Hello to all, welcome to therichpost.com. In this post, I will tell you, Angular 15 Flight Booking Form with Validations Working Example.
Guys with this we will cover below things:
- Angular 15 Reactive Form Implelemtnation.
- Reactive forms validation email with valid format.
- Reactive form checkbox validation.
- Angular15 Reactive Form Responsiveness with Bootstrap 5.
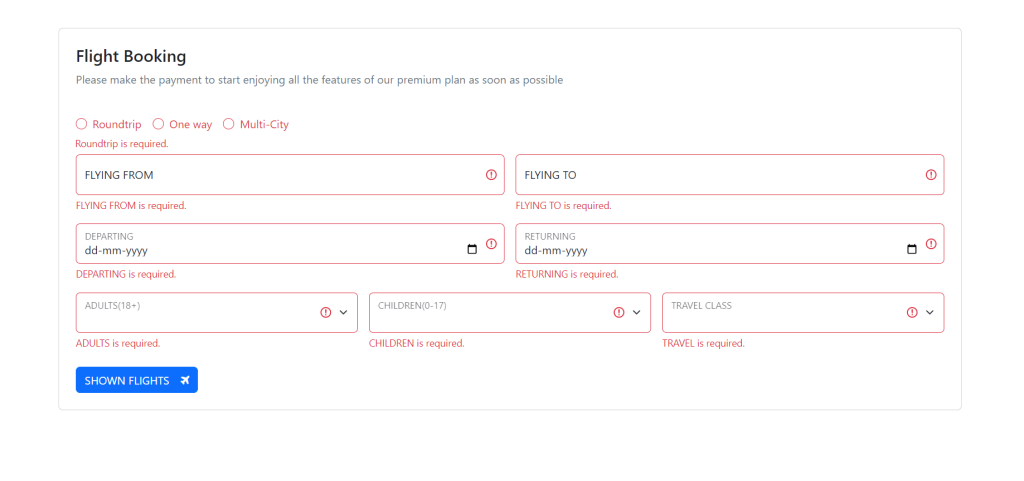
Angular15 came and if you are new then you must check below link:
Here is the code snippet and please use carefully:
1. Very first guys, here are common basics steps to add angular 15 application on your machine and also we must have latest nodejs version(14.17.0) installed for angular 15:
npm install -g @angular/cli ng new angularform // Set Angular 15 Application on your pc cd angularform // Go inside project folder
2. Now run below commands to set bootstrap 5 modules into our angular 15 application for responsiveness (optional):
npm install bootstrap npm i @popperjs/core
3. Now friends we just need to add below code into angularform/angular.json file (optional):
"styles": [ ... "node_modules/bootstrap/dist/css/bootstrap.min.css" ], "scripts": [ ... "node_modules/bootstrap/dist/js/bootstrap.min.js" ]
4. Now guys we will add below code into our angularform/src/app/app.module.ts file:
... import { ReactiveFormsModule } from '@angular/forms'; @NgModule({ ... imports: [ ... ReactiveFormsModule, ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
5. Now guys we will add below code into our angularform/src/app/app.component.ts file:
import { Component } from '@angular/core'; import { FormControl,FormGroup,Validators,FormBuilder } from '@angular/forms'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { //Form variables registerForm:any = FormGroup; submitted = false; constructor( private formBuilder: FormBuilder){} //Add user form actions get f() { return this.registerForm.controls; } onSubmit() { this.submitted = true; // stop here if form is invalid if (this.registerForm.invalid) { return; } //True if all the fields are filled if(this.submitted) { alert("Great!!"); } } ngOnInit() { //Add form validations this.registerForm = this.formBuilder.group({ ffrom: ['', [Validators.required]], fto: ['', [Validators.required]], dparting: ['', [Validators.required]], returning: ['', [Validators.required]], adults: ['', [Validators.required]], children: ['', [Validators.required]], travel: ['', [Validators.required]], roundtripopt: ['', [Validators.required]], }); } }
6. Finally we will add below code into our angularform/src/app/app.component.html file:
<div class="container"> <div class="card p-4 mt-5"> <form [formGroup]="registerForm" (ngSubmit)="onSubmit()"> <div class="row g-3"> <div class="col-12 mb-4"> <h4>Flight Booking</h4> <span class="text-muted">Please make the payment to start enjoying all the features of our premium plan as soon as possible</span> </div> <div class="col-12"> <div class="form-check form-check-inline mb-3"> <input class="form-check-input" type="radio" name="inlineRadioOptions" id="Roundtrip" value="option1" formControlName="roundtripopt" [ngClass]="{ 'is-invalid': submitted && f.roundtripopt.errors }"> <label class="form-check-label" for="Roundtrip">Roundtrip</label> <div *ngIf="submitted && f.roundtripopt.errors" class="invalid-feedback mb" style="display: table;position: absolute;left: 16px;top: 150px;margin-bottom: 5px;"> <div *ngIf="f.roundtripopt.errors.required">Roundtrip is required.</div> </div> </div> <div class="form-check form-check-inline"> <input class="form-check-input" type="radio" name="inlineRadioOptions" id="Oneway" value="option2" formControlName="roundtripopt" [ngClass]="{ 'is-invalid': submitted && f.roundtripopt.errors }"> <label class="form-check-label" for="Oneway">One way</label> </div> <div class="form-check form-check-inline"> <input class="form-check-input" type="radio" name="inlineRadioOptions" id="MultiCity" value="option3" formControlName="roundtripopt" [ngClass]="{ 'is-invalid': submitted && f.roundtripopt.errors }"> <label class="form-check-label" for="MultiCity">Multi-City</label> </div> </div> <div class="col-lg-6 col-md-12"> <div class="form-floating"> <input type="text" placeholder="FLYING FROM" formControlName="ffrom" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.ffrom.errors }"> <div *ngIf="submitted && f.ffrom.errors" class="invalid-feedback"> <div *ngIf="f.ffrom.errors.required">FLYING FROM is required.</div> </div> <label>FLYING FROM</label> </div> </div> <div class="col-lg-6 col-md-12"> <div class="form-floating"> <input type="text" placeholder="FLYING TO" formControlName="fto" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.fto.errors }"> <div *ngIf="submitted && f.fto.errors" class="invalid-feedback"> <div *ngIf="f.fto.errors.required">FLYING TO is required.</div> </div> <label>FLYING TO</label> </div> </div> <div class="col-lg-6 col-md-12"> <div class="form-floating"> <input type="date" placeholder="DEPARTING" formControlName="dparting" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.dparting.errors }"> <div *ngIf="submitted && f.dparting.errors" class="invalid-feedback"> <div *ngIf="f.dparting.errors.required">DEPARTING is required.</div> </div> <label>DEPARTING</label> </div> </div> <div class="col-lg-6 col-md-12"> <div class="form-floating"> <input type="date" placeholder="RETURNING" formControlName="returning" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.returning.errors }"> <div *ngIf="submitted && f.returning.errors" class="invalid-feedback"> <div *ngIf="f.returning.errors.required">RETURNING is required.</div> </div> <label>RETURNING</label> </div> </div> <div class="col-lg-4 col-md-12"> <div class="form-floating"> <select class="form-select" formControlName="adults" [ngClass]="{ 'is-invalid': submitted && f.adults.errors }"> <option selected="" hidden="">Open this select menu</option> <option value="1">One</option> <option value="2">Two</option> <option value="3">Three</option> </select> <div *ngIf="submitted && f.adults.errors" class="invalid-feedback"> <div *ngIf="f.adults.errors.required">ADULTS is required.</div> </div> <label>ADULTS(18+)</label> </div> </div> <div class="col-lg-4 col-md-12"> <div class="form-floating"> <select class="form-select" formControlName="children" [ngClass]="{ 'is-invalid': submitted && f.children.errors }"> <option selected="" hidden="">Open this select menu</option> <option value="1">One</option> <option value="2">Two</option> <option value="3">Three</option> </select> <div *ngIf="submitted && f.children.errors" class="invalid-feedback"> <div *ngIf="f.children.errors.required">CHILDREN is required.</div> </div> <label>CHILDREN(0-17)</label> </div> </div> <div class="col-lg-4 col-md-12"> <div class="form-floating"> <select class="form-select" formControlName="travel" [ngClass]="{ 'is-invalid': submitted && f.travel.errors }"> <option selected="" hidden="">Open this select menu</option> <option value="1">Economy</option> <option value="2">Premium</option> <option value="3">Business</option> </select> <div *ngIf="submitted && f.travel.errors" class="invalid-feedback"> <div *ngIf="f.travel.errors.required">TRAVEL is required.</div> </div> <label>TRAVEL CLASS</label> </div> </div> <div class="col-12 mt-4"> <button class="btn btn-primary text-uppercase" type="submit">SHOWN FLIGHTS<i class="fa fa-plane ms-3"></i></button> </div> </div> </form> </div> </div>
7. Now we will add below font awesome icons link into our angularform/src/index.html file:
<!doctype html> <html lang="en"> <head> ... <link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.0.3/css/font-awesome.css"/> </head>
Now we are done friends and please run ng serve command to check the output in browser(locahost:4200) and if you have any kind of query then please do comment below.
Note: Friends, I just tell the basic setup and things, you can change the code according to your requirements. For better understanding please watch video above.
Guys I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad.
Jassa
Thanks
Recent Comments