Hello guys, how are you? Welcome back on my blog therichpost.com. Today in this blog post we will be Creating a Bootstrap 5 modal pop-up with a reactive form and validation in an Angular 17.
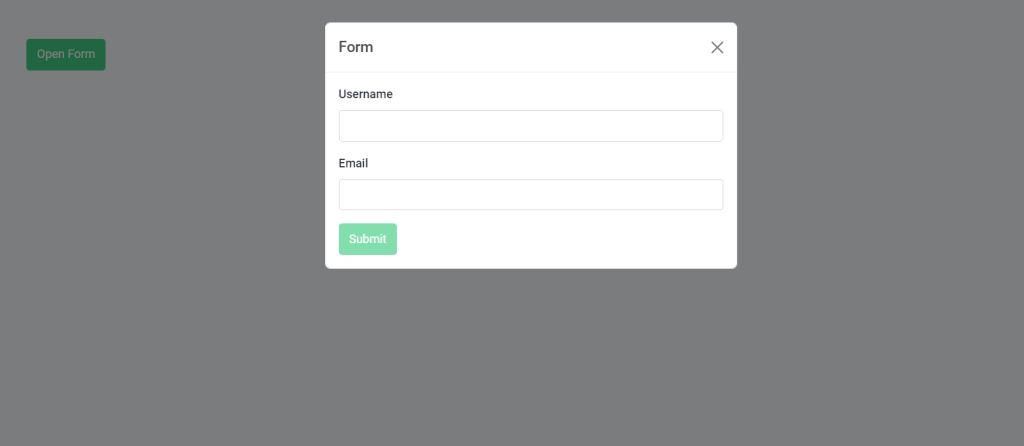
Angular 17 came and Bootstrap 5 also. If you are new then you must check below two links:
Guys now here is the complete code snippet for Creating a Bootstrap 5 modal pop-up with a reactive form and validation in an Angular 17:
1. Firstly friends we need fresh angular 17 setup and for this we need to run below commands but if you already have angular 17 setup then you can avoid below commands. Secondly we should also have latest node version installed on our system:
npm install -g @angular/cli ng new angularforms//Create new Angular Project cd angularforms// Go inside the Angular Project Folder
2. First, ensure that your Angular project is set up and Bootstrap 5 is integrated. You can add Bootstrap to your project by running:
npm install bootstrap
3. Then, include Bootstrap in your project by adding it to the styles, script array in your angular.json file:
"styles": [ "node_modules/bootstrap/dist/css/bootstrap.min.css", "src/styles.css" ], "scripts": [ "node_modules/bootstrap/dist/js/bootstrap.bundle.min.js" ]
4. Now guys we need to add below code inside app.component.ts file to define modal form functionality:
import { Component } from '@angular/core'; import { CommonModule } from '@angular/common'; import { RouterOutlet } from '@angular/router'; import { ReactiveFormsModule } from '@angular/forms'; import { FormBuilder, Validators } from '@angular/forms'; @Component({ selector: 'app-root', standalone: true, imports: [CommonModule, RouterOutlet, ReactiveFormsModule], templateUrl: './app.component.html', styleUrl: './app.component.css' }) export class AppComponent { title = 'angularadmin5'; form = this.fb.group({ username: ['', [Validators.required, Validators.minLength(4)]], email: ['', [Validators.required, Validators.email]], }); constructor(private fb: FormBuilder) { } onSubmit() { console.log(this.form.value); } }
5. Guys now In your component’s template (e.g., app.component.html), create the Bootstrap modal and include the reactive form with validation feedback:
<!-- Button to trigger modal --> <div class="container p-5"> <button type="button" class="btn btn-primary" data-bs-toggle="modal" data-bs-target="#exampleModal"> Open Form </button> <!-- Modal --> <div class="modal fade" id="exampleModal" tabindex="-1" aria-labelledby="exampleModalLabel" aria-hidden="true"> <div class="modal-dialog"> <div class="modal-content"> <div class="modal-header"> <h5 class="modal-title" id="exampleModalLabel">Form</h5> <button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button> </div> <div class="modal-body"> <form [formGroup]="form" (ngSubmit)="onSubmit()"> <div class="mb-3"> <label for="username" class="form-label">Username</label> <input type="text" id="username" class="form-control" formControlName="username"> <div *ngIf="form.get('username')?.invalid && form.get('username')?.touched" class="text-danger"> Username is required and must be at least 4 characters. </div> </div> <div class="mb-3"> <label for="email" class="form-label">Email</label> <input type="email" id="email" class="form-control" formControlName="email"> <div *ngIf="form.get('email')?.invalid && form.get('email')?.touched" class="text-danger"> Please enter a valid email address. </div> </div> <button type="submit" class="btn btn-primary" [disabled]="form.invalid">Submit</button> </form> </div> </div> </div> </div> </div>
This code snippet demonstrates how to create a Bootstrap modal containing a reactive form with simple validation for a username and an email address. The form validation feedback is displayed conditionally based on the state of each form control.
Friends in the end must run ng serve command into your terminal to run the angular 17 project(localhost:4200).
Now we are done friends. If you have any kind of query, suggestion and new requirement then feel free to comment below.
Note: Friends, In this post, I just tell the basic setup and things, you can change the code according to your requirements.
I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad because with your views, I will make my next posts more good and helpful.
Jassa
Thanks