works. - Start the Angular Frontend:
ng serve
- Visit
http://localhost:4200/login
to test the login functionality.
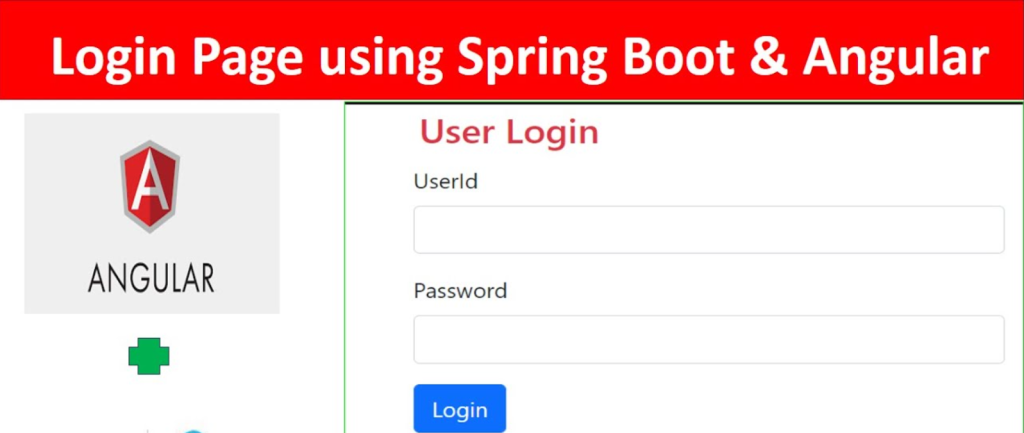
Enhancements
- Use JWT for authentication.
- Secure routes in Angular.
- Handle role-based access in the backend and frontend.
Let me know if you’d like to expand on any part!
Let me know if you need more help! ???? or comment below.
Jassa
Thanks