Hello guys, how are you? Welcome back to my blog. In this post we will create a simple ReactJS template with a header, footer, and sidebar, you can set up a basic React application structure using functional components. Below is an example of how you might organize the components and their basic styles using CSS modules.
For react js new comers, please check the below links:
Step 1: Setup
Guys very First, make sure you have Node.js installed. Then, create a new React application and navigate to it:
npx create-react-app react-template cd react-template
Step 2: Create Component Files
Guys In your React application directory, create the following files inside the src
folder:
Header.js
Footer.js
Sidebar.js
Layout.js
You can also create corresponding CSS module files for each component:
Header.module.css
Footer.module.css
Sidebar.module.css
Layout.module.css
Step 3: Define Components
Here’s a simple way to define each component and style them.
Header Component (Header.js
)
import React from 'react'; import styles from './Header.module.css'; function Header() { return ( <header className={styles.header}> <h1>Header</h1> </header> ); } export default Header;
Header.module.css
.header { background-color: #f0f0f0; padding: 10px; text-align: center; }
Footer Component (Footer.js
)
import React from 'react'; import styles from './Footer.module.css'; function Footer() { return ( <footer className={styles.footer}> Footer Content </footer> ); } export default Footer;
Footer.module.css
.footer { background-color: #f0f0f0; padding: 10px; text-align: center; }
Sidebar Component (Sidebar.js
)
import React from 'react'; import styles from './Sidebar.module.css'; function Sidebar() { return ( <div className={styles.sidebar}> Sidebar Content </div> ); } export default Sidebar;
Sidebar.module.css
.sidebar { background-color: #f0f0f0; width: 200px; padding: 10px; }
Layout Component (Layout.js
)
import React from 'react'; import Header from './Header'; import Footer from './Footer'; import Sidebar from './Sidebar'; import styles from './Layout.module.css'; function Layout({ children }) { return ( <div className={styles.layout}> <Header /> <div className={styles.content}> <Sidebar /> <main className={styles.main}> {children} </main> </div> <Footer /> </div> ); } export default Layout;
Layout.module.css
.layout { display: flex; flex-direction: column; height: 100vh; } .content { display: flex; flex: 1; } .main { flex-grow: 1; padding: 10px; }
Step 4: Use Layout in App.js
Finally, use the Layout
component in your App.js
to structure the page:
import React from 'react'; import Layout from './Layout'; function App() { return ( <Layout> <p>Main content goes here</p> </Layout> ); } export default App;
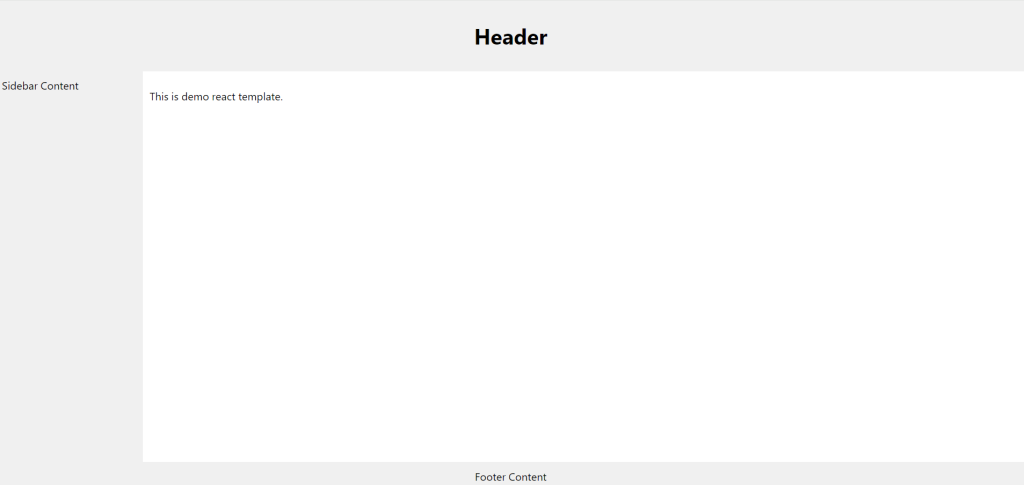
This setup gives you a basic layout with a header, footer, and sidebar, and it’s scalable for more complex applications.
Guys if you will have any kind of query, suggestion and requirements then feel free to comment below.
Note: Friends, I just tell the basic setup and things, you can change the code according to your requirements. For better understanding must watch video above.
Thanks
Remembering Sidhu Moose wala
Recent Comments