Hello to all welcome back on my blog therichpost.com. Today in this blog post, I am going to tell you, Angular 12 Crud Tutorial with Services Part 2 – Delete User.
Important : Guy’s before stating with this, please check the first part for basic angular 12 setup with services:
Angular 12 Crud Tutorial with Services Part 1 – Add User
Guy’s with this post we will do below things:
- Sweetalert2 implementation in angular 12.
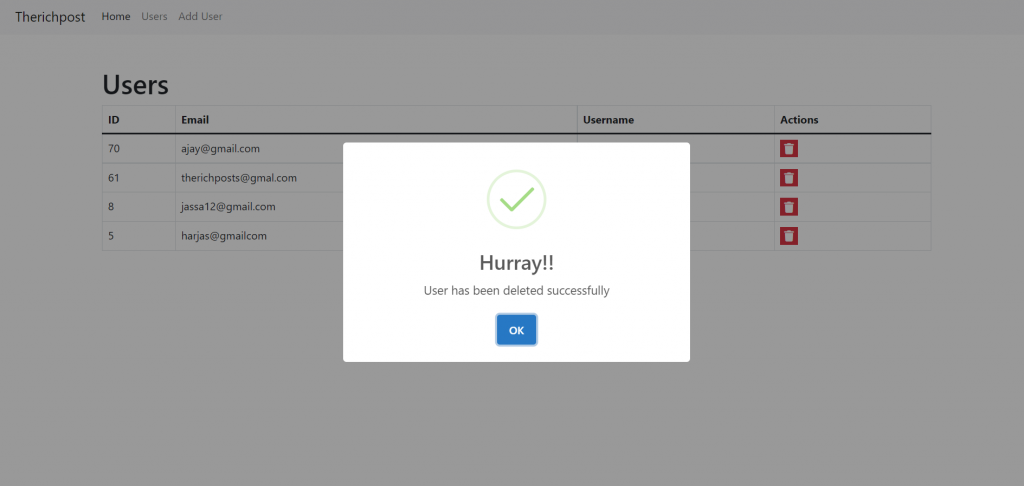
Guys here is working code snippet for Angular 12 Crud Tutorial with Services Part 2 – Delete User and please follow carefully to avoid the mistakes:
1. Now friends, here we need to run below command into our project terminal to install sweetalert1 module for popup alerts into our angular application:
npm install --save sweetalert2
2. Now friends, now we need to add below code into our angularcrud/angular.json file to add bootstrap style:
"styles": [ ... "node_modules/sweetalert2/src/sweetalert2.scss" ], "scripts": [ ... "node_modules/sweetalert2/dist/sweetalert2.js" ],
3. Now friends, now we need to add below code into our angularcrud/src/app/crud.service.ts file to create API services:
... export class CrudService { ... //delete user public deleteuser(userid) { return this.http.post('http://localhost/users.php/' , userid).subscribe((res: Response) => {}); } }
4. Now guys, now we need to add below code into our angularcrud/src/app/users/users.component.ts file to create delete user functionality:
I must say please replace old file code with this code completely because I have done some more changes.
... import { CrudService } from '../crud.service'; import Swal from 'sweetalert2/dist/sweetalert2.js'; export class UsersComponent implements OnInit { data = []; constructor( private crudservice: CrudService){ this.loaddata(); } //Get all users data loaddata() { //Get all usera details this.crudservice.getusers().subscribe((res: any[])=>{ this.data = res; }); } //Delete User deleteuser(id) { if(confirm("Are you sure to delete?")) { // Initialize Params Object var myFormData = new FormData(); // Begin assigning parameters myFormData.append('deleteid', id); this.crudservice.deleteuser(myFormData); //sweetalert message popup Swal.fire({ title: 'Hurray!!', text: "User has been deleted successfully", icon: 'success' }); this.loaddata(); } } ngOnInit(): void { } }
5. Now guys, now we need to add below code into our angularcrud/src/app/users/users.component.html file to create show users details table:
I must say please replace old file code with this code completely because I have done some more changes.
<div class="container p-5"> <h1>Users</h1> <table class="table table-hover table-bordered"> <thead> <tr> <th>ID</th> <th>Email</th> <th>Username</th> <th>Actions</th> </tr> </thead> <tbody> <tr *ngFor="let group of data"> <td>{{group.id}}</td> <td>{{group.email}}</td> <td>{{group.username}}</td> <td><button class="bg-danger"> <i class="fas fa-trash" (click)="deleteuser(group.id)"></i> </button></td> </tr> </tbody> </table> </div>
6. Now guy’s please add below code inside https://angularcrud/src/index.html file for icons:
... <head> ... <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.3.1/css/bootstrap.min.css"> <link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.6.3/css/all.css"> <style> .bg-primary, .bg-warning, .bg-danger, .bg-info{border:none; margin-right: 3px;} .fa-edit:before, .fa-user:before, .fa-trash:before, .fa-eye:before{color: #fff;} .btn{margin-right: 3px;} </style> </head> ...
7. Now guys, now we need to add below code inside our xampp/htdocs/users.php file:
I must say please replace old file code with this code completely because I have done some more changes.
<?php header('Access-Control-Allow-Origin: *'); header('Access-Control-Allow-Credentials: true'); header('Access-Control-Allow-Methods:POST,GET,PUT,DELETE'); header('Access-Control-Allow-Headers: content-type or other'); header('Content-Type: application/json'); //Please create users database inside phpmysql admin and create userdetails tabel and create id, email and username fields $servername = "localhost"; $username = "root"; $password = ""; $dbname = "users"; // Create connection $conn = new mysqli($servername, $username, $password, $dbname); // Check connection if ($conn->connect_error) { die("Connection failed: " . $conn->connect_error); } //Add user if(isset($_POST['myEmail'])) { $sql = "INSERT INTO userdetails (email, username) VALUES ('".$_POST['myEmail']."', '".$_POST['myUsername']."')"; if (mysqli_query($conn,$sql)) { $data = array("data" => "You Data added successfully"); echo json_encode($data); } else { $data = array("data" => "Error: " . $sql . "<br>" . $conn->error); echo json_encode($data); } } //Delete user elseif(isset($_POST['deleteid'])) { $sql = mysqli_query($conn, "DELETE from userdetails where id =".$_POST['deleteid']); if ($sql) { $data = array("data" => "Record deleted successfully"); echo json_encode($data); } else { $data = array("data" =>"Error deleting record: " . mysqli_error($conn)); echo json_encode($data); } } else { //get all users details $trp = mysqli_query($conn, "SELECT * from userdetails ORDER BY id DESC"); $rows = array(); while($r = mysqli_fetch_assoc($trp)) { $rows[] = $r; } print json_encode($rows); } die();
Now in the end please run ng serve command to check the out on browser(localhost:4200) and also please start your xampp.
Guy’s in next post we will do update user functionality. If you have any kind of query then please comment below.
Jassa
Thanks.