Hello to all, welcome to therichpost.com. In this post, I will tell you, Angular 15 Doctor Appointment Booking Form Template with Validations Working Demo.
Guys with this we will cover below things:
- Angular 15 Reactive Form Implelemtnation.
- Reactive forms validation email with valid format.
- Reactive form checkbox validation.
- Angular15 Reactive Form Responsiveness with Bootstrap 5.
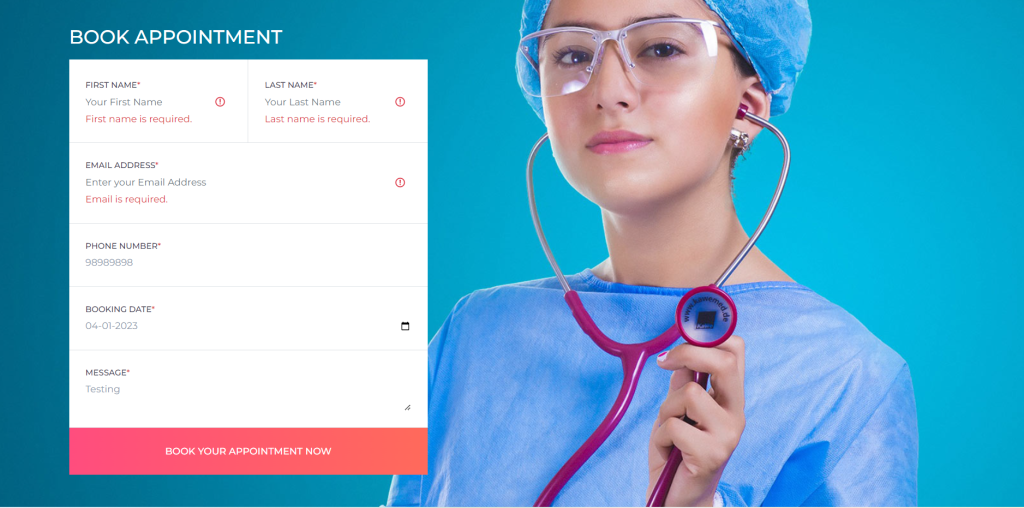
Angular15 came and if you are new then you must check below link:
Here is the code snippet and please use carefully:
1. Very first guys, here are common basics steps to add angular 15 application on your machine and also we must have latest nodejs version(14.17.0) installed for angular 15:
npm install -g @angular/cli ng new angularform // Set Angular 15 Application on your pc cd angularform // Go inside project folder
2. Now run below commands to set bootstrap 5 modules into our angular 15 application for responsiveness (optional):
npm install bootstrap npm i @popperjs/core
3. Now friends we just need to add below code into angularform/angular.json file (optional):
"styles": [ ... "node_modules/bootstrap/dist/css/bootstrap.min.css" ], "scripts": [ ... "node_modules/bootstrap/dist/js/bootstrap.min.js" ]
4. Now guys we will add below code into our angularform/src/app/app.module.ts file:
... import { ReactiveFormsModule } from '@angular/forms'; @NgModule({ ... imports: [ ... ReactiveFormsModule, ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
5. Now guys we will add below code into our angularform/src/app/app.component.ts file:
import { Component } from '@angular/core'; import { FormControl,FormGroup,Validators,FormBuilder } from '@angular/forms'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { //Form variables registerForm:any = FormGroup; submitted = false; constructor( private formBuilder: FormBuilder){} //Add user form actions get f() { return this.registerForm.controls; } onSubmit() { this.submitted = true; // stop here if form is invalid if (this.registerForm.invalid) { return; } //True if all the fields are filled if(this.submitted) { alert("Great!!"); } } ngOnInit() { //Add form validations this.registerForm = this.formBuilder.group({ fname: ['', [Validators.required]], lname: ['', [Validators.required]], email: ['', [Validators.required]], phone: ['', [Validators.required]], date: ['', [Validators.required]], message: ['', [Validators.required]], }); } }
6. Finally we will add below code into our angularform/src/app/app.component.html file:
<div class="banner3"> <div class="py-5 banner" style="background-image:url(https://therichpost.com/wp-content/uploads/2023/01/banner-bg-book.jpg);"> <div class="container"> <form [formGroup]="registerForm" (ngSubmit)="onSubmit()"> <div class="row"> <div class="col-md-7 col-lg-5"> <h3 class="my-3 text-white font-weight-medium text-uppercase">Book Appointment</h3> <div class="bg-white"> <div class="form-row border-bottom"> <div class="p-4 left border-right w-50"> <label class="text-inverse font-12 text-uppercase">First Name<span class="text-danger">*</span></label> <input type="text" class="border-0 p-0 font-14 form-control" placeholder="Your First Name" formControlName="fname" [ngClass]="{ 'is-invalid': submitted && f.fname.errors }" /> <div *ngIf="submitted && f.fname.errors" class="invalid-feedback mb"> <div *ngIf="f.fname.errors.required">First name is required.</div> </div> </div> <div class="p-4 right w-50"> <label class="text-inverse font-12 text-uppercase">Last Name<span class="text-danger">*</span></label> <input type="text" class="border-0 p-0 font-14 form-control" placeholder="Your Last Name" formControlName="lname" [ngClass]="{ 'is-invalid': submitted && f.fname.errors }" /> <div *ngIf="submitted && f.lname.errors" class="invalid-feedback mb"> <div *ngIf="f.lname.errors.required">Last name is required.</div> </div> </div> </div> <div class="form-row border-bottom p-4"> <label class="text-inverse font-12 text-uppercase">Email Address<span class="text-danger">*</span></label> <input type="text" class="border-0 p-0 font-14 form-control" placeholder="Enter your Email Address" formControlName="email" [ngClass]="{ 'is-invalid': submitted && f.email.errors }" /> <div *ngIf="submitted && f.email.errors" class="invalid-feedback mb"> <div *ngIf="f.email.errors.required">Email is required.</div> </div> </div> <div class="form-row border-bottom p-4"> <label class="text-inverse font-12 text-uppercase">Phone Number<span class="text-danger">*</span></label> <input type="text" class="border-0 p-0 font-14 form-control" placeholder="Enter your Phone Number" formControlName="phone" [ngClass]="{ 'is-invalid': submitted && f.phone.errors }" /> <div *ngIf="submitted && f.phone.errors" class="invalid-feedback mb"> <div *ngIf="f.phone.errors.required">Phone is required.</div> </div> </div> <div class="form-row border-bottom p-4 position-relative"> <label class="text-inverse font-12 text-uppercase">Booking Date<span class="text-danger">*</span></label> <div class="input-group date"> <input type="date" class="border-0 p-0 font-14 form-control" id="dp" placeholder="Select the Appointment Date" formControlName="date" [ngClass]="{ 'is-invalid': submitted && f.date.errors }" /> <div *ngIf="submitted && f.date.errors" class="invalid-feedback mb"> <div *ngIf="f.date.errors.required">Date is required.</div> </div> </div> </div> <div class="form-row border-bottom p-4"> <label class="text-inverse font-12 text-uppercase">Message<span class="text-danger">*</span></label> <textarea col="1" row="1" class="border-0 p-0 font-weight-light font-14 form-control" placeholder="Write Down the Message" formControlName="message" [ngClass]="{ 'is-invalid': submitted && f.message.errors }"></textarea> <div *ngIf="submitted && f.message.errors" class="invalid-feedback mb"> <div *ngIf="f.message.errors.required">Message is required.</div> </div> </div> <div> <button type="submit" class="w-100 m-0 border-0 py-4 font-14 font-weight-medium btn btn-danger-gradiant btn-block position-relative rounded-0 text-center text-white text-uppercase"> <span>Book Your Appointment Now</span> </button> </div> </div> </div> </div> </form> </div> </div> </div>
7. Now we will add below custom style into our angularform/src/app/app.component.css file:
@import url(//fonts.googleapis.com/css?family=Montserrat:400,500,700); .banner3 { font-family: "Montserrat", sans-serif; color: #8d97ad; font-weight: 300; max-height: 800px; } .banner3 .banner { position: relative; max-height: 700px; background-size: cover; background-repeat: no-repeat; background-position: center top; width: 100%; display: table; } .banner3 h1, .banner3 h2, .banner3 h3, .banner3 h4, .banner3 h5, .banner3 h6 { color: #3e4555; } .banner3 .font-weight-medium { font-weight: 500; } .banner3 .subtitle { color: #8d97ad; line-height: 24px; } .banner3 .btn-danger-gradiant { background: #ff4d7e; background: -webkit-linear-gradient(legacy-direction(to right), #ff4d7e 0%, #ff6a5b 100%); background: -webkit-gradient(linear, left top, right top, from(#ff4d7e), to(#ff6a5b)); background: -webkit-linear-gradient(left, #ff4d7e 0%, #ff6a5b 100%); background: -o-linear-gradient(left, #ff4d7e 0%, #ff6a5b 100%); background: linear-gradient(to right, #ff4d7e 0%, #ff6a5b 100%); border: 0px; } .banner3 .btn-danger-gradiant:hover { background: #ff6a5b; background: -webkit-linear-gradient(legacy-direction(to right), #ff6a5b 0%, #ff4d7e 100%); background: -webkit-gradient(linear, left top, right top, from(#ff6a5b), to(#ff4d7e)); background: -webkit-linear-gradient(left, #ff6a5b 0%, #ff4d7e 100%); background: -o-linear-gradient(left, #ff6a5b 0%, #ff4d7e 100%); background: linear-gradient(to right, #ff6a5b 0%, #ff4d7e 100%); } .banner3 .btn-danger-gradiant.active, .banner3 .btn-danger-gradiant:active, .banner3 .btn-danger-gradiant:focus { -webkit-box-shadow: 0px; box-shadow: 0px; opacity: 1; } .banner3 .btn-md { padding: 15px 45px; font-size: 16px; } .banner3 .form-row { margin: 0; } .banner3 label.font-12 { font-size: 12px; font-weight: 500; margin-bottom: 5px; } .banner3 .form-control { color: #8d97ad; -o-text-overflow: ellipsis; text-overflow: ellipsis; } .banner3 .date label { cursor: pointer; margin: 0; } @media (max-width: 370px) { .banner3 .left, .banner3 .right { padding: 25px; } } @media (max-width: 320px) { .banner3 .left, .banner3 .right { padding: 25px 15px; } } .banner3 .font-14 { font-size: 14px; } .banner3 .text-inverse { color: #3e4555 !important; } .form-row { display: -ms-flexbox; display: flex; -ms-flex-wrap: wrap; flex-wrap: wrap; margin-right: -5px; margin-left: -5px; } .border-bottom { border-bottom: 1px solid #dee2e6!important; } .border-right { border-right: 1px solid #dee2e6!important; }
Now we are done friends and please run ng serve command to check the output in browser(locahost:4200) and if you have any kind of query then please do comment below.
Note: Friends, I just tell the basic setup and things, you can change the code according to your requirements. For better understanding please watch video above.
Guys I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad.
Jassa
Thanks