Hello to all welcome back on my blog therichpost.com. Today in this blog post, I am going to tell you, Angular 12 Crud Tutorial with Services Part 4 – Update User.
Important : Guy’s before stating with this, please check the below links for basic angular 12 setup with services:
Angular12 Crud Tutorial with Services Part 1 – Add User
Angular 12 Crud Tutorial with Services Part 2 – Delete User
Angular 12 Crud Tutorial with Services Part 3 – View User
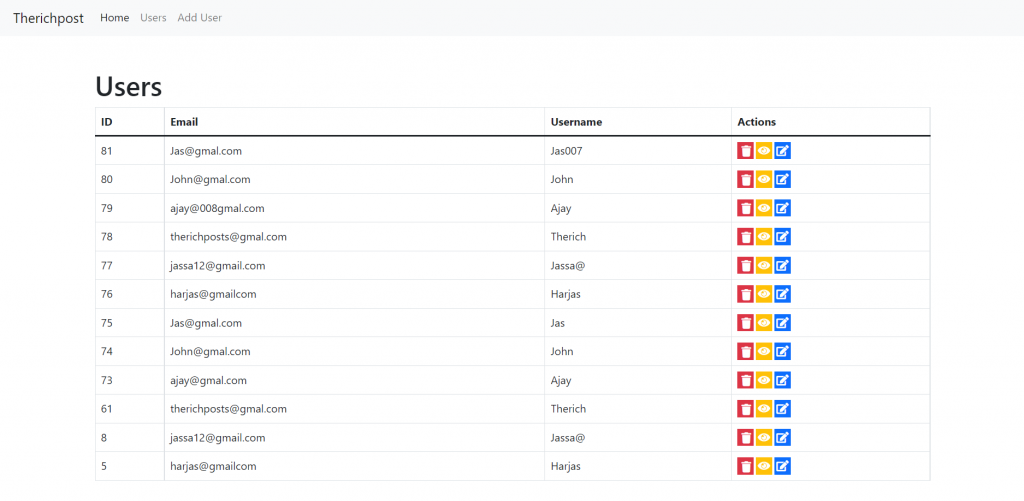
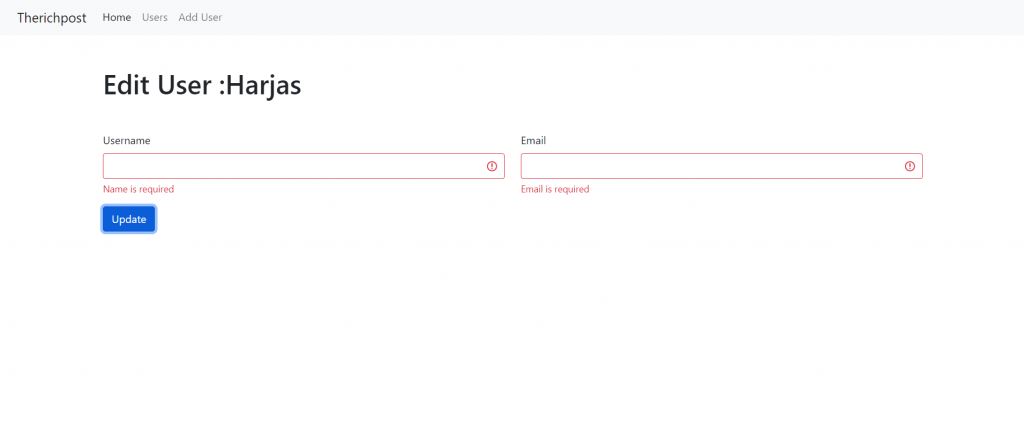
Guy’s with this post we will learn below things:
- Angular12 dynamic routing.
- Get url parameter value in angular12.
Guys here is working code snippet and please follow carefully to avoid the mistakes:
1. Guy’s very first we need to run the below command into our project terminal to create the edit user component:
ng g c edituser
2. Now friends, now we need to add below code into our angularcrud/src/app/app-routing.module.ts file to make edit user dynamic route:
... import { EdituserComponent } from './edituser/edituser.component'; const routes: Routes = [ ... { path: 'edituser/:id', component: EdituserComponent }, ];
3. Now friends, now we need to add below code into our angularcrud/src/app/crud.service.ts file to create EDIT User API services:
... export class CrudService { ... //update user public updateuser(userid) { return this.http.post('http://localhost/users.php/' , userid).subscribe((res: Response) => {}); } }
4. Now guys, now we need to add below code into our angularcrud/src/app/users/users.component.html file:
I must say please replace old file code with this code completely because I have done some more changes.
<div class="container p-5"> <h1>Users</h1> <table class="table table-hover table-bordered"> <thead> <tr> <th>ID</th> <th>Email</th> <th>Username</th> <th>Actions</th> </tr> </thead> <tbody> <tr *ngFor="let group of data"> <td>{{group.id}}</td> <td>{{group.email}}</td> <td>{{group.username}}</td> <td><button class="bg-danger"> <i class="fas fa-trash" (click)="deleteuser(group.id)"></i> </button> <a class="bg-warning" routerLink="/user/{{group.id}}"> <i class="fas fa-eye"></i> </a> <a class="bg-primary" routerLink="/edituser/{{group.id}}"> <i class="fas fa-edit"></i> </a></td> </tr> </tbody> </table> </div>
5. Now guys, now we need to add below code into our angularcrud/src/app/edituser/edituser.component.ts file:
... import {Router, ActivatedRoute, Params} from '@angular/router'; //ActivatedRoute module to get dynamicid from route import { FormBuilder, FormGroup, Validators } from '@angular/forms'; import { CrudService } from '../crud.service'; export class EdituserComponent implements OnInit { ... id:any; user:any; constructor(private activatedRoute: ActivatedRoute, private crudservice: CrudService, private formBuilder: FormBuilder, private router: Router) { //getting and storing dynamic ID this.id = this.activatedRoute.snapshot.paramMap.get('id'); //Single Product WEB API // Initialize Params Object var myFormData = new FormData(); // Begin assigning parameters myFormData.append('userid', this.id); //user details post request this.crudservice.getsingleuser(myFormData); setTimeout(()=>{ this.user = this.crudservice.singleuserdata; this.editForm.controls["firstname"].setValue(this.user.username); this.editForm.controls["email"].setValue(this.user.email); }, 100); } //Edit User editForm: FormGroup; submitted = false; //Add user form actions get f() { return this.editForm.controls; } onSubmit() { this.submitted = true; // stop here if form is invalid if (this.editForm.invalid) { return; } //True if all the fields are filled if(this.submitted) { // Initialize Params Object var myFormData = new FormData(); // Begin assigning parameters myFormData.append('updateUsername', this.editForm.value.firstname); myFormData.append('updateEmail', this.editForm.value.email); myFormData.append('updateid', this.user.id); this.crudservice.updateuser(myFormData); this.router.navigate([`/users`]); } } ngOnInit() { //Add User form validations this.editForm = this.formBuilder.group({ email: ['', [Validators.required, Validators.email]], firstname: ['', [Validators.required]] }); } }
6. Now guys, now we need to add below code into our angularcrud/src/app/edituser/edituser.component.html file:
<div class="container p-5"> <h1 class="text-left mb-5">Edit User :{{user.username}}</h1> <form [formGroup]="editForm" (ngSubmit)="onSubmit()"> <div class="row"> <div class="col-sm-6"> <div class="form-group"> <label>Username</label> <input type="text" formControlName="firstname" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.firstname.errors }" /> <div *ngIf="submitted && f.firstname.errors" class="invalid-feedback"> <div *ngIf="f.firstname.errors.required">Name is required</div> </div> </div> </div> <div class="col-sm-6"> <div class="form-group"> <label>Email</label> <input type="text" formControlName="email" class="form-control" [ngClass]="{ 'is-invalid': submitted && f.email.errors }" /> <div *ngIf="submitted && f.email.errors" class="invalid-feedback"> <div *ngIf="f.email.errors.required">Email is required</div> <div *ngIf="f.email.errors.email">Email must be a valid email address</div> </div> </div> </div> </div> <button type="submit" class="btn btn-primary">Update</button> </form> </div>
7. Now guys, now we need to add below code inside our xampp/htdocs/users.php file:
I must say please replace old file code with this code completely because I have done some more changes.
<?php header('Access-Control-Allow-Origin: *'); header('Access-Control-Allow-Credentials: true'); header('Access-Control-Allow-Methods:POST,GET,PUT,DELETE'); header('Access-Control-Allow-Headers: content-type or other'); header('Content-Type: application/json'); //Please create users database inside phpmysql admin and create userdetails tabel and create id, email and username fields $servername = "localhost"; $username = "root"; $password = ""; $dbname = "users"; // Create connection $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } //Add user if(isset($_POST['myEmail'])) { $sql = "INSERT INTO userdetails (email, username) VALUES ('".$_POST['myEmail']."', '".$_POST['myUsername']."')"; if (mysqli_query($conn,$sql)) { $data = array("data" => "You Data added successfully"); echo json_encode($data); } else { $data = array("data" => "Error: " . $sql . "<br>" . $conn->error); echo json_encode($data); } } //Delete user elseif(isset($_POST['deleteid'])) { $sql = mysqli_query($conn, "DELETE from userdetails where id =".$_POST['deleteid']); if ($sql) { $data = array("data" => "Record deleted successfully"); echo json_encode($data); } else { $data = array("data" =>"Error deleting record: " . mysqli_error($conn)); echo json_encode($data); } } //Get single user details elseif(isset($_POST['userid'])) { $trp = mysqli_query($conn, "SELECT * from userdetails where id =".$_POST['userid']); $rows = array(); while($r = mysqli_fetch_assoc($trp)) { $rows[] = $r; } print json_encode($rows); } //Update user elseif(isset($_POST["updateid"])) { $sql = "UPDATE userdetails SET email='".$_POST["updateEmail"]."', username='".$_POST["updateUsername"]."' WHERE id=".$_POST["updateid"]; if ($conn->query($sql) === TRUE) { $data = array("data" => "Record updated successfully"); echo json_encode($data); } else { $data = array("data" => "Error updating record: " . $conn->error); echo json_encode($data); } } else { //get all users details $trp = mysqli_query($conn, "SELECT * from userdetails ORDER BY id DESC"); $rows = array(); while($r = mysqli_fetch_assoc($trp)) { $rows[] = $r; } print json_encode($rows); } die();
Guy’s in the end please run ng serve command to check the out on browser(localhost:4200) and also please start your xampp. Guy’s If you have any kind of query then feel free to comment below.
Note: Friends, I just tell the basic setup and things, you can change the code according to your requirements. For better understanding must watch video above. I will appreciate that if you will tell your views for this post. Nothing matters if your views will be good or bad.
Jassa
Thanks.